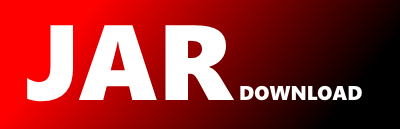
org.nuiton.db.meta.ImmutableDatabaseMeta Maven / Gradle / Ivy
package org.nuiton.db.meta;
/*-
* #%L
* Nuiton DB Meta
* %%
* Copyright (C) 2019 - 2021 Nuiton
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import com.google.common.base.MoreObjects;
import com.google.common.collect.ImmutableList;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link DatabaseMeta}.
*
* Use the builder to create immutable instances:
* {@code ImmutableDatabaseMeta.builder()}.
*/
@Generated(from = "DatabaseMeta", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
@CheckReturnValue
public final class ImmutableDatabaseMeta implements DatabaseMeta {
private final String schema;
private final ImmutableList customTypes;
private final ImmutableList tables;
private final ImmutableList views;
private final ImmutableList procedures;
private ImmutableDatabaseMeta(
String schema,
ImmutableList customTypes,
ImmutableList tables,
ImmutableList views,
ImmutableList procedures) {
this.schema = schema;
this.customTypes = customTypes;
this.tables = tables;
this.views = views;
this.procedures = procedures;
}
/**
* @return The value of the {@code schema} attribute
*/
@Override
public String getSchema() {
return schema;
}
/**
* @return The value of the {@code customTypes} attribute
*/
@Override
public ImmutableList getCustomTypes() {
return customTypes;
}
/**
* @return The value of the {@code tables} attribute
*/
@Override
public ImmutableList getTables() {
return tables;
}
/**
* @return The value of the {@code views} attribute
*/
@Override
public ImmutableList getViews() {
return views;
}
/**
* @return The value of the {@code procedures} attribute
*/
@Override
public ImmutableList getProcedures() {
return procedures;
}
/**
* Copy the current immutable object by setting a value for the {@link DatabaseMeta#getSchema() schema} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for schema
* @return A modified copy of the {@code this} object
*/
public final ImmutableDatabaseMeta withSchema(String value) {
String newValue = Objects.requireNonNull(value, "schema");
if (this.schema.equals(newValue)) return this;
return new ImmutableDatabaseMeta(newValue, this.customTypes, this.tables, this.views, this.procedures);
}
/**
* Copy the current immutable object with elements that replace the content of {@link DatabaseMeta#getCustomTypes() customTypes}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableDatabaseMeta withCustomTypes(CustomTypeMeta... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableDatabaseMeta(this.schema, newValue, this.tables, this.views, this.procedures);
}
/**
* Copy the current immutable object with elements that replace the content of {@link DatabaseMeta#getCustomTypes() customTypes}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of customTypes elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableDatabaseMeta withCustomTypes(Iterable extends CustomTypeMeta> elements) {
if (this.customTypes == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableDatabaseMeta(this.schema, newValue, this.tables, this.views, this.procedures);
}
/**
* Copy the current immutable object with elements that replace the content of {@link DatabaseMeta#getTables() tables}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableDatabaseMeta withTables(TableMeta... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableDatabaseMeta(this.schema, this.customTypes, newValue, this.views, this.procedures);
}
/**
* Copy the current immutable object with elements that replace the content of {@link DatabaseMeta#getTables() tables}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of tables elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableDatabaseMeta withTables(Iterable extends TableMeta> elements) {
if (this.tables == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableDatabaseMeta(this.schema, this.customTypes, newValue, this.views, this.procedures);
}
/**
* Copy the current immutable object with elements that replace the content of {@link DatabaseMeta#getViews() views}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableDatabaseMeta withViews(TableMeta... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableDatabaseMeta(this.schema, this.customTypes, this.tables, newValue, this.procedures);
}
/**
* Copy the current immutable object with elements that replace the content of {@link DatabaseMeta#getViews() views}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of views elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableDatabaseMeta withViews(Iterable extends TableMeta> elements) {
if (this.views == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableDatabaseMeta(this.schema, this.customTypes, this.tables, newValue, this.procedures);
}
/**
* Copy the current immutable object with elements that replace the content of {@link DatabaseMeta#getProcedures() procedures}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableDatabaseMeta withProcedures(ProcedureMeta... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableDatabaseMeta(this.schema, this.customTypes, this.tables, this.views, newValue);
}
/**
* Copy the current immutable object with elements that replace the content of {@link DatabaseMeta#getProcedures() procedures}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of procedures elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableDatabaseMeta withProcedures(Iterable extends ProcedureMeta> elements) {
if (this.procedures == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableDatabaseMeta(this.schema, this.customTypes, this.tables, this.views, newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableDatabaseMeta} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableDatabaseMeta
&& equalTo((ImmutableDatabaseMeta) another);
}
private boolean equalTo(ImmutableDatabaseMeta another) {
return schema.equals(another.schema)
&& customTypes.equals(another.customTypes)
&& tables.equals(another.tables)
&& views.equals(another.views)
&& procedures.equals(another.procedures);
}
/**
* Computes a hash code from attributes: {@code schema}, {@code customTypes}, {@code tables}, {@code views}, {@code procedures}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + schema.hashCode();
h += (h << 5) + customTypes.hashCode();
h += (h << 5) + tables.hashCode();
h += (h << 5) + views.hashCode();
h += (h << 5) + procedures.hashCode();
return h;
}
/**
* Prints the immutable value {@code DatabaseMeta} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("DatabaseMeta")
.omitNullValues()
.add("schema", schema)
.add("customTypes", customTypes)
.add("tables", tables)
.add("views", views)
.add("procedures", procedures)
.toString();
}
/**
* Creates an immutable copy of a {@link DatabaseMeta} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable DatabaseMeta instance
*/
public static ImmutableDatabaseMeta copyOf(DatabaseMeta instance) {
if (instance instanceof ImmutableDatabaseMeta) {
return (ImmutableDatabaseMeta) instance;
}
return ImmutableDatabaseMeta.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableDatabaseMeta ImmutableDatabaseMeta}.
*
* ImmutableDatabaseMeta.builder()
* .schema(String) // required {@link DatabaseMeta#getSchema() schema}
* .addCustomTypes|addAllCustomTypes(org.nuiton.db.meta.CustomTypeMeta) // {@link DatabaseMeta#getCustomTypes() customTypes} elements
* .addTables|addAllTables(org.nuiton.db.meta.TableMeta) // {@link DatabaseMeta#getTables() tables} elements
* .addViews|addAllViews(org.nuiton.db.meta.TableMeta) // {@link DatabaseMeta#getViews() views} elements
* .addProcedures|addAllProcedures(org.nuiton.db.meta.ProcedureMeta) // {@link DatabaseMeta#getProcedures() procedures} elements
* .build();
*
* @return A new ImmutableDatabaseMeta builder
*/
public static ImmutableDatabaseMeta.Builder builder() {
return new ImmutableDatabaseMeta.Builder();
}
/**
* Builds instances of type {@link ImmutableDatabaseMeta ImmutableDatabaseMeta}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "DatabaseMeta", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_SCHEMA = 0x1L;
private long initBits = 0x1L;
private @Nullable String schema;
private ImmutableList.Builder customTypes = ImmutableList.builder();
private ImmutableList.Builder tables = ImmutableList.builder();
private ImmutableList.Builder views = ImmutableList.builder();
private ImmutableList.Builder procedures = ImmutableList.builder();
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code DatabaseMeta} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(DatabaseMeta instance) {
Objects.requireNonNull(instance, "instance");
schema(instance.getSchema());
addAllCustomTypes(instance.getCustomTypes());
addAllTables(instance.getTables());
addAllViews(instance.getViews());
addAllProcedures(instance.getProcedures());
return this;
}
/**
* Initializes the value for the {@link DatabaseMeta#getSchema() schema} attribute.
* @param schema The value for schema
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder schema(String schema) {
this.schema = Objects.requireNonNull(schema, "schema");
initBits &= ~INIT_BIT_SCHEMA;
return this;
}
/**
* Adds one element to {@link DatabaseMeta#getCustomTypes() customTypes} list.
* @param element A customTypes element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addCustomTypes(CustomTypeMeta element) {
this.customTypes.add(element);
return this;
}
/**
* Adds elements to {@link DatabaseMeta#getCustomTypes() customTypes} list.
* @param elements An array of customTypes elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addCustomTypes(CustomTypeMeta... elements) {
this.customTypes.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link DatabaseMeta#getCustomTypes() customTypes} list.
* @param elements An iterable of customTypes elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder customTypes(Iterable extends CustomTypeMeta> elements) {
this.customTypes = ImmutableList.builder();
return addAllCustomTypes(elements);
}
/**
* Adds elements to {@link DatabaseMeta#getCustomTypes() customTypes} list.
* @param elements An iterable of customTypes elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllCustomTypes(Iterable extends CustomTypeMeta> elements) {
this.customTypes.addAll(elements);
return this;
}
/**
* Adds one element to {@link DatabaseMeta#getTables() tables} list.
* @param element A tables element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addTables(TableMeta element) {
this.tables.add(element);
return this;
}
/**
* Adds elements to {@link DatabaseMeta#getTables() tables} list.
* @param elements An array of tables elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addTables(TableMeta... elements) {
this.tables.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link DatabaseMeta#getTables() tables} list.
* @param elements An iterable of tables elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder tables(Iterable extends TableMeta> elements) {
this.tables = ImmutableList.builder();
return addAllTables(elements);
}
/**
* Adds elements to {@link DatabaseMeta#getTables() tables} list.
* @param elements An iterable of tables elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllTables(Iterable extends TableMeta> elements) {
this.tables.addAll(elements);
return this;
}
/**
* Adds one element to {@link DatabaseMeta#getViews() views} list.
* @param element A views element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addViews(TableMeta element) {
this.views.add(element);
return this;
}
/**
* Adds elements to {@link DatabaseMeta#getViews() views} list.
* @param elements An array of views elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addViews(TableMeta... elements) {
this.views.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link DatabaseMeta#getViews() views} list.
* @param elements An iterable of views elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder views(Iterable extends TableMeta> elements) {
this.views = ImmutableList.builder();
return addAllViews(elements);
}
/**
* Adds elements to {@link DatabaseMeta#getViews() views} list.
* @param elements An iterable of views elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllViews(Iterable extends TableMeta> elements) {
this.views.addAll(elements);
return this;
}
/**
* Adds one element to {@link DatabaseMeta#getProcedures() procedures} list.
* @param element A procedures element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addProcedures(ProcedureMeta element) {
this.procedures.add(element);
return this;
}
/**
* Adds elements to {@link DatabaseMeta#getProcedures() procedures} list.
* @param elements An array of procedures elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addProcedures(ProcedureMeta... elements) {
this.procedures.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link DatabaseMeta#getProcedures() procedures} list.
* @param elements An iterable of procedures elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder procedures(Iterable extends ProcedureMeta> elements) {
this.procedures = ImmutableList.builder();
return addAllProcedures(elements);
}
/**
* Adds elements to {@link DatabaseMeta#getProcedures() procedures} list.
* @param elements An iterable of procedures elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllProcedures(Iterable extends ProcedureMeta> elements) {
this.procedures.addAll(elements);
return this;
}
/**
* Builds a new {@link ImmutableDatabaseMeta ImmutableDatabaseMeta}.
* @return An immutable instance of DatabaseMeta
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableDatabaseMeta build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableDatabaseMeta(schema, customTypes.build(), tables.build(), views.build(), procedures.build());
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_SCHEMA) != 0) attributes.add("schema");
return "Cannot build DatabaseMeta, some of required attributes are not set " + attributes;
}
}
}