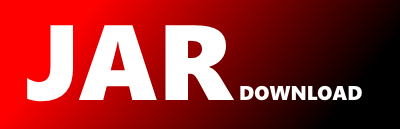
org.nuiton.updater.ApplicationUpdater Maven / Gradle / Ivy
package org.nuiton.updater;
/*
* #%L
* Nuiton Application Updater
* $Id: ApplicationUpdater.java 2588 2013-07-20 14:25:42Z tchemit $
* $HeadURL: https://nuiton.org/svn/nuiton-updater/tags/nuiton-updater-3.0-alpha-2/src/main/java/org/nuiton/updater/ApplicationUpdater.java $
* %%
* Copyright (C) 2013 CodeLutin, Tony Chemit
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import org.apache.commons.io.FileUtils;
import org.apache.commons.lang3.StringUtils;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.nuiton.config.ApplicationConfig;
import org.nuiton.config.ArgumentsParserException;
import java.io.File;
import java.io.IOException;
import java.util.Map;
/**
* Permet de telecharger des mises a jour d'application.
*
* Le principe est qu'un fichier properties pointe par une URL indique les
* information necessaire pour la recuperation de l'application.
*
* Si une nouvelle version de l'application existe, elle est alors telechargee
* et decompressee dans un repertoire specifique (elle ne remplace pas l'application
* courante).
*
* Il est alors a la charge d'un script de mettre en place cette nouvelle application
* a la place de l'ancienne.
*
* Il est possible d'interagir avec ApplicationUpdater via l'implantation d'un
* {@link ApplicationUpdaterCallback} passer en parametre de la methode {@link #update}
*
* Configuration possible
* Vous pouvez passer un ApplicationConfig dans le constructeur ou utiliser
* la recherche du fichier de configuration par defaut (ApplicationUpdater.properties)
*
* Cette configuration permet de récupérer les informations suivantes:
* http_proxy: le proxy a utiliser pour l'acces au reseau (ex: squid.chezmoi.fr:8080)
* os.name: le nom du systeme d'exploitation sur lequel l'application fonctionne (ex: Linux)
* os.arch: l'architecture du systeme d'exploitation sur lequel l'application fonctionne (ex: amd64)
*
* format du fichier de properties
*
* [osName.][osArch.]appName.version=version de l'application
* [osName.][osArch.]appName.auth=true ou false selon que l'acces a l'url
* demande une authentification a fournir par le callback
* (voir {@link ApplicationUpdaterCallback#updateToDo})
* [osName.][osArch.]appName.url=url du fichier compresse de la nouvelle version
* (format commons-vfs2)
*
* appName est a remplacer par le nom de l'application. Il est possible
* d'avoir plusieurs application dans le meme fichier ou plusieurs version
* en fonction de l'os et de l'architecture.
*
* osName et osArch sont toujours en minuscule
*
* format des fichiers compresses
*
* Le fichier compresse doit avoir un repertoire racine qui contient l'ensemble de l'application
* c-a-d que les fichiers ne doivent pas etre directement a la racine lorsqu'on
* decompresse le fichier.
*
* exemple de contenu de fichier compresse convenable
*
* MonApp-0.3/Readme.txt
* MonApp-0.3/License.txt
*
*
* Ceci est du au fait qu'on renomme le repertoire racine avec le nom de l'application,
* donc si le repertoire racine n'existe pas ou qu'il y a plusieurs repertoires
* a la racine le resultat de l'operation n'est pas celui souhaite
*
* os.name and os.arch
*
* os.name os.arch
* linux amd64
* linux i386
* mac ppc
* windows x86
* solaris sparc
*
*
* os.name est tronque apres le 1er mot donc "windows 2000" et "windows 2003"
* deviennet tous les deux "windows". Si vous souhaitez gérer plus finement vos
* url de telechargement vous pouvez modifier les donnees via
* {@link ApplicationUpdaterCallback#updateToDo(java.util.Map) } en modifiant
* l'url avant de retourner la map
*
* @author bpoussin
* @author tchemit
* @since 2.7
*/
public class ApplicationUpdater {
/** Logger. */
private static final Log log = LogFactory.getLog(ApplicationUpdater.class);
protected static final String VERSION_FILE = "version.appup";
protected final static String SEPARATOR_KEY = ".";
public static final String ZERO_VERSION = "0";
protected ApplicationConfig config;
/** Utilise le fichier de configuration par defaut: ApplicationUpdater.properties */
public ApplicationUpdater() {
this(null);
}
/**
* @param config La configuration a utiliser pour rechercher le proxy (http_proxy)
* et os.name, os.arch
*/
public ApplicationUpdater(ApplicationConfig config) {
if (config == null) {
try {
config = new ApplicationConfig(
ApplicationUpdater.class.getSimpleName() + ".properties");
config.parse();
config = config.getSubConfig(
ApplicationUpdater.class.getSimpleName() + SEPARATOR_KEY);
} catch (ArgumentsParserException eee) {
throw new RuntimeException(eee);
}
}
this.config = config;
}
public static File getVersionFile(File dir) {
File versionFile = new File(dir, VERSION_FILE);
return versionFile;
}
public static void createVersionFile(File dir, String version) throws IOException {
File versionFile = getVersionFile(dir);
FileUtils.writeStringToFile(versionFile, version);
}
public static String loadVersionFile(String appName, File dir) {
File f = getVersionFile(dir);
String version = ZERO_VERSION;
try {
version = FileUtils.readFileToString(f);
} catch (IOException ex) {
log.warn(String.format(
"Can't find file version file for application '%s', this file should be '%s'",
appName, f));
}
version = StringUtils.trim(version);
return version;
}
public static void storeVersionFile(File dir, String version) throws IOException {
File f = getVersionFile(dir);
FileUtils.writeStringToFile(f, version);
}
/**
* @param url url where properties file is downloadable. This properties
* must contains information on application release
* @param currentDir directory where application is currently
* @param destDir default directory to put new application version, can be null if you used callback
* @param async if true, check is done in background mode
* @param callback callback used to interact with updater, can be null
*/
public void update(String url,
File currentDir,
File destDir,
boolean async,
ApplicationUpdaterCallback callback) {
update(url, currentDir, destDir, async, callback, null);
}
/**
* @param url url where properties file is downloadable. This properties
* must contains information on application release
* @param currentDir directory where application is currently
* @param destDir default directory to put new application version, can be null if you used callback
* @param async if true, check is done in background mode
* @param callback callback used to interact with updater, can be null
* @param downloadMonitor optinal download monitor
*/
public void update(String url,
File currentDir,
File destDir,
boolean async,
ApplicationUpdaterCallback callback,
DownloadMonitor downloadMonitor) {
ApplicationUpdaterActionUpdate action =
new ApplicationUpdaterActionUpdate(
config,
url,
currentDir,
destDir,
downloadMonitor,
callback);
if (async) {
Thread thread = new Thread(action, ApplicationUpdater.class.getSimpleName());
thread.start();
} else {
action.run();
}
}
/**
* @param url url where properties file is downloadable. This properties
* must contains information on application release
* @param currentDir directory where application is currently
*/
public Map getVersions(String url,
File currentDir) {
ApplicationUpdaterActionGetVersions action =
new ApplicationUpdaterActionGetVersions(
config,
url,
currentDir);
action.run();
Map updates = action.getUpdates();
return updates;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy