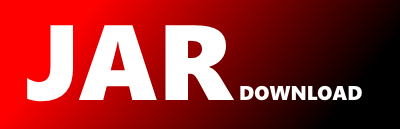
org.nuiton.config.plugin.io.ConfigModelIOIniImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nuiton-config-maven-plugin Show documentation
Show all versions of nuiton-config-maven-plugin Show documentation
Maven plugin to use the config library
The newest version!
package org.nuiton.config.plugin.io;
/*-
* #%L
* Nuiton Config :: Maven plugin
* %%
* Copyright (C) 2016 Code Lutin, Tony Chemit
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import com.google.common.base.Joiner;
import com.google.common.io.Files;
import org.apache.commons.configuration2.INIConfiguration;
import org.apache.commons.configuration2.SubnodeConfiguration;
import org.apache.commons.lang3.StringUtils;
import org.codehaus.plexus.component.annotations.Component;
import org.nuiton.config.plugin.model.ActionModel;
import org.nuiton.config.plugin.model.ConfigModel;
import org.nuiton.config.plugin.model.OptionModel;
import java.io.Reader;
import java.io.Writer;
import java.nio.charset.StandardCharsets;
import java.nio.file.Path;
import java.util.Arrays;
import java.util.LinkedList;
/**
* Implementation using {@code ini} format.
*
* Created on 02/10/16.
*
* @author Tony Chemit - [email protected]
* @since 3.0
*/
@Component(role = ConfigModelIO.class, hint = "ini")
public class ConfigModelIOIniImpl implements ConfigModelIO {
private static final String DESCRIPTION = "description";
private static final String KEY = "key";
private static final String TYPE = "type";
private static final String DEFAULT_VALUE = "defaultValue";
private static final String TRANSIENT = "transient";
private static final String FINAL = "final";
private static final String ACTION = "action";
private static final String ALIASES = "aliases";
@Override
public ConfigModel read(Path path) throws ReadConfigModelException {
try (Reader reader = Files.newReader(path.toFile(), StandardCharsets.UTF_8)) {
INIConfiguration iniConfiguration = new INIConfiguration();
iniConfiguration.read(reader);
ConfigModel configModel = new ConfigModel();
configModel.setDescription(iniConfiguration.getString(DESCRIPTION));
LinkedList options = new LinkedList<>();
LinkedList actions = new LinkedList<>();
configModel.setOptions(options);
configModel.setActions(actions);
for (String section : iniConfiguration.getSections()) {
if (section == null) {
continue;
}
if (section.startsWith("option ")) {
SubnodeConfiguration optionSection = iniConfiguration.getSection(section);
OptionModel optionModel = new OptionModel();
String optionName = StringUtils.removeStart(section, "option ");
optionModel.setName(optionName);
String description = optionSection.getString(DESCRIPTION, "");
optionModel.setDescription(description);
String key = optionSection.getString(KEY);
optionModel.setKey(key);
String type = optionSection.getString(TYPE);
optionModel.setType(type);
String defaultValue = optionSection.getString(DEFAULT_VALUE);
optionModel.setDefaultValue(defaultValue);
String _transient = optionSection.getString(TRANSIENT);
if (StringUtils.isNotEmpty(_transient)) {
optionModel.setTransient(Boolean.valueOf(_transient));
}
String _final = optionSection.getString(FINAL);
if (StringUtils.isNotEmpty(_final)) {
optionModel.setFinal(Boolean.valueOf(_final));
}
options.add(optionModel);
} else if (section.startsWith("action ")) {
SubnodeConfiguration actionSection = iniConfiguration.getSection(section);
ActionModel actionModel = new ActionModel();
String actionName = StringUtils.removeStart(section, "action ");
actionModel.setName(actionName);
String description = actionSection.getString(DESCRIPTION, "");
actionModel.setDescription(description);
String action = actionSection.getString(ACTION);
actionModel.setAction(action);
String aliases = actionSection.getString(ALIASES);
if (StringUtils.isNotEmpty(aliases)) {
actionModel.setAliases(aliases.split("\\s*,\\s*"));
}
actions.add(actionModel);
}
}
return configModel;
} catch (Exception e) {
throw new ReadConfigModelException("Can't real ini config model from file: " + path, e);
}
}
@Override
public void write(ConfigModel configModel, Path path) throws WriteConfigModelException {
try (Writer writer = Files.newWriter(path.toFile(), StandardCharsets.UTF_8)) {
INIConfiguration iniConfiguration = new INIConfiguration();
iniConfiguration.addProperty(DESCRIPTION, configModel.getDescription());
for (OptionModel optionModel : configModel.getOptions()) {
SubnodeConfiguration section = iniConfiguration.getSection("option " + optionModel.getName());
section.addProperty(DESCRIPTION, optionModel.getDescription());
section.addProperty(KEY, optionModel.getKey());
section.addProperty(TYPE, optionModel.getType());
if (optionModel.getDefaultValue() != null) {
section.addProperty(DEFAULT_VALUE, optionModel.getDefaultValue());
}
if (optionModel.isTransient()) {
section.addProperty(TRANSIENT, "true");
}
if (optionModel.isFinal()) {
section.addProperty(FINAL, "true");
}
}
for (ActionModel actionModel : configModel.getActions()) {
SubnodeConfiguration section = iniConfiguration.getSection("action " + actionModel.getName());
section.addProperty(DESCRIPTION, actionModel.getDescription());
section.addProperty(ACTION, actionModel.getAction());
if (actionModel.getAliases().length > 0) {
section.addProperty(ALIASES, Joiner.on(",").join(Arrays.asList(actionModel.getAliases())));
}
}
iniConfiguration.write(writer);
} catch (Exception e) {
throw new WriteConfigModelException("Can't write ini config model from file: " + path, e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy