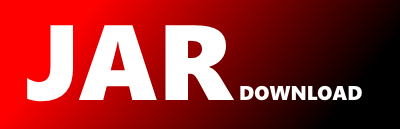
org.nuiton.io.mail.ProjectJavamailMailSender Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-helper-plugin Show documentation
Show all versions of maven-helper-plugin Show documentation
Plugin d'aide pour les projets nuiton
/*
* #%L
* Maven helper plugin
*
* $Id: ProjectJavamailMailSender.java 776 2010-10-23 11:44:57Z tchemit $
* $HeadURL: http://svn.nuiton.org/svn/maven-helper-plugin/tags/maven-helper-plugin-1.2.10/src/main/java/org/nuiton/io/mail/ProjectJavamailMailSender.java $
* %%
* Copyright (C) 2009 - 2010 Tony Chemit, CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.io.mail;
import java.security.Security;
import java.util.Date;
import java.util.Iterator;
import java.util.Properties;
import javax.mail.Authenticator;
import javax.mail.Message;
import javax.mail.MessagingException;
import javax.mail.PasswordAuthentication;
import javax.mail.Session;
import javax.mail.Transport;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeMessage;
import com.sun.net.ssl.internal.ssl.Provider;
import org.codehaus.plexus.mailsender.AbstractMailSender;
import org.codehaus.plexus.mailsender.MailMessage;
import org.codehaus.plexus.mailsender.MailSenderException;
import org.codehaus.plexus.mailsender.util.DateFormatUtils;
import org.codehaus.plexus.util.StringUtils;
/**
* Helper class for sending email.
*
* Note : this code was stolen in {@code maven-changes-plugin}, should thanks
* them...
*
* @author tchemit
* @since 1.0.3
*/
public class ProjectJavamailMailSender
extends AbstractMailSender {
private static final String SSL_FACTORY = "javax.net.ssl.SSLSocketFactory";
// ----------------------------------------------------------------------
//
// ----------------------------------------------------------------------
private Properties userProperties;
private Properties props;
// ----------------------------------------------------------------------
// Component Lifecycle
// ----------------------------------------------------------------------
public void initialize() {
if (StringUtils.isEmpty(getSmtpHost())) {
System.out.println("Error in configuration: Missing smtpHost.");
}
if (getSmtpPort() == 0) {
setSmtpPort(DEFAULT_SMTP_PORT);
}
props = new Properties();
props.put("mail.smtp.host", getSmtpHost());
props.put("mail.smtp.port", String.valueOf(getSmtpPort()));
if (getUsername() != null) {
props.put("mail.smtp.auth", "true");
}
props.put("mail.debug", String.valueOf(getLogger().isDebugEnabled()));
if (isSslMode()) {
Security.addProvider(new Provider());
props.put("mail.smtp.socketFactory.port", String.valueOf(getSmtpPort()));
props.put("mail.smtp.socketFactory.class", SSL_FACTORY);
props.put("mail.smtp.socketFactory.fallback", "false");
}
if (userProperties != null) {
for (Iterator> i = userProperties.keySet().iterator(); i.hasNext();) {
String key = (String) i.next();
String value = userProperties.getProperty(key);
props.put(key, value);
}
}
}
// ----------------------------------------------------------------------
// MailSender Implementation
// ----------------------------------------------------------------------
@Override
public void send(MailMessage mail)
throws MailSenderException {
verify(mail);
try {
Authenticator auth = null;
if (getUsername() != null) {
auth = new Authenticator() {
@Override
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication(getUsername(), getPassword());
}
};
}
Session session = Session.getDefaultInstance(props, auth);
session.setDebug(getLogger().isDebugEnabled());
Message msg = new MimeMessage(session);
InternetAddress addressFrom = new InternetAddress(mail.getFrom().getRfc2822Address());
msg.setFrom(addressFrom);
if (mail.getToAddresses().size() > 0) {
InternetAddress[] addressTo = new InternetAddress[mail.getToAddresses().size()];
int count = 0;
for (Iterator> i = mail.getToAddresses().iterator(); i.hasNext();) {
String address = ((MailMessage.Address) i.next()).getRfc2822Address();
addressTo[count++] = new InternetAddress(address);
}
msg.setRecipients(Message.RecipientType.TO, addressTo);
}
if (mail.getCcAddresses().size() > 0) {
InternetAddress[] addressCc = new InternetAddress[mail.getCcAddresses().size()];
int count = 0;
for (Iterator> i = mail.getCcAddresses().iterator(); i.hasNext();) {
String address = ((MailMessage.Address) i.next()).getRfc2822Address();
addressCc[count++] = new InternetAddress(address);
}
msg.setRecipients(Message.RecipientType.CC, addressCc);
}
if (mail.getBccAddresses().size() > 0) {
InternetAddress[] addressBcc = new InternetAddress[mail.getBccAddresses().size()];
int count = 0;
for (Iterator> i = mail.getBccAddresses().iterator(); i.hasNext();) {
String address = ((MailMessage.Address) i.next()).getRfc2822Address();
addressBcc[count++] = new InternetAddress(address);
}
msg.setRecipients(Message.RecipientType.BCC, addressBcc);
}
// Setting the Subject and Content Type
msg.setSubject(mail.getSubject());
msg.setContent(mail.getContent(), mail.getContentType() == null ? "text/plain" : mail.getContentType());
if (mail.getSendDate() != null) {
msg.setHeader("Date", DateFormatUtils.getDateHeader(mail.getSendDate()));
} else {
msg.setHeader("Date", DateFormatUtils.getDateHeader(new Date()));
}
// Send the message
Transport.send(msg);
} catch (MessagingException e) {
throw new MailSenderException("Error while sending mail.", e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy