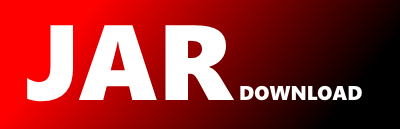
org.nuiton.plugin.TestHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of helper-maven-plugin-test-api Show documentation
Show all versions of helper-maven-plugin-test-api Show documentation
Simple maven mojo test api for our projects
package org.nuiton.plugin;
/*
* #%L
* Helper Maven Plugin :: Test Api
* %%
* Copyright (C) 2009 - 2015 CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.codehaus.plexus.PlexusTestCase;
import org.junit.Assert;
import java.io.File;
import java.io.IOException;
/**
* @author tchemit - [email protected]
* @since 1.0.3
*/
public abstract class TestHelper extends PluginHelper {
/** Logger. */
private static final Log log = LogFactory.getLog(TestHelper.class);
protected static boolean verbose;
protected static File basedir;
protected static File testBasedir;
public static File getTestBasedir() {
if (testBasedir == null) {
testBasedir = TestHelper.getFile(getBasedir(), "target", "test-classes");
if (log.isDebugEnabled()) {
log.debug("testBasedir = " + testBasedir);
}
}
return testBasedir;
}
/**
* @return the basedir for all tests
* @see PlexusTestCase#getBasedir()
*/
public static File getBasedir() {
if (basedir == null) {
String path = PlexusTestCase.getBasedir();
basedir = new File(path);
if (verbose) {
System.out.println("basedir = " + basedir.getAbsolutePath());
}
}
return basedir;
}
public static void setBasedir(File basedir) {
TestHelper.basedir = basedir;
}
public static boolean isVerbose() {
return verbose;
}
public static void setVerbose(boolean verbose) {
TestHelper.verbose = verbose;
}
public static File getTestDir(Class> type, String prefix) {
File f = new File(getBasedir(), prefix);
String rep = type.getName();
String[] paths = rep.split("\\.");
File testDir = PluginHelper.getFile(f, paths);
return testDir;
}
/**
* Checks on the given {@code file} that :
*
* - file exists
* - the given {@code pattern} exists {@code required = true}
* (or not {@code required = false}) in the content of the file.
*
*
* @param file the file to test
* @param pattern the pattern to search
* @param encoding encoding of the file to read
* @param required flag to says if pattern should (or not) be found in
* file's content
* @throws IOException if could not read file
*/
public static void checkExistsPattern(File file,
String pattern,
String encoding,
boolean required) throws IOException {
// checks file exists
Assert.assertTrue("File '" + file + "' does not exist, but should...",
file.exists()
);
//obtain file content as a string
String content = PluginHelper.readAsString(file, encoding);
checkPattern(file, content, pattern, required);
}
public static void checkPattern(File file,
String content,
String pattern,
boolean required) throws IOException {
String errorMessage = required ? "could not find the pattern : " :
"should not have found pattern :";
// checks pattern found (or not) in file's content
Assert.assertEquals(errorMessage + pattern + " in '" + file + "'",
required,
content.contains(pattern)
);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy