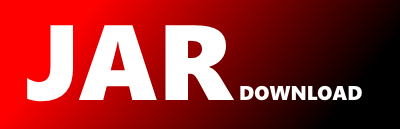
org.nuiton.license.plugin.AddThirdPartyMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-license-plugin Show documentation
Show all versions of maven-license-plugin Show documentation
Plugin pour switcher de license dans un module maven 2
The newest version!
/*
* #%L
* Maven License Plugin
*
* $Id: AddThirdPartyMojo.java 1879 2010-11-18 20:25:57Z tchemit $
* $HeadURL: http://svn.nuiton.org/svn/maven-license-plugin/tags/maven-license-plugin-3.0/src/main/java/org/nuiton/license/plugin/AddThirdPartyMojo.java $
* %%
* Copyright (C) 2008 - 2010 CodeLutin, Tony Chemit
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.license.plugin;
import org.apache.commons.collections.CollectionUtils;
import org.apache.commons.lang.StringUtils;
import org.apache.maven.artifact.Artifact;
import org.apache.maven.artifact.repository.ArtifactRepository;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.plugin.logging.Log;
import org.apache.maven.project.MavenProject;
import org.apache.maven.project.MavenProjectBuilder;
import org.apache.maven.project.ProjectBuildingException;
import org.nuiton.io.SortedProperties;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.SortedSet;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import java.util.regex.PatternSyntaxException;
/**
* The gola to generate the third-party file.
*
* This file contains for all dependencies of the project a line giving the
* license used by the dependency.
*
* It will also copy it in the class-path (says add the generated directory as
* a resource of the build).
*
* @author tchemit
* @goal add-third-party
* @phase generate-resources
* @requiresDependencyResolution test
* @requiresProject true
* @since 2.2 (was previously {@code AddThirdPartyFileMojo}).
*/
public class AddThirdPartyMojo extends AbstractAddThirdPartyMojo {
/**
* Local Repository.
*
* @parameter expression="${localRepository}"
* @required
* @readonly
* @since 1.0.0
*/
protected ArtifactRepository localRepository;
/**
* Remote repositories used for the project.
*
* @parameter expression="${project.remoteArtifactRepositories}"
* @required
* @readonly
* @since 1.0.0
*/
protected List> remoteRepositories;
/**
* Maven Project Builder component.
*
* @component
* @required
* @readonly
* @since 1.0.0
*/
protected MavenProjectBuilder mavenProjectBuilder;
private boolean doGenerateMissing;
@Override
protected boolean checkPackaging() {
return rejectPackaging(Packaging.pom);
}
@Override
protected LicenseMap createLicenseMap() throws ProjectBuildingException {
Log log = getLog();
LicenseMap licenseMap = new LicenseMap();
licenseMap.setLog(log);
boolean haveNoIncludedGroups = StringUtils.isEmpty(includedGroups);
boolean haveNoIncludedArtifacts = StringUtils.isEmpty(includedArtifacts);
boolean haveExcludedGroups = StringUtils.isNotEmpty(excludedGroups);
boolean haveExcludedArtifacts = StringUtils.isNotEmpty(excludedArtifacts);
boolean haveExclusions = haveExcludedGroups || haveExcludedArtifacts;
Pattern includedGroupPattern = null;
Pattern includedArtifactPattern = null;
Pattern excludedGroupPattern = null;
Pattern excludedArtifactPattern = null;
if (!haveNoIncludedGroups) {
includedGroupPattern = Pattern.compile(includedGroups);
}
if (!haveNoIncludedArtifacts) {
includedArtifactPattern = Pattern.compile(includedArtifacts);
}
if (haveExcludedGroups) {
excludedGroupPattern = Pattern.compile(excludedGroups);
}
if (haveExcludedArtifacts) {
excludedArtifactPattern = Pattern.compile(excludedArtifacts);
}
// build the license map for the dependencies of the project
for (Object o : getProject().getArtifacts()) {
Artifact artifact = (Artifact) o;
if (Artifact.SCOPE_SYSTEM.equals(artifact.getScope())) {
// never treate system artifacts (they are mysterious and
// no information can be retrive from anywhere)...
continue;
}
String id = ArtifactHelper.getArtifactId(artifact);
if (isVerbose()) {
getLog().info("detected artifact " + id);
}
// Check if the project should be included
// If there is no specified artifacts and group to include, include all
boolean isToInclude = haveNoIncludedArtifacts && haveNoIncludedGroups
|| isIncludable(artifact, includedGroupPattern, includedArtifactPattern);
// Check if the project should be excluded
boolean isToExclude = isToInclude
&& haveExclusions
&& isExcludable(artifact, excludedGroupPattern, excludedArtifactPattern);
if (isToInclude && !isToExclude) {
MavenProject project = addArtifact(id, artifact);
licenseMap.addLicense(project, project.getLicenses());
}
}
return licenseMap;
}
@Override
protected SortedProperties createUnsafeMapping() throws ProjectBuildingException, IOException {
SortedProperties unsafeMappings =
getLicenseMap().loadUnsafeMapping(getEncoding(),
getMissingFile());
SortedSet unsafeDependencies = getUnsafeDependencies();
if (isVerbose()) {
getLog().info("found " + unsafeMappings.size() + " unsafe mappings");
}
// compute if missing file should be (re)-generate
boolean generateMissingfile = computeDoGenerateMissingFile(
unsafeMappings,
unsafeDependencies
);
setDoGenerateMissing(generateMissingfile);
if (generateMissingfile && isVerbose()) {
StringBuilder sb = new StringBuilder();
sb.append("Will use from missing file ");
sb.append(unsafeMappings.size());
sb.append(" dependencies :");
for (Map.Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy