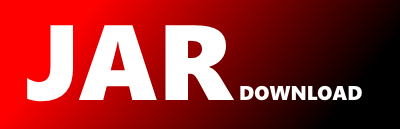
org.nuiton.plugin.VelocityTemplateGenerator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of helper-maven-plugin-api Show documentation
Show all versions of helper-maven-plugin-api Show documentation
Simple maven mojo api for our projects
package org.nuiton.plugin;
/*
* #%L
* Helper Maven Plugin :: API
* $Id: VelocityTemplateGenerator.java 876 2012-11-11 08:14:19Z tchemit $
* $HeadURL: http://svn.nuiton.org/svn/maven-helper-plugin/tags/maven-helper-plugin-2.1/helper-maven-plugin-api/src/main/java/org/nuiton/plugin/VelocityTemplateGenerator.java $
* %%
* Copyright (C) 2009 - 2012 Codelutin, Tony Chemit
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import org.apache.maven.project.MavenProject;
import org.apache.velocity.Template;
import org.apache.velocity.VelocityContext;
import org.apache.velocity.app.VelocityEngine;
import org.apache.velocity.runtime.resource.loader.JarResourceLoader;
import java.io.File;
import java.io.FileWriter;
import java.io.Writer;
import java.net.URISyntaxException;
import java.net.URL;
import java.util.Properties;
/**
* Generator of template base on velocity.
*
* TODO TC-20091011 should use plexus velocity component
*
* @author tchemit
* @since 1.3
*/
public class VelocityTemplateGenerator {
protected VelocityEngine engine;
protected MavenProject mavenProject;
protected Template velocityTemplate;
public VelocityTemplateGenerator(MavenProject mavenProject,
URL template) throws URISyntaxException {
if (mavenProject == null) {
throw new IllegalArgumentException("mavenProject must not be null");
}
if (template == null) {
throw new IllegalArgumentException("template must not be null");
}
this.mavenProject = mavenProject;
Properties props = new Properties();
String templateName;
if (template.toURI().isOpaque()) {
// template is in a jar
props = new Properties();
props.setProperty("resource.loader", "jar");
props.setProperty(
"jar.resource.loader.description",
"Jar resource loader for default webstart templates"
);
props.setProperty(
"jar.resource.loader.class",
JarResourceLoader.class.getName()
//"org.apache.velocity.runtime.resource.loader.JarResourceLoader"
);
// obtain the jar url
String url = template.toString();
int i = url.indexOf("!");
templateName = url.substring(i + 2);
props.setProperty("jar.resource.loader.path",
url.substring(0, i + 2));
} else {
File f = new File(template.getFile());
templateName = f.getName();
props.setProperty("file.resource.loader.path", f.getParent());
}
try {
engine = new VelocityEngine();
engine.init(props);
} catch (Exception e) {
IllegalArgumentException iae = new IllegalArgumentException(
"Could not initialise Velocity");
iae.initCause(e);
throw iae;
}
try {
velocityTemplate = engine.getTemplate(templateName);
} catch (Exception e) {
IllegalArgumentException iae =
new IllegalArgumentException(
"Could not load the template file from '" +
template + "'");
iae.initCause(e);
throw iae;
}
}
public void generate(Properties context, Writer writer) throws Exception {
VelocityContext vcontext = new VelocityContext();
// Note: properties that contain dots will not be properly parsed by
// Velocity. Should we replace dots with underscores ?
addPropertiesToContext(System.getProperties(), vcontext);
addPropertiesToContext(mavenProject.getProperties(), vcontext);
addPropertiesToContext(context, vcontext);
vcontext.put("project", mavenProject.getModel());
try {
velocityTemplate.merge(vcontext, writer);
writer.flush();
} catch (Exception e) {
throw new Exception(
"Could not generate the template " +
velocityTemplate.getName() + ": " + e.getMessage(), e);
} finally {
writer.close();
}
}
public void generate(Properties context, File outputFile) throws Exception {
VelocityContext vcontext = new VelocityContext();
// Note: properties that contain dots will not be properly parsed by
// Velocity. Should we replace dots with underscores ?
addPropertiesToContext(System.getProperties(), vcontext);
addPropertiesToContext(mavenProject.getProperties(), vcontext);
addPropertiesToContext(context, vcontext);
vcontext.put("project", mavenProject.getModel());
vcontext.put("outputFile", outputFile.getName());
FileWriter writer = new FileWriter(outputFile);
try {
velocityTemplate.merge(vcontext, writer);
writer.flush();
} catch (Exception e) {
throw new Exception(
"Could not generate the template " +
velocityTemplate.getName() + ": " + e.getMessage(), e);
} finally {
writer.close();
}
}
protected void addPropertiesToContext(Properties properties,
VelocityContext context) {
for (Object o : properties.keySet()) {
String key = (String) o;
Object value = properties.get(key);
context.put(key, value);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy