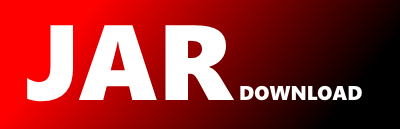
org.nuiton.helper.plugin.ShareServerSecretPlugin Maven / Gradle / Ivy
/*
* #%L
* Maven helper plugin
*
* $Id: ShareServerSecretPlugin.java 814 2011-05-10 22:07:16Z tchemit $
* $HeadURL: http://svn.nuiton.org/svn/maven-helper-plugin/tags/maven-helper-plugin-1.3/src/main/java/org/nuiton/helper/plugin/ShareServerSecretPlugin.java $
* %%
* Copyright (C) 2009 - 2010 Tony Chemit, CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.helper.plugin;
import org.apache.commons.lang.StringUtils;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.project.MavenProject;
import org.apache.maven.settings.Server;
import org.apache.maven.settings.Settings;
import org.nuiton.plugin.AbstractPlugin;
import org.sonatype.plexus.components.sec.dispatcher.SecDispatcher;
import java.util.EnumMap;
import java.util.Map;
import java.util.Properties;
/**
* Obtain a server authentication and share it in the maven
* project properties.
*
* To select data to export from the server with the given {@code serverId},
* fill the properties :
*
* usernameOut
* passwordOut
* privateKeyOut
* passphraseOut
*
*
* @author tchemit
* @goal share-server-secret
* @phase initialize
* @requiresProject true
* @since 1.1.0
*/
public class ShareServerSecretPlugin extends AbstractPlugin {
/**
* Project.
*
* @parameter default-value="${project}"
* @required
* @readonly
* @since 1.1.0
*/
protected MavenProject project;
/**
* Settings.
*
* @parameter default-value="${settings}"
* @required
* @readonly
* @since 1.1.0
*/
protected Settings settings;
/**
* Server id to use for authentication (must be defined in your setting
* and use the maven >= 2.1.0 password encryption mecanism).
*
*
* @parameter expression="${helper.serverId}"
* @required
* @since 1.1.0
*/
protected String serverId;
/**
* The name of the property where to export the username of the server.
*
* Note: If not set - then no export of the username of the server.
*
* @parameter
* @since 1.1.0
*/
protected String usernameOut;
/**
* The name of the property where to export the password of the server.
*
* Note: If not set - then no export of the password of the server.
*
* Note: If the password is crypted (since maven 2.1.0) then
* decrypt it.
*
* @parameter
* @since 1.1.0
*/
protected String passwordOut;
/**
* The name of the property where to export the passphrase of the server.
*
* Note: If not set - then no export of the passphrase of the server.
*
* Note: If the passphrase is crypted (since maven 2.1.0) then
* decrypt it.
*
* @parameter
* @since 1.1.0
*/
protected String passphraseOut;
/**
* The name of the property where to export the private key of the server.
*
* Note: If not set - then no export of the private key of the server.
*
* @parameter
* @since 1.1.0
*/
protected String privateKeyOut;
/**
* A flag to activate verbose mode.
*
* @parameter expression="${helper.verbose}" default-value="${maven.verbose}"
* @since 1.1.0
*/
protected boolean verbose;
/**
* A flag to execute only once the mojo.
*
* Note: By default, value is {@code true} since it is not necessary
* to inject twice secrets in session.
*
* @parameter expression="${helper.runOnce}" default-value="true"
* @since 1.1.0
*/
protected boolean runOnce;
/**
* password decypher
*
* @component roleHint="maven-helper-plugin"
* @since 1.1.0
*/
protected SecDispatcher sec;
/**
* the server to use to obtain secrets
*/
private Server server;
/**
* server secrets to expose
*/
private Map propertiesToTreate;
public enum Property {
username {
@Override
public String getSecret(Server server) {
return server.getUsername();
}
},
password {
@Override
public String getSecret(Server server) {
return server.getPassword();
}
},
passphrase {
@Override
public String getSecret(Server server) {
return server.getPassphrase();
}
},
privateKey {
@Override
public String getSecret(Server server) {
return server.getPrivateKey();
}
};
public abstract String getSecret(Server server);
}
@Override
public void init() throws Exception {
propertiesToTreate = new EnumMap(Property.class);
if (StringUtils.isNotEmpty(usernameOut)) {
propertiesToTreate.put(Property.username, usernameOut);
}
if (StringUtils.isNotEmpty(passwordOut)) {
propertiesToTreate.put(Property.password, passwordOut);
}
if (StringUtils.isNotEmpty(passphraseOut)) {
propertiesToTreate.put(Property.passphrase, passphraseOut);
}
if (StringUtils.isNotEmpty(privateKeyOut)) {
propertiesToTreate.put(Property.privateKey, privateKeyOut);
}
if (propertiesToTreate.isEmpty()) {
throw new MojoExecutionException(
"Nothing to export, you should specify what to " +
"export via 'usernameOut', 'passwordOut', " +
"'passphraseOut' or 'privateKeyOut' parameters.");
}
if (StringUtils.isEmpty(serverId)) {
throw new MojoExecutionException("No 'serverId' defined.");
}
server = settings.getServer(serverId.trim());
if (server == null) {
throw new MojoExecutionException(
"Could not find server with id '" + serverId +
"', check your settings.xml file.");
}
}
@Override
public boolean checkSkip() {
if (runOnce) {
// compute the unique key refering to parameters of plugin
StringBuilder buffer = new StringBuilder("share-secret##");
buffer.append(serverId);
buffer.append("##");
for (Map.Entry entry :
propertiesToTreate.entrySet()) {
buffer.append(entry.getKey()).append(entry.getValue());
}
// check if plugin was already done.
String key = buffer.toString();
if (verbose) {
getLog().info("check if already done for key : " + key);
}
Object value = project.getProperties().get(key);
if (value != null) {
// ok was already done
getLog().info("Goal was already executed, will skip goal.");
return false;
}
long timestamp = System.nanoTime();
project.getProperties().put(key, timestamp + "");
if (verbose) {
getLog().info("Adding cache key " + key +
" with timestamp " + timestamp);
}
}
return true;
}
@Override
protected void doAction() throws Exception {
Properties properties = project.getModel().getProperties();
for (Map.Entry entry :
propertiesToTreate.entrySet()) {
Property key = entry.getKey();
String propertyValue = key.getSecret(server);
String propertyTargetName = entry.getValue();
if (isVerbose()) {
getLog().info("will decrypt [" + key + "] : " + propertyValue);
}
propertyValue = sec.decrypt(propertyValue);
getLog().info("export server [" + serverId + "] " + key.name() +
" in ${" + propertyTargetName + "}");
properties.setProperty(propertyTargetName, propertyValue);
}
}
@Override
public MavenProject getProject() {
return project;
}
@Override
public void setProject(MavenProject project) {
this.project = project;
}
@Override
public boolean isVerbose() {
return verbose;
}
@Override
public void setVerbose(boolean verbose) {
this.verbose = verbose;
}
public String getPassphraseOut() {
return passphraseOut;
}
public void setPassphraseOut(String passphraseOut) {
this.passphraseOut = passphraseOut;
}
public String getPasswordOut() {
return passwordOut;
}
public void setPasswordOut(String passwordOut) {
this.passwordOut = passwordOut;
}
public String getPrivateKeyOut() {
return privateKeyOut;
}
public void setPrivateKeyOut(String privateKeyOut) {
this.privateKeyOut = privateKeyOut;
}
public String getUsernameOut() {
return usernameOut;
}
public void setUsernameOut(String usernameOut) {
this.usernameOut = usernameOut;
}
public String getServerId() {
return serverId;
}
public void setServerId(String serverId) {
this.serverId = serverId;
}
public void setSec(SecDispatcher sec) {
this.sec = sec;
}
public void setSettings(Settings settings) {
this.settings = settings;
}
public void setRunOnce(boolean runOnce) {
this.runOnce = runOnce;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy