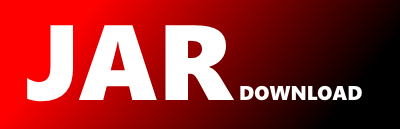
org.nuiton.helper.plugin.SendEmailMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-helper-plugin Show documentation
Show all versions of maven-helper-plugin Show documentation
Plugin d'aide pour les projets nuiton
The newest version!
/*
* #%L
* Maven helper plugin
*
* $Id: SendEmailMojo.java 814 2011-05-10 22:07:16Z tchemit $
* $HeadURL: http://svn.nuiton.org/svn/maven-helper-plugin/tags/maven-helper-plugin-1.3/src/main/java/org/nuiton/helper/plugin/SendEmailMojo.java $
* %%
* Copyright (C) 2009 - 2010 Tony Chemit, CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.helper.plugin;
import org.apache.commons.lang.StringUtils;
import org.apache.maven.model.Developer;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.project.MavenProject;
import org.codehaus.plexus.logging.Logger;
import org.codehaus.plexus.logging.console.ConsoleLogger;
import org.codehaus.plexus.mailsender.MailMessage;
import org.codehaus.plexus.mailsender.MailSenderException;
import org.nuiton.io.mail.MailSender;
import org.nuiton.io.mail.ProjectJavamailMailSender;
import org.nuiton.plugin.AbstractPlugin;
import org.nuiton.plugin.PluginHelper;
import org.nuiton.plugin.PluginWithEncoding;
import java.io.File;
import java.util.List;
/**
* Send a email.
*
* @author tchemit
* @goal send-email
* @requiresOnline true
* @requiresProject true
* @since 1.0.3
*/
public class SendEmailMojo extends AbstractPlugin implements PluginWithEncoding{
/**
* Dependance du projet.
*
* @parameter default-value="${project}"
* @required
* @readonly
* @since 1.0.3
*/
protected MavenProject project;
/**
* Un flag pour activer le mode verbeux.
*
* @parameter expression="${helper.verbose}" default-value="${maven.verbose}"
* @since 1.0.3
*/
protected boolean verbose;
/**
* The Velocity template used to format the email announcement.
*
* @parameter expression="${helper.emailContentFile}"
* @required
* @since 1.0.3
*/
protected File emailContentFile;
/**
* The title of the email to send.
*
* @parameter expression="${helper.emailTitle}"
* @required
* @since 1.0.3
*/
protected String emailTitle;
/**
* Smtp Server.
*
* @parameter expression="${helper.smtpHost}"
* @required
* @since 1.0.3
*/
protected String smtpHost;
/**
* Port.
*
* @parameter default-value="25" expression="${helper.smtpPort}"
* @required
* @since 1.0.3
*/
protected int smtpPort;
/**
* The username used to send the email.
*
* @parameter expression="${helper.username}"
* @since 1.0.3
*/
protected String username;
/**
* The password used to send the email.
*
* @parameter expression="${helper.password}"
* @since 1.0.3
*/
protected String password;
/**
* If the email should be sent in SSL mode.
*
* @parameter default-value="false" expression="${helper.sslMode}"
* @since 1.0.3
*/
protected boolean sslMode;
/**
* The id of the developer sending the announcement mail. Only used if
* the mailSender attribute is not set. In this case, this should
* match the id of one of the developers in the pom. If a matching
* developer is not found, then the first developer in the pom will be
* used.
*
* @parameter expression="${helper.fromDeveloperId}"
* @since 1.0.3
*/
protected String fromDeveloperId;
/**
* Defines the sender of the announcement if the list of developer is empty
* or if the sender is not a member of the development team.
*
* @parameter expression="${helper.mailSender}"
* @since 1.0.3
*/
protected MailSender mailSender;
/**
* Recipient email address.
*
* @parameter
* @required
* @since 1.0.3
*/
protected List toAddresses;
/**
* Possible senders.
*
* @parameter expression="${project.developers}"
* @required
* @readonly
* @since 1.0.3
*/
protected List from;
/**
* Mail content type to use.
*
* @parameter default-value="text/plain"
* @required
* @since 1.0.3
*/
protected String mailContentType;
/**
* The encoding used to read and write files.
*
* @parameter expression="${helper.encoding}" default-value="${project.build.sourceEncoding}"
* @since 1.0.3
*/
protected String encoding;
/**
* A flag to skip the goal.
*
* @parameter expression="${helper.skipSendEmail}" default-value="false"
* @since 1.0.3
*/
protected boolean skipSendEmail;
/**
* A flag to test plugin but send nothing to redmine.
*
* @parameter expression="${dryRun}" default-value="false"
* @since 1.0.3
*/
protected boolean dryRun;
/**
* A flag to restirct only one run in a build (for multi-module context).
*
* @parameter expression="${redmine.runOnce}" default-value="true"
* @since 1.0.3
*/
protected boolean runOnce;
/**
* internal flag to know if plugin was already executed
* (for multi-module projects)
*/
private boolean runOnceDone;
///////////////////////////////////////////////////////////////////////////
/// Plugin
///////////////////////////////////////////////////////////////////////////
@Override
public MavenProject getProject() {
return project;
}
@Override
public void setProject(MavenProject project) {
this.project = project;
}
@Override
public boolean isVerbose() {
return verbose;
}
@Override
public void setVerbose(boolean verbose) {
this.verbose = verbose;
}
///////////////////////////////////////////////////////////////////////////
/// AbstractPlugin
///////////////////////////////////////////////////////////////////////////
@Override
protected void init() throws Exception {
if (isGoalSkip()) {
return;
}
runOnceDone = false;
if (runOnce) {
// check
if (!isExecutionRoot()) {
runOnceDone = true;
return;
}
}
if (!emailContentFile.exists()) {
// no file to publish...
throw new MojoExecutionException(
"could not find the template " + emailContentFile);
}
// check mail sender
if (mailSender == null) {
if (from == null || from.isEmpty()) {
throw new MojoExecutionException(
"The section in your pom should " +
"not be empty. Add a entry or set " +
"the " + "mailSender parameter.");
}
if (fromDeveloperId == null) {
Developer dev = from.get(0);
mailSender = new MailSender(dev.getName(), dev.getEmail());
} else {
for (Developer developer : from) {
if (fromDeveloperId.equals(developer.getId())) {
mailSender = new MailSender(developer.getName(),
developer.getEmail()
);
break;
}
}
if (mailSender == null) {
throw new MojoExecutionException(
"Missing developer with id '" + fromDeveloperId +
"' in the section in your pom.");
}
}
}
String fromAddress = mailSender.getEmail();
if (StringUtils.isEmpty(fromAddress)) {
throw new MojoExecutionException(
"Invalid mail sender: name and email is mandatory (" +
mailSender + ").");
}
}
@Override
protected boolean checkSkip() {
if (isGoalSkip()) {
// goal mark to be skipped
getLog().info("skipGoal flag is on, will skip the goal.");
return false;
}
if (runOnce && runOnceDone) {
// already run
getLog().info("runOnce flag is on and goal was already " +
"executed, will skip the goal");
return false;
}
return true;
}
@Override
protected void doAction() throws Exception {
if (dryRun) {
getLog().info("\n dryRun flag is on, no mail will be send!\n");
}
String newsContent =
PluginHelper.readAsString(emailContentFile, encoding);
MailMessage mailMsg = createMessage(newsContent, mailSender);
ProjectJavamailMailSender mailer = createMailer();
if (getLog().isDebugEnabled()) {
getLog().debug("fromDeveloperId: " + fromDeveloperId);
}
if (dryRun) {
getLog().info("Mail title : " + emailTitle);
getLog().info("Mail content :\n" + mailMsg.getContent());
return;
}
getLog().info("Connecting to Host: " + smtpHost + ":" + smtpPort);
try {
mailer.send(mailMsg);
getLog().info("Sent...");
} catch (MailSenderException e) {
throw new MojoExecutionException(
"Failed to send email for reason " + e.getMessage(), e);
}
}
///////////////////////////////////////////////////////////////////////////
/// Other
///////////////////////////////////////////////////////////////////////////
protected boolean isGoalSkip() {
return skipSendEmail;
}
protected MailMessage createMessage(
String newsContent,
MailSender mailSender) throws MailSenderException {
String fromName = mailSender.getName();
String fromAddress = mailSender.getEmail();
getLog().info("Using this sender for email announcement: " +
fromAddress + " < " + fromName + " > ");
MailMessage mailMsg = new MailMessage();
mailMsg.setSubject(emailTitle);
if (getLog().isDebugEnabled()) {
getLog().debug("email announcement :\n" + newsContent);
}
mailMsg.setContent(newsContent);
mailMsg.setContentType(mailContentType);
mailMsg.setFrom(fromAddress, fromName);
for (String e : toAddresses) {
getLog().info("Sending mail to " + e + "...");
mailMsg.addTo(e, "");
}
return mailMsg;
}
protected ProjectJavamailMailSender createMailer() {
ProjectJavamailMailSender mailer = new ProjectJavamailMailSender();
ConsoleLogger logger = new ConsoleLogger(Logger.LEVEL_INFO, "base");
if (isVerbose()) {
logger.setThreshold(Logger.LEVEL_DEBUG);
}
mailer.enableLogging(logger);
mailer.setSmtpHost(smtpHost);
mailer.setSmtpPort(smtpPort);
mailer.setSslMode(sslMode);
if (username != null) {
mailer.setUsername(username);
}
if (password != null) {
mailer.setPassword(password);
}
mailer.initialize();
return mailer;
}
public void setMailSender(MailSender mailSender) {
this.mailSender = mailSender;
}
public void setFrom(List from) {
this.from = from;
}
public void setToAddresses(List toAddresses) {
this.toAddresses = toAddresses;
}
@Override
protected boolean isExecutionRoot() {
return project.isExecutionRoot();
}
@Override
public String getEncoding() {
return encoding;
}
@Override
public void setEncoding(String encoding) {
this.encoding = encoding;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy