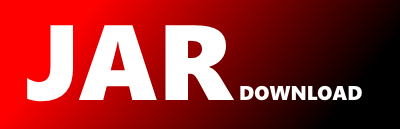
org.nuiton.io.rest.RestClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-helper-plugin Show documentation
Show all versions of maven-helper-plugin Show documentation
Plugin d'aide pour les projets nuiton
/*
* #%L
* Maven helper plugin
*
* $Id: RestClient.java 776 2010-10-23 11:44:57Z tchemit $
* $HeadURL: http://svn.nuiton.org/svn/maven-helper-plugin/tags/maven-helper-plugin-1.2.10/src/main/java/org/nuiton/io/rest/RestClient.java $
* %%
* Copyright (C) 2009 - 2010 Tony Chemit, CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.io.rest;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import java.io.IOException;
import java.io.InputStream;
import java.util.Map;
import java.util.TreeMap;
/**
* Abtract REST client.
*
* @author tchemit
* @since 1.0.3
*/
public abstract class RestClient {
private static final Log log = LogFactory.getLog(RestClient.class);
/** rest client configuration */
protected RestClientConfiguration configuration;
/** rest session */
protected RestSession session;
/** registred requests */
protected final Map requestBuilders;
/**
* Add the default available requests for this client.
*
* Note: This method is invoked in the constructor of the client.
*/
protected abstract void addDefaultRequests();
/**
* Open the client.
*
* Note: At the end of this method, if every thing is ok, then
* the method {@link #isOpen()} must returns {@code true}.
*
* @param session the rest session
* @throws IOException if any pb
*/
protected abstract void open(RestSession session) throws IOException;
/**
* Close the client.
*
* Note: At the end of this method, if every thing is ok, then
* the method {@link #isOpen()} must returns {@code false}.
*
* @param session the rest session
* @throws IOException if any pb
*/
protected abstract void close(RestSession session) throws IOException;
public RestClient() {
requestBuilders = new TreeMap();
addDefaultRequests();
}
public RestClient(RestClientConfiguration configuration) {
this();
this.configuration = configuration;
}
/**
* Add a request into the client.
*
* @param builder the new request to add
*/
public void addRequestBuilder(RestRequestBuilder builder) {
String name = builder.getName();
if (requestBuilders.containsKey(name)) {
throw new IllegalArgumentException("a request builder with name " + name + " already exists.");
}
requestBuilders.put(name, builder);
}
/**
* Obtain a request given his id and the args given.
*
* @param id id of the request
* @param args args passed to build the request
* @return the new request
*/
public RestRequest getRequest(String id, Object... args) {
RestRequestBuilder builder = requestBuilders.get(id);
if (builder == null) {
// pas de builder de requete...
return null;
}
RestRequest r = builder.create(args);
return r;
}
/**
* Ask some data from the server
*
* @param request request used for asking data
* @return the stream of the response
* @throws RestException if any pb while asking data
*/
public InputStream askData(RestRequest request) throws RestException {
if (!isOpen()) {
throw new IllegalStateException("the client is not opened");
}
try {
InputStream in = session.askData(request);
return in;
} catch (IOException e) {
throw new RestException(
"could not ask data to server to reason " +
e.getMessage(), e);
}
}
/**
* Send some datas to the server.
*
* @param request the request used for sending data
* @return the stream of the response
* @throws RestException if any pb while sending data
*/
public InputStream sendData(RestRequest request) throws RestException {
if (!isOpen()) {
throw new IllegalStateException("the client is not opened");
}
try {
InputStream in = session.sendData(request);
return in;
} catch (IOException e) {
throw new RestException(
"could not send data to server for reason " +
e.getMessage(), e);
}
}
/**
* Open the client.
*
* Note: this method will instanciate the {@link #session} and invoke
* the method {@link #open(RestSession)}.
*
* @throws RestException if any pb while opening session
*/
public void open() throws RestException {
if (isOpen()) {
return;
}
try {
session = new RestSession(configuration);
open(session);
session.setOpen(true);
} catch (Exception e) {
if (session != null) {
try {
try {
close(session);
} catch (IOException ex) {
// not important one...
}
} finally {
session = null;
}
}
throw new RestException(
"could not open rest client for reason " +
e.getMessage(), e);
}
}
/**
* Close the client.
*
* Note: this method will erase the {@link #session} and invoke
* the method {@link #close(RestSession)}.
*
* @throws RestException if any pb while closing session
*/
public void close() throws RestException {
if (!isOpen()) {
return;
}
try {
close(session);
} catch (Exception e) {
throw new RestException(
"could not close client for reason " +
e.getMessage(), e);
} finally {
session = null;
}
}
/**
* Is the client opened ?
*
* @return {@code true} if the internal {@link #session} is opened,
* {@code false} otherwise.
*/
public boolean isOpen() {
return session != null;
}
/** @return the internal rest {@link #session} */
public RestSession getSession() {
return session;
}
/** @return the internal {@link #configuration} */
public RestClientConfiguration getConfiguration() {
return configuration;
}
/**
* Use a new configuration.
*
* TODO : this method should check client is not opened!
*
* @param configuration the new configuration
*/
public void setConfiguration(RestClientConfiguration configuration) {
this.configuration = configuration;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy