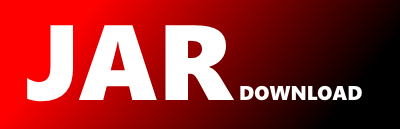
org.nuiton.io.xpp3.PropertyMapper Maven / Gradle / Ivy
/*
* #%L
* Maven helper plugin
*
* $Id: PropertyMapper.java 814 2011-05-10 22:07:16Z tchemit $
* $HeadURL: http://svn.nuiton.org/svn/maven-helper-plugin/tags/maven-helper-plugin-1.3/src/main/java/org/nuiton/io/xpp3/PropertyMapper.java $
* %%
* Copyright (C) 2009 - 2010 Tony Chemit, CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.io.xpp3;
import org.apache.commons.lang.builder.ToStringBuilder;
import org.codehaus.plexus.util.xml.pull.XmlPullParser;
import org.codehaus.plexus.util.xml.pull.XmlPullParserException;
import java.beans.PropertyDescriptor;
import java.io.IOException;
import java.text.ParseException;
import java.util.Map;
import java.util.Set;
/**
* A abstract object to map an xml value (tag or attribute, or esle?) to a pojo property.
*
* Two implementations are given :
*
*
* - {@link TagTextContentToProperty} to map the text content of a tag
* to a pojo's property
* - {@link AttributeValueToProperty} to map the text content of a tag
* to a pojo's property
*
*
* There is two convinient factory methods in {@link Xpp3Helper} to add some new mappers into a given
* dictionnary of mappers.
*
* - {@link Xpp3Helper#addTagTextContentMappers(Class, DataConverter, boolean, Map, String...)}
* - {@link Xpp3Helper#addAttributeValueMappers(Class, DataConverter, boolean, Map, String...)}
*
*
* @author tchemit
* @since 1.0.3
*/
public abstract class PropertyMapper {
public static class TagTextContentToProperty extends PropertyMapper {
public TagTextContentToProperty(String tagName,
String propertyName,
Class> containerType,
DataConverter type,
boolean onlyOne,
PropertyDescriptor descriptor) {
super(tagName,
propertyName,
containerType,
type,
onlyOne,
descriptor
);
}
@Override
protected Object getDataFromXml(XmlPullParser parser)
throws Exception {
String t = parser.nextText();
Object result = null;
if (t != null && !(t = t.trim()).isEmpty()) {
result = type.convert(t);
}
return result;
}
}
public static class AttributeValueToProperty extends PropertyMapper {
public AttributeValueToProperty(String tagName,
String propertyName,
Class> containerType,
DataConverter type,
boolean onlyOne,
PropertyDescriptor descriptor) {
super(tagName,
propertyName,
containerType,
type,
onlyOne,
descriptor
);
}
@Override
protected Object getDataFromXml(XmlPullParser parser)
throws Exception {
String t = parser.getAttributeValue("", name);
Object result = null;
if (t != null && !(t = t.trim()).isEmpty()) {
result = type.convert(t);
}
return result;
}
}
/** name of tag (or attribute to deal with) */
protected final String name;
/** the pojo's property to set */
protected final String propertyName;
/** the converter from xml to pojo's property type */
protected final DataConverter type;
/** the type of the pojo container of the property */
protected final Class> containerType;
/** the pojo's property descriptor */
protected final PropertyDescriptor descriptor;
/**
* a flag to check to use only once the mapper. (should not be used for
* attributes implementations).
*/
protected final boolean onlyOne;
protected PropertyMapper(String tagName,
String propertyName,
Class> containerType,
DataConverter type,
boolean onlyOne,
PropertyDescriptor descriptor) {
name = tagName;
this.propertyName = propertyName;
this.type = type;
this.onlyOne = onlyOne;
this.containerType = containerType;
this.descriptor = descriptor;
}
protected abstract Object getDataFromXml(XmlPullParser parser)
throws Exception;
public void setProperty(Object src,
XmlPullParser parser,
Set parsed, boolean strict)
throws XmlPullParserException, IOException {
if (onlyOne && parsed.contains(name)) {
throw new XmlPullParserException(
"Duplicated tag: \'" + parser.getName() + "\'",
parser,
null
);
}
parsed.add(name);
try {
Object r = getDataFromXml(parser);
if (r != null) {
descriptor.getWriteMethod().invoke(src, r);
}
} catch (XmlPullParserException e) {
throw e;
} catch (NumberFormatException e) {
if (strict) {
throw new XmlPullParserException(e.getMessage());
}
} catch (ParseException e) {
if (strict) {
throw new XmlPullParserException(e.getMessage());
}
} catch (Exception e) {
throw new XmlPullParserException(e.getMessage());
}
}
public PropertyDescriptor getDescriptor() {
return descriptor;
}
public boolean isOnlyOne() {
return onlyOne;
}
public String getPropertyName() {
return propertyName;
}
public String getTagName() {
return name;
}
public DataConverter getType() {
return type;
}
public Class> getContainerType() {
return containerType;
}
public String getName() {
return name;
}
@Override
public String toString() {
ToStringBuilder toStringBuilder = new ToStringBuilder(this);
toStringBuilder.append("name", name);
toStringBuilder.append("propertyName", propertyName);
toStringBuilder.append("onlyOne", onlyOne);
toStringBuilder.append("type", type);
toStringBuilder.append("containerType", containerType);
return toStringBuilder.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy