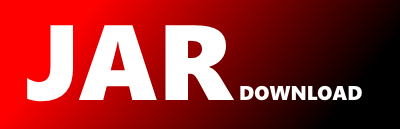
org.nuiton.plugin.PluginHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-helper-plugin Show documentation
Show all versions of maven-helper-plugin Show documentation
Plugin d'aide pour les projets nuiton
The newest version!
/*
* #%L
* Maven helper plugin
*
* $Id: PluginHelper.java 814 2011-05-10 22:07:16Z tchemit $
* $HeadURL: http://svn.nuiton.org/svn/maven-helper-plugin/tags/maven-helper-plugin-1.3/src/main/java/org/nuiton/plugin/PluginHelper.java $
* %%
* Copyright (C) 2009 - 2010 Tony Chemit, CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.plugin;
import org.apache.maven.model.Resource;
import org.apache.maven.project.MavenProject;
import org.codehaus.plexus.util.DirectoryScanner;
import org.codehaus.plexus.util.FileUtils;
import org.codehaus.plexus.util.IOUtil;
import org.codehaus.plexus.util.ReaderFactory;
import org.codehaus.plexus.util.StringUtils;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStreamWriter;
import java.io.Reader;
import java.io.StringReader;
import java.net.MalformedURLException;
import java.net.URL;
import java.text.MessageFormat;
import java.util.ArrayList;
import java.util.Enumeration;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.SortedSet;
import java.util.TreeSet;
import java.util.zip.ZipEntry;
import java.util.zip.ZipFile;
/**
* Une classe pour mutualiser toutes les méthodes utiles pour un plugin.
*
* @author tchemit
*/
public class PluginHelper {
public static final String SNAPSHOT_SUFFIX = "-SNAPSHOT";
public static String removeSnapshotSuffix(String versionId) {
if (versionId.endsWith(SNAPSHOT_SUFFIX)) {
// remove snapshot suffix
versionId = versionId.substring(
0,
versionId.length() - SNAPSHOT_SUFFIX.length()
);
}
return versionId;
}
/**
* Permet de convertir une liste non typee, en une liste typee.
*
* La liste en entree en juste bien castee.
*
* On effectue une verification sur le typage des elements de la liste.
*
* Note : Aucune liste n'est creee, ni recopiee
*
* @param le type des objets de la liste
* @param list la liste a convertir
* @param type le type des elements de la liste
* @return la liste typee
* @throws IllegalArgumentException si un element de la liste en entree
* n'est pas en adequation avec le type
* voulue.
*/
@SuppressWarnings({"unchecked"})
static public List toGenericList(
List> list, Class type) throws IllegalArgumentException {
if (list.isEmpty()) {
return (List) list;
}
for (Object o : list) {
if (!type.isAssignableFrom(o.getClass())) {
throw new IllegalArgumentException(
"can not cast List with object of type " +
o.getClass() + " to " + type + " type!");
}
}
return (List) list;
}
static final protected double[] timeFactors = {1000000, 1000, 60, 60, 24};
static final protected String[] timeUnites = {"ns", "ms", "s", "m", "h",
"d"};
static final protected double[] memoryFactors = {1024, 1024, 1024, 1024};
static final protected String[] memoryUnites = {"o", "Ko", "Mo", "Go",
"To"};
static public String convertMemory(long value) {
return convert(value, memoryFactors, memoryUnites);
}
static public String convertTime(long value) {
return convert(value, timeFactors, timeUnites);
}
static public String convertTime(long value, long value2) {
return convertTime(value2 - value);
}
static public String convert(long value,
double[] factors,
String[] unites) {
long sign = value == 0 ? 1 : value / Math.abs(value);
int i = 0;
double tmp = Math.abs(value);
while (i < factors.length && i < unites.length && tmp > factors[i]) {
tmp = tmp / factors[i++];
}
tmp *= sign;
String result;
result = MessageFormat.format("{0,number,0.###}{1}", tmp, unites[i]);
return result;
}
/**
* Split the given {@code text} using the {@code separator} and trim each
* part of result.
*
* @param txt text to split
* @param separator the split separator
* @return the splited text with trimmed parts.
* @since 1.1.0
*/
public static String[] splitAndTrim(String txt, String separator) {
String[] result = txt.split(separator);
for (int i = 0, j = result.length; i < j; i++) {
result[i] = result[i].trim();
}
return result;
}
/**
* Prefix the lines of the given content with a given prefix.
*
* @param prefix prefix to add on each line of text
* @param prefixForEmpty prefix to add for empty lines
* @param content the text to treate
* @return the text transformed
* @throws IOException if any reading problem
*/
static public String prefixLines(String prefix,
String prefixForEmpty,
String content) throws IOException {
BufferedReader reader = null;
reader = new BufferedReader(new StringReader(content));
try {
StringBuilder sb = new StringBuilder();
String line = reader.readLine();
while (line != null) {
line = line.trim();
if (line.isEmpty()) {
sb.append(prefixForEmpty);
} else {
sb.append(prefix);
sb.append(line);
}
line = reader.readLine();
if (line != null) {
sb.append('\n');
}
}
String result = sb.toString();
return result;
} finally {
reader.close();
}
}
/**
* Obtenir les clefs de toutes les valeurs nulles ou vide a partir d'un
* dictionnaire donne.
*
* @param map le dictionner a parcourir
* @return la liste des clefs dont la valeur est nulle ou vide
*/
static public SortedSet getEmptyKeys(Map, ?> map) {
SortedSet result = new TreeSet();
for (Entry, ?> e : map.entrySet()) {
if (e.getValue() == null || e.getValue().toString().isEmpty()) {
result.add(e.getKey().toString());
}
}
return result;
}
/**
* Add the directory as a resource of the given project.
*
* @param dir the directory to add
* @param project the project to update
* @param includes the includes of the resource
* @return {@code true} if the resources was added (not already existing)
* @since 1.1.1
*/
public static boolean addResourceDir(File dir,
MavenProject project,
String... includes) {
List> resources = project.getResources();
boolean added = addResourceDir(dir, project, resources, includes);
return added;
}
/**
* Add thedirectory as a test resource of the given project.
*
* @param dir the directory to add
* @param project the project to update
* @param includes the includes of the resource
* @return {@code true} if the resources was added (not already existing)
* @since 1.1.1
*/
public static boolean addTestResourceDir(File dir,
MavenProject project,
String... includes) {
List> resources = project.getTestResources();
boolean added = addResourceDir(dir, project, resources, includes);
return added;
}
/**
* Add the directory as a resource in the given resource list.
*
* @param dir the directory to add
* @param project the project involved
* @param resources the list of existing resources
* @param includes includes of the new resources
* @return {@code true} if the resource was added (not already existing)
* @since 1.1.1
*/
public static boolean addResourceDir(File dir,
MavenProject project,
List> resources,
String... includes) {
String newresourceDir = dir.getAbsolutePath();
boolean shouldAdd = true;
for (Object o : resources) {
Resource r = (Resource) o;
if (!r.getDirectory().equals(newresourceDir)) {
continue;
}
for (String i : includes) {
if (!r.getIncludes().contains(i)) {
r.addInclude(i);
}
}
shouldAdd = false;
break;
}
if (shouldAdd) {
Resource r = new Resource();
r.setDirectory(newresourceDir);
for (String i : includes) {
if (!r.getIncludes().contains(i)) {
r.addInclude(i);
}
}
project.addResource(r);
}
return shouldAdd;
}
/**
* Cretae the directory (and his parents) if necessary.
*
* @param dir the directory to create if not exisiting
* @return {@code true} if directory was created, {@code false} if was no
* need to create it
* @throws IOException if could not create directory
* @since 1.1.1
*/
public static boolean createDirectoryIfNecessary(
File dir) throws IOException {
if (!dir.exists()) {
boolean b = dir.mkdirs();
if (!b) {
throw new IOException("Could not create directory " + dir);
}
return true;
}
return false;
}
/**
* Create the given file.
*
* @param file the file to create
* @throws IOException if could not create the file
* @since 1.1.1
*/
public static void createNewFile(File file) throws IOException {
createDirectoryIfNecessary(file.getParentFile());
boolean b = file.createNewFile();
if (!b) {
throw new IOException("could not create new file " + file);
}
}
/**
* Delete the given file.
*
* @param file the file to delete
* @throws IOException if could not delete the file
* @since 1.1.1
*/
public static void deleteFile(File file) throws IOException {
if (!file.exists()) {
// file does not exist, can not delete it
return;
}
boolean b = file.delete();
if (!b) {
throw new IOException("could not delete file " + file);
}
}
/**
* Rename the given file to a new destination.
*
* @param file the file to rename
* @param destination the destination file
* @throws IOException if could not rename the file
* @since 1.2.0
*/
public static void renameFile(File file,
File destination) throws IOException {
boolean b = file.renameTo(destination);
if (!b) {
throw new IOException(
"could not rename " + file + " to " + destination);
}
}
public static void setLastModified(File file,
long lastModified) throws IOException {
boolean b = file.setLastModified(lastModified);
if (!b) {
throw new IOException(
"could not changed lastModified [" + lastModified +
"] for " + file);
}
}
/**
* Permet de copier le fichier source vers le fichier cible.
*
* @param source le fichier source
* @param target le fichier cible
* @throws IOException Erreur de copie
*/
public static void copy(File source, File target) throws IOException {
createDirectoryIfNecessary(target.getParentFile());
FileUtils.copyFile(source, target);
}
/**
* Permet de lire un fichier et de retourner sont contenu sous forme d'une
* chaine de carateres
*
* @param file le fichier a lire
* @param encoding encoding to read file
* @return the content of the file
* @throws IOException if IO pb
*/
static public String readAsString(File file,
String encoding) throws IOException {
FileInputStream inf = new FileInputStream(file);
BufferedReader in = new BufferedReader(
new InputStreamReader(inf, encoding));
try {
return IOUtil.toString(in);
} finally {
in.close();
}
}
/**
* Permet de lire un fichier et de retourner sont contenu sous forme d'une
* chaine de carateres
*
* @param reader la source alire
* @return the content of the file
* @throws IOException if IO pb
*/
static public String readAsString(Reader reader) throws IOException {
return IOUtil.toString(reader);
}
/**
* Sauvegarde un contenu dans un fichier.
*
* @param file le fichier a ecrire
* @param content le contenu du fichier
* @param encoding l'encoding d'ecriture
* @throws IOException if IO pb
*/
static public void writeString(File file, String content,
String encoding) throws IOException {
createDirectoryIfNecessary(file.getParentFile());
BufferedWriter out = null;
out = new BufferedWriter(new OutputStreamWriter(
new FileOutputStream(file), encoding));
try {
IOUtil.copy(content, out);
} finally {
out.close();
}
}
public static List getIncludedFiles(File dir,
String[] includes,
String[] excludes) {
DirectoryScanner ds = new DirectoryScanner();
List result = new ArrayList();
ds.setBasedir(dir);
if (includes != null) {
ds.setIncludes(includes);
}
if (excludes != null) {
ds.setExcludes(excludes);
}
ds.addDefaultExcludes();
ds.scan();
for (String file : ds.getIncludedFiles()) {
File in = new File(dir, file);
result.add(in);
}
return result;
}
public static void copyFiles(File src, File dst,
String[] includes,
String[] excludes,
boolean overwrite) throws IOException {
PluginIOContext c = new PluginIOContext();
c.setInput(src);
c.setOutput(dst);
copyFiles(c, includes, excludes, overwrite);
}
public static void copyFiles(PluginIOContext p,
String[] includes,
String[] excludes,
boolean overwrite) throws IOException {
DirectoryScanner ds = new DirectoryScanner();
for (File input : p.getInputs()) {
ds.setBasedir(input);
if (includes != null) {
ds.setIncludes(includes);
}
if (excludes != null) {
ds.setExcludes(excludes);
}
ds.addDefaultExcludes();
ds.scan();
for (String file : ds.getIncludedFiles()) {
File in = new File(input, file);
File out = new File(p.getOutput(), file);
if (overwrite) {
FileUtils.copyFile(in, out);
} else {
FileUtils.copyFileIfModified(in, out);
}
}
}
}
public static void expandFiles(PluginIOContext p,
String[] includes,
String[] excludes,
String[] zipIncludes,
boolean overwrite) throws IOException {
DirectoryScanner ds = new DirectoryScanner();
for (File input : p.getInputs()) {
ds.setBasedir(input);
if (includes != null) {
ds.setIncludes(includes);
}
if (excludes != null) {
ds.setExcludes(excludes);
}
ds.addDefaultExcludes();
ds.scan();
for (String file : ds.getIncludedFiles()) {
File in = new File(input, file);
File out = new File(p.getOutput(), file).getParentFile();
expandFile(in, out, zipIncludes, overwrite);
}
}
}
public static File getFile(File base, String... paths) {
StringBuilder buffer = new StringBuilder();
for (String path : paths) {
buffer.append(File.separator).append(path);
}
File f = new File(base, buffer.substring(1));
return f;
}
public static String getRelativePath(File base, File file) {
String result = file.getAbsolutePath().substring(
base.getAbsolutePath().length() + 1);
return result;
}
public static void expandFile(File src,
File dst,
String[] includes,
boolean overwrite) throws IOException {
ZipFile zipFile = new ZipFile(src);
Enumeration extends ZipEntry> entries = zipFile.entries();
while (entries.hasMoreElements()) {
ZipEntry nextElement = entries.nextElement();
String name = nextElement.getName();
for (String include : includes) {
if (DirectoryScanner.match(include, name)) {
System.out.println("matching name : " + name +
" with pattern " + include);
File dstFile = new File(dst, name);
if (overwrite ||
!dstFile.exists() ||
nextElement.getTime() > dstFile.lastModified()) {
System.out.println("will expand : " + name + " to " +
dstFile);
InputStream inputStream =
zipFile.getInputStream(nextElement);
FileOutputStream outStream =
new FileOutputStream(dstFile);
try {
IOUtil.copy(inputStream, outStream, 2048);
} finally {
outStream.close();
}
}
}
}
}
}
/**
* @param src the source file to read
* @return the lines of the source file.
* @throws IOException if any pb while reading file
*/
public static String[] getLines(File src) throws IOException {
List result = new ArrayList();
BufferedReader stream = new BufferedReader(
new InputStreamReader(new FileInputStream(src)));
try {
while (stream.ready()) {
String line = stream.readLine().trim();
if (!line.isEmpty()) {
result.add(line);
}
}
} finally {
stream.close();
}
return result.toArray(new String[result.size()]);
}
/**
* @param src the source file to read
* @return the files instanciate from lines of the source file.
* @throws IOException if any pb while reading file
*/
public static File[] getLinesAsFiles(File src) throws IOException {
List result = new ArrayList();
for (String line : getLines(src)) {
if (!line.isEmpty()) {
result.add(new File(line));
}
}
return result.toArray(new File[result.size()]);
}
/**
* @param src the source file to read
* @return the url instanciated from lines of the source file.
* @throws IOException if any pb while reading file
*/
public static URL[] getLinesAsURL(File src) throws IOException {
List result = new ArrayList();
for (String line : getLines(src)) {
if (!line.isEmpty()) {
result.add(new URL(line));
}
}
return result.toArray(new URL[result.size()]);
}
/**
* suffix a given {@code baseUrl} with the given {@code suffix}
*
* @param baseUrl base url to use
* @param suffix suffix to add
* @return the new url
* @throws IllegalArgumentException if malformed url.
* @since 1.2.3
*/
public static URL getUrl(URL baseUrl,
String suffix) throws IllegalArgumentException {
String url = baseUrl.toString() + "/" + suffix;
try {
return new URL(url);
} catch (MalformedURLException ex) {
throw new IllegalArgumentException(
"could not obtain url " + url, ex);
}
}
/**
* Method to be invoked in init phase to check sanity of {@link PluginWithEncoding#getEncoding()}.
*
* If no encoding was filled, then use the default for system
* (via {@code file.encoding} environement property).
*
* Note: If mojo is not implementing {@link PluginWithEncoding},
* nothing is done.
*
* @param mojo the mojo to check.
* @since 1.2.6
*/
public static void checkEncoding(PluginWithEncoding mojo) {
if (StringUtils.isEmpty(mojo.getEncoding())) {
mojo.getLog().warn(
"File encoding has not been set, using platform encoding " + ReaderFactory.FILE_ENCODING
+ ", i.e. build is platform dependent!");
mojo.setEncoding(ReaderFactory.FILE_ENCODING);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy