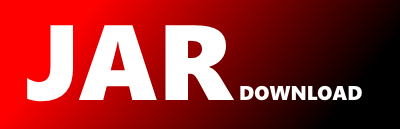
org.nuiton.helper.plugin.CheckAutoContainerPlugin Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-helper-plugin Show documentation
Show all versions of maven-helper-plugin Show documentation
Plugin d'aide pour les projets nuiton
/*
* #%L
* Maven helper plugin
*
* $Id: CheckAutoContainerPlugin.java 776 2010-10-23 11:44:57Z tchemit $
* $HeadURL: http://svn.nuiton.org/svn/maven-helper-plugin/tags/maven-helper-plugin-1.2.10/src/main/java/org/nuiton/helper/plugin/CheckAutoContainerPlugin.java $
* %%
* Copyright (C) 2009 - 2010 Tony Chemit, CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.helper.plugin;
import org.apache.maven.artifact.Artifact;
import org.apache.maven.artifact.ArtifactUtils;
import org.apache.maven.artifact.factory.ArtifactFactory;
import org.apache.maven.artifact.manager.WagonManager;
import org.apache.maven.artifact.repository.ArtifactRepository;
import org.apache.maven.artifact.repository.ArtifactRepositoryFactory;
import org.apache.maven.artifact.repository.layout.ArtifactRepositoryLayout;
import org.apache.maven.artifact.repository.layout.DefaultRepositoryLayout;
import org.apache.maven.artifact.resolver.ArtifactResolver;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.plugin.logging.Log;
import org.apache.maven.project.MavenProject;
import org.apache.maven.settings.Proxy;
import org.apache.maven.wagon.ConnectionException;
import org.apache.maven.wagon.Wagon;
import org.apache.maven.wagon.authentication.AuthenticationInfo;
import org.apache.maven.wagon.observers.Debug;
import org.apache.maven.wagon.proxy.ProxyInfo;
import org.apache.maven.wagon.repository.Repository;
import org.codehaus.plexus.util.StringUtils;
import org.nuiton.plugin.AbstractPlugin;
import org.nuiton.plugin.PluginHelper;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.TreeMap;
/**
* Check all dependencies are auto contained in the given repositories.
*
* @author tchemit
* @version $Id: CheckAutoContainerPlugin.java 776 2010-10-23 11:44:57Z tchemit $
* @goal check-auto-container
* @phase validate
* @requiresProject true
* @requiresOnline true
* @requiresDependencyResolution runtime
* @since 1.2.5
*/
public class CheckAutoContainerPlugin extends AbstractPlugin {
/**
* Map of repositories to use.
*
* Keys are repository id and Values are repository url.
*
* @parameter
* @since 1.2.5
*/
protected Map repositories;
/**
* A flag to add the maven central repository http://repo1.maven.org/maven2
* to {@link #repositories}.
*
* @parameter expression="${addMavenCentral}" default-value="false"
* @since 1.2.5
*/
protected boolean addMavenCentral;
/**
* A flag to fail if project is not auto container.
*
* @parameter expression="${helper.failIfNotSafe}" default-value="false"
* @since 1.2.5
*/
protected boolean failIfNotSafe;
/**
* A flag to activate verbose mode.
*
* @parameter expression="${helper.verbose}" default-value="${maven.verbose}"
* @since 1.2.5
*/
protected boolean verbose;
/**
* A flag to execute only once the mojo.
*
* Note: By default, value is {@code true} since it is not necessary
* to check twice for a same artifact.
*
* @parameter expression="${helper.runOnce}" default-value="true"
* @since 1.2.6
*/
protected boolean runOnce;
/**
* Project.
*
* @parameter default-value="${project}"
* @required
* @readonly
* @since 1.2.5
*/
protected MavenProject project;
/**
* The projects in reactor (used to detected sibling dependencies).
*
* @parameter expression="${reactorProjects}"
* @readonly
* @since 1.2.5
*/
protected List> reactorProjects;
/**
* Active proxy from settings (if any).
*
* @parameter default-value="${settings.activeProxy}"
* @required
* @readonly
* @since 1.2.5
*/
protected Proxy proxy;
/**
* Local repository.
*
* @parameter expression="${localRepository}"
* @required
* @readonly
* @since 1.2.5
*/
protected ArtifactRepository localRepository;
/**
* Artifact repository factory component.
*
* @component
* @required
* @readonly
* @since 1.2.5
*/
protected ArtifactRepositoryFactory artifactRepositoryFactory;
/**
* Artifact factory component.
*
* @component
* @required
* @readonly
* @since 1.2.5
*/
protected ArtifactFactory factory;
/**
* Artifact resolver component.
*
* @component
* @required
* @readonly
* @since 1.2.5
*/
protected ArtifactResolver resolver;
/**
* Wagon manager component.
*
* @component
* @required
* @readonly
* @since 1.2.5
*/
protected WagonManager wagonManager;
/**
* Authorized Remote Repositories.
*
* @since 1.2.5
*/
private List safeRepositories;
/**
* List of artifacts to treate.
*
* @since 1.2.5
*/
private List artifacts;
/**
* Dictionnary of already resolved artifacts (keys are repository url and
* values are list of artifact ids).
*
* @since 1.2.5
*/
private static Map> resolved;
public static final String MAVEN_CENTRAL_ID = "maven-central";
public static final String MAVEN_CENTRAL_URL = "http://repo1.maven.org/maven2/";
private boolean wasAlreadyExecuted;
@Override
public MavenProject getProject() {
return project;
}
@Override
public void setProject(MavenProject project) {
this.project = project;
}
@Override
public boolean isVerbose() {
return verbose;
}
@Override
public void setVerbose(boolean verbose) {
this.verbose = verbose;
}
@Override
public void init() throws Exception {
Log log = getLog();
if (log.isDebugEnabled()) {
// always be verbose in debug mode
setVerbose(true);
}
if (runOnce) {
boolean wasAlreadyExecuted = checkAlreadyExecuted();
if (wasAlreadyExecuted) {
return;
}
}
safeRepositories = createSafeRepositories();
artifacts = prepareArtifacts();
if (isVerbose()) {
log.info(artifacts.size() + " detected dependencies : ");
for (Object artifact : artifacts) {
log.info(" - " + artifact);
}
}
}
protected boolean checkAlreadyExecuted() {
// compute the unique key refering to parameters of plugin
StringBuilder buffer = new StringBuilder("check-auto-container##");
Artifact artifact = project.getArtifact();
buffer.append(artifact.getGroupId()).append(":");
buffer.append(artifact.getArtifactId()).append(":");
buffer.append(artifact.getVersion()).append("##");
// check if plugin was already done.
String key = buffer.toString();
if (verbose) {
getLog().info("check if already done for key : " + key);
}
Object value = project.getProperties().get(key);
if (value != null) {
// ok was already done
wasAlreadyExecuted = true;
}
long timestamp = System.nanoTime();
project.getProperties().put(key, timestamp + "");
if (verbose) {
getLog().info("Adding cache key " + key +
" with timestamp " + timestamp);
}
return wasAlreadyExecuted;
}
@Override
protected boolean checkSkip() {
if (runOnce && wasAlreadyExecuted) {
// ok was already done
getLog().info("Goal was already executed, will skip goal.");
return false;
}
if (artifacts.isEmpty()) {
getLog().info("Project has no dependecy.");
return false;
}
if (repositories.isEmpty()) {
getLog().info("No repository defined.");
return false;
}
return super.checkSkip();
}
@Override
protected void doAction() throws Exception {
Log log = getLog();
log.info(artifacts.size() + " dependencies to check.");
for (ArtifactRepository repository : safeRepositories) {
if (artifacts.isEmpty()) {
// no more artifacts to check
continue;
}
long t0 = System.nanoTime();
String url = repository.getUrl();
List ids = getRepositoryCache(url);
Wagon wagon = getWagon(repository);
try {
if (verbose) {
log.info("Use repository " + url);
}
List found = checkDependency(repository, wagon, ids);
long delay = System.nanoTime() - t0;
String message;
if (found.isEmpty()) {
message = "No artifact resolved by the repository " + url;
} else {
message = String.format("%1$4s artifact(s) resolved by repository %2$s in %3$s", found.size(), url, PluginHelper.convertTime(delay));
}
log.info(message);
artifacts.removeAll(found);
} finally {
disconnect(wagon);
}
}
boolean safe = artifacts.isEmpty();
if (safe) {
log.info("All dependencies are safe.");
return;
}
log.warn("There is " + artifacts.size() + " unsafe dependencie(s) :");
for (Object artifact : artifacts) {
log.warn(" - " + artifact);
}
if (failIfNotSafe) {
throw new MojoFailureException("There is still some unsafe dependencies.");
}
}
protected List createSafeRepositories() {
List safeRepositories = new ArrayList();
ArtifactRepositoryLayout repositoryLayout = new DefaultRepositoryLayout();
if (repositories == null) {
repositories = new TreeMap();
}
List ids = new ArrayList(repositories.keySet());
if (addMavenCentral) {
ids.add(0, MAVEN_CENTRAL_ID);
repositories.put(MAVEN_CENTRAL_ID, MAVEN_CENTRAL_URL);
}
for (String id : ids) {
String url = repositories.get(id);
url = url.trim();
ArtifactRepository repo;
repo = artifactRepositoryFactory.createDeploymentArtifactRepository(
String.valueOf(id),
url,
repositoryLayout, true);
getLog().info("Will use repository " + repo.getUrl());
if (verbose) {
getLog().info(repo.toString());
}
safeRepositories.add(repo);
}
return safeRepositories;
}
protected List prepareArtifacts() {
List result = new ArrayList();
List siblings = new ArrayList();
for (Object o : reactorProjects) {
MavenProject m = (MavenProject) o;
siblings.add(getArtifactId(m.getArtifact()));
}
// treate classic dependencies
for (Object o : project.getArtifacts()) {
Artifact a = (Artifact) o;
Artifact artifact = acceptArtifact(a, siblings);
if (artifact != null) {
result.add(a);
}
}
// treate also plugin dependencies
for (Object o : project.getPluginArtifacts()) {
Artifact a = (Artifact) o;
Artifact artifact = acceptArtifact(a, siblings);
if (artifact != null) {
result.add(a);
}
}
return result;
}
protected Artifact acceptArtifact(Artifact a, List siblings) {
String id = getArtifactId(a);
if (siblings.contains(id)) {
// skip a sibling dependency
if (verbose) {
getLog().info("Skip sibling dependency : " + id);
}
return null;
}
if (a.isSnapshot()) {
// skip snapshot
getLog().warn("Skip SNAPSHOT dependency : " + id);
return null;
}
Artifact artifact = ArtifactUtils.copyArtifact(a);
// force artifact not to be treated
artifact.setResolved(false);
// will treate this artifact
return artifact;
}
private List checkDependency(ArtifactRepository repo,
Wagon wagon,
List ids) throws Exception {
Log log = getLog();
List result = new ArrayList();
String url = repo.getUrl();
for (Artifact artifact : artifacts) {
if (log.isDebugEnabled()) {
log.debug(" - check artifact : " + artifact);
}
String id = getArtifactId(artifact);
boolean resolved;
boolean inCache = ids.contains(id);
if (inCache) {
// already resolved
resolved = true;
} else {
// first time resolving this artifact
Artifact copyArtifact = ArtifactUtils.copyArtifact(artifact);
String path = url + "/" + repo.pathOf(copyArtifact);
resolved = wagon.resourceExists(StringUtils.replace(path, repo.getUrl(), ""));
}
if (resolved) {
if (verbose) {
log.info(" - [ resolved ] " + artifact + (inCache ? " (from cache)" : ""));
}
result.add(artifact);
ids.add(id);
} else {
if (log.isDebugEnabled()) {
log.debug(" - [unresolved] " + artifact);
}
}
}
return result;
}
protected String getArtifactId(Artifact artifact) {
String id = artifact.getGroupId() + ":" +
artifact.getArtifactId() + ":" +
artifact.getVersion();
return id;
}
protected List getRepositoryCache(String url) {
if (resolved == null) {
resolved = new TreeMap>();
}
List ids = resolved.get(url);
if (ids == null) {
ids = new ArrayList();
resolved.put(url, ids);
}
return ids;
}
protected Wagon getWagon(ArtifactRepository repo) throws Exception {
Repository repository = new Repository(repo.getId(), repo.getUrl());
Wagon wagon = wagonManager.getWagon(repository);
wagon.setTimeout(1000);
if (getLog().isDebugEnabled()) {
Debug debug = new Debug();
wagon.addSessionListener(debug);
wagon.addTransferListener(debug);
}
// FIXME when upgrading to maven 3.x : this must be changed.
AuthenticationInfo auth = wagonManager.getAuthenticationInfo(repo.getId());
ProxyInfo proxyInfo = getProxyInfo();
if (proxyInfo != null) {
wagon.connect(repository, auth, proxyInfo);
} else {
wagon.connect(repository, auth);
}
return wagon;
}
protected void disconnect(Wagon wagon) {
try {
wagon.disconnect();
}
catch (ConnectionException e) {
Log log = getLog();
if (log.isDebugEnabled()) {
log.error("Error disconnecting wagon - ignored", e);
} else {
log.error("Error disconnecting wagon - ignored");
}
}
}
protected ProxyInfo getProxyInfo() {
ProxyInfo proxyInfo = null;
if (proxy != null && !StringUtils.isEmpty(proxy.getHost())) {
proxyInfo = new ProxyInfo();
proxyInfo.setHost(proxy.getHost());
proxyInfo.setType(proxy.getProtocol());
proxyInfo.setPort(proxy.getPort());
proxyInfo.setNonProxyHosts(proxy.getNonProxyHosts());
proxyInfo.setUserName(proxy.getUsername());
proxyInfo.setPassword(proxy.getPassword());
}
return proxyInfo;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy