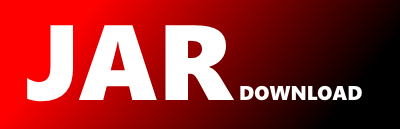
org.nuiton.scmwebeditor.svn.SvnConnection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scmwebeditor-svn Show documentation
Show all versions of scmwebeditor-svn Show documentation
Adds the support of SVN repositories to ScmWebEditor.
The newest version!
/*
* #%L
* ScmWebEditor
* %%
* Copyright (C) 2009 - 2015 CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.scmwebeditor.svn;
import org.apache.commons.io.FileUtils;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.nuiton.scmwebeditor.api.ScmConnection;
import org.nuiton.scmwebeditor.api.ScmRevision;
import org.nuiton.scmwebeditor.api.dto.BrowseDto;
import org.nuiton.scmwebeditor.api.dto.CommitDto;
import org.nuiton.scmwebeditor.api.dto.result.BrowseResultDto;
import org.nuiton.scmwebeditor.api.dto.result.CommitResultDto;
import org.nuiton.util.FileUtil;
import org.tmatesoft.svn.core.*;
import org.tmatesoft.svn.core.auth.ISVNAuthenticationManager;
import org.tmatesoft.svn.core.internal.io.dav.DAVRepositoryFactory;
import org.tmatesoft.svn.core.internal.util.SVNEncodingUtil;
import org.tmatesoft.svn.core.internal.wc.DefaultSVNOptions;
import org.tmatesoft.svn.core.io.SVNFileRevision;
import org.tmatesoft.svn.core.io.SVNRepository;
import org.tmatesoft.svn.core.io.SVNRepositoryFactory;
import org.tmatesoft.svn.core.wc.*;
import javax.naming.AuthenticationException;
import java.io.*;
import java.text.DateFormat;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Collection;
import java.util.Date;
import java.util.Map;
import java.util.TreeMap;
/**
* Implementation of the SVN's main features
*/
public class SvnConnection implements ScmConnection {
private static final Log log = LogFactory.getLog(SvnConnection.class);
/** full svn path */
protected String addressSvn;
/** svn path without fileName */
protected String svnPath;
/** fileName of modif file */
protected String fileName;
/** Temp directory for checkout */
protected File checkoutdir;
/** url of svn path */
protected SVNURL remoteUrl;
/** AuthenticationManager with login and password */
protected ISVNAuthenticationManager authManager;
/** svn default option */
protected DefaultSVNOptions svnOption;
/** the path to the local repositories */
protected String pathToLocalRepos;
/** the manager which allows authentication */
protected SVNClientManager manager;
public String getSvnPath() { return svnPath; }
public DefaultSVNOptions getSvnOption() { return svnOption; }
public String getPathToLocalRepos() { return pathToLocalRepos; }
public void setPathToLocalRepos(String pathToLocalRepos) { this.pathToLocalRepos = pathToLocalRepos; }
public SVNClientManager getManager() { return manager; }
public SvnConnection(String address, String pathToLocalRepos) throws SVNException {
if(log.isDebugEnabled()) {
log.debug("SVN repository");
}
if (address.lastIndexOf('/') != -1){
svnPath = address.substring(0, address.lastIndexOf('/'));
fileName = address.substring(address.lastIndexOf('/') + 1);
}
addressSvn = address;
remoteUrl = SVNURL.parseURIEncoded(svnPath);
authManager = SVNWCUtil.createDefaultAuthenticationManager();
svnOption = SVNWCUtil.createDefaultOptions(false);
svnOption.setPropertyValue(SVNProperty.EOL_STYLE, SVNProperty.EOL_STYLE_LF);
manager = SVNClientManager.newInstance(svnOption, authManager);
this.pathToLocalRepos = pathToLocalRepos;
}
@Override
public BrowseResultDto browse(BrowseDto dto) {
BrowseResultDto resultDto = new BrowseResultDto();
String url;
DAVRepositoryFactory.setup();
SVNRepository repository;
ISVNAuthenticationManager svnAuthManager;
String name = dto.getUsername();
String password = dto.getPassword();
if (dto.getId().equals("")) {
try {
if (log.isDebugEnabled()) {
log.debug("Address svn : " + dto.getAddress());
}
String encodedUrl = SVNEncodingUtil.autoURIEncode(addressSvn);
repository = SVNRepositoryFactory.create(SVNURL.parseURIEncoded(encodedUrl));
svnAuthManager = SVNWCUtil.createDefaultAuthenticationManager(name, password);
repository.setAuthenticationManager(svnAuthManager);
repository.testConnection();
} catch (SVNAuthenticationException e) {
if (log.isErrorEnabled()) {
log.error("Can't access to the repository : Auth Problem", e);
}
resultDto.setError(BrowseResultDto.AUTH_ERROR);
return resultDto;
} catch (SVNException e) {
if (log.isErrorEnabled()) {
log.error("Can't access to the repository", e);
}
resultDto.setError(BrowseResultDto.ERROR);
return resultDto;
}
resultDto.setError(BrowseResultDto.ROOT);
return resultDto;
} else if (dto.getId().equals("0")) {
url = dto.getAddress();
} else {
url = dto.getId();
dto.setAddress(dto.getId());
}
try {
String encodedUrl = SVNEncodingUtil.autoURIEncode(url);
repository = SVNRepositoryFactory.create(SVNURL.parseURIEncoded(encodedUrl));
svnAuthManager = SVNWCUtil.createDefaultAuthenticationManager(name, password);
repository.setAuthenticationManager(svnAuthManager);
if (log.isDebugEnabled()) {
log.debug("Repository Root: " + repository.getRepositoryRoot(true));
log.debug("Repository UUID: " + repository.getRepositoryUUID(true));
}
SVNNodeKind nodeKind = repository.checkPath("", -1);
if (nodeKind == SVNNodeKind.NONE) {
if (log.isWarnEnabled()) {
log.warn("There is no entry at '" + url + "'.");
}
resultDto.setError(BrowseResultDto.ERROR);
return resultDto;
} else if (nodeKind == SVNNodeKind.FILE) {
if (log.isDebugEnabled()) {
log.debug("The entry at '" + url + "' is a file.");
}
return resultDto;
}
listEntries(repository, "", dto.getAddress(), resultDto);
if (log.isDebugEnabled()) {
log.debug("Number of file : " + resultDto.getFiles().size());
}
} catch (SVNAuthenticationException authexep) {
if (log.isErrorEnabled()) {
log.error("Can't access to the repository : Auth Problem");
}
resultDto.setError(BrowseResultDto.AUTH_ERROR);
return resultDto;
} catch (SVNException svne) {
if (log.isErrorEnabled()) {
log.error("Can't access to the repository");
}
resultDto.setError(BrowseResultDto.ERROR);
return resultDto;
}
if (log.isDebugEnabled()) {
log.debug("Search success");
}
resultDto.setError(null);
return resultDto;
}
@Override
public CommitResultDto commit(CommitDto dto) {
try {
createCheckoutdir();
} catch (IOException e) {
if (log.isErrorEnabled()) {
log.error("Can not create checkoutdir", e);
}
}
CommitResultDto resultDto = new CommitResultDto();
updateAuthentication(dto.getUsername(), dto.getPassword());
if (log.isDebugEnabled()) {
log.debug("Entering SVN commit");
}
try {
createCheckoutdir();
} catch (IOException e1) {
if (log.isErrorEnabled()) {
log.error("Can't create checkoutDir", e1);
}
resultDto.setError(CommitResultDto.ERROR);
return resultDto;
}
// before the commit, we have to checkout the repository
try {
checkout(checkoutdir);
} catch (SVNAuthenticationException authexep) {
// if svn authentication failed user is redirected on login page
if (log.isDebugEnabled()) {
log.debug("Private SCM on reading " + remoteUrl);
}
// we delete the temporary directory
delTempDirectory(checkoutdir);
resultDto.setError(CommitResultDto.AUTH_ERROR);
return resultDto;
} catch (SVNException e) {
if (log.isErrorEnabled()) {
log.error("Can't checkout the file", e);
}
// deleting the temporary directory
delTempDirectory(checkoutdir);
resultDto.setError(CommitResultDto.ERROR_PATH);
return resultDto;
}
File checkOutFile = new File(checkoutdir, fileName);
resultDto.setLastText(dto.getNewText());
try {
String originalText = FileUtils.readFileToString(checkOutFile);
resultDto.setOrigText(originalText);
} catch (FileNotFoundException ee) {
/* file not found, redirecting to BadFileRedirect.jsp
* after deleting the temporary directory
*/
delTempDirectory(checkoutdir);
resultDto.setError(CommitResultDto.ERROR);
return resultDto;
} catch (IOException e) {
log.error("Can't find the checkout file", e);
}
/*
* Diff
*/
if (!dto.isForce()) {
try {
if (isDifferent(resultDto.getOrigText())) {
ByteArrayOutputStream differents = getDiff(dto.getNewText());
if (differents.size() > 0) {
resultDto.setDiff(differents.toString("UTF-8"));
String diff = resultDto.getDiff();
resultDto.setDiff(diff.substring(diff.indexOf("@@")));
delTempDirectory(checkoutdir);
try {
resultDto.setHeadCommiter(getHeadcommiter(dto.getAddress(), dto.getUsername(), dto.getPassword()));
} catch (SVNException e) {
log.error("Can't get head commiter", e);
}
resultDto.setError(CommitResultDto.FILE_MODIFY);
return resultDto;
}
}
} catch (IOException e) {
if (log.isErrorEnabled()) {
log.error("Can't do diff on file, IO error", e);
}
}
}
/*
* Commit process
*/
SVNCommitClient commitClient = new SVNCommitClient(manager, svnOption);
File pathToFile = new File(checkoutdir, fileName);
try {
Writer writer = FileUtil.getWriter(pathToFile, "UTF-8");
writer.write(dto.getNewText());
writer.flush();
} catch (IOException e1) {
delTempDirectory(checkoutdir);
resultDto.setError(CommitResultDto.ERROR);
return resultDto;
}
File[] tabFile = new File[1];
tabFile[0] = pathToFile;
try {
if (log.isDebugEnabled()) {
log.debug("Try to commit");
}
commitClient.doCommit(tabFile, false, "From scmwebeditor -- " + dto.getCommitMessage(), null, null, false,
false, SVNDepth.FILES);
} catch (SVNAuthenticationException authexep) {
if (log.isErrorEnabled()) {
log.error("AUTH FAIL", authexep);
}
// if authentication failed edition page is reloaded form user's relogin
resultDto.setOrigText(dto.getNewText());
// deleting temporary directory
delTempDirectory(checkoutdir);
dto.setUsername(null);
dto.setPassword(null);
resultDto.setError(CommitResultDto.AUTH_ERROR);
return resultDto;
} catch (SVNException e) {
if (log.isErrorEnabled()) {
log.error("SVN FAIL", e);
}
// deleting temporary directory
delTempDirectory(checkoutdir);
resultDto.setError(CommitResultDto.ERROR);
return resultDto;
}
if (checkoutdir != null) {
// deleting temporary directory
delTempDirectory(checkoutdir);
}
if (log.isDebugEnabled()) {
log.debug("End of commit");
}
// deleting temporary directory
delTempDirectory(checkoutdir);
if (log.isInfoEnabled()) {
log.info(dto.getUsername() + " commit the file " + dto.getAddress() + " with message : "
+ dto.getCommitMessage());
}
try {
resultDto.setNumRevision(getHeadRevisionNumber(dto.getAddress(), dto.getUsername(), dto.getPassword()));
} catch (AuthenticationException e) {
if (log.isErrorEnabled()) {
log.error("Auth fail", e);
}
}
return resultDto;
}
@Override
public File getFileContent(String path, String username, String password) throws AuthenticationException {
File fileContent = getFileContentAtRevision(path, username, password, "-1");
return fileContent;
}
@Override
public String getHeadRevisionNumber(String path, String username, String password) throws AuthenticationException {
if (log.isDebugEnabled()) {
log.debug("headRevisionNumber expected " + addressSvn + " ; got " + path);
}
ISVNAuthenticationManager svnAuthManager = SVNWCUtil.createDefaultAuthenticationManager(username, password);
DefaultSVNOptions svnOptions = new DefaultSVNOptions();
svnOptions.setPropertyValue(SVNProperty.EOL_STYLE, SVNProperty.EOL_STYLE_LF);
SVNWCClient wcClient = new SVNWCClient(svnAuthManager, svnOptions);
SVNInfo info = null;
try {
info = wcClient.doInfo(SVNURL.parseURIEncoded(path), SVNRevision.HEAD, SVNRevision.HEAD);
} catch (SVNAuthenticationException e) {
throw new AuthenticationException("Auth fail");
} catch (SVNException e) {
if (log.isErrorEnabled()) {
log.error("Can not get info from SVN repository", e);
}
}
String headRevision = "";
if (info != null) {
headRevision = info.getRevision().toString();
}
return headRevision;
}
@Override
public String getRepositoryId() {
String repositoryUUID;
try {
String encodedUrl = SVNEncodingUtil.autoURIEncode(addressSvn);
SVNRepository repository = SVNRepositoryFactory.create(SVNURL.parseURIEncoded(encodedUrl));
ISVNAuthenticationManager svnAuthManager = SVNWCUtil.createDefaultAuthenticationManager();
repository.setAuthenticationManager(svnAuthManager);
repositoryUUID = repository.getRepositoryUUID(true);
} catch (SVNException e) {
if (log.isDebugEnabled()) {
log.debug("Can't get UUID", e);
}
return null;
}
return repositoryUUID;
}
@Override
public String getFileName() {
return fileName;
}
@Override
public String getFilePath(String address, String repositoryRoot, String username, String password) {
String path = address;
if (address.toLowerCase().endsWith(".svg")) {
File svgFile = null;
try {
svgFile = getFileContent(address, username, password);
} catch (AuthenticationException e) {
if (log.isErrorEnabled()) {
log.error("Can not get file content for address " + address);
}
}
if (svgFile != null) {
path = svgFile.getAbsolutePath();
}
}
return path;
}
@Override
public Map getRevisions(String address, String username, String password) throws AuthenticationException {
final int MAX_MESSAGE_LENGTH = 64;
String url = address.substring(0, address.lastIndexOf('/'));
String file = address.substring(address.lastIndexOf('/') + 1);
Map revisions = new TreeMap();
updateAuthentication(username, password);
SVNRepository repository;
try {
// getting the revisions
repository = SVNRepositoryFactory.create(SVNURL.parseURIEncoded(url));
ISVNAuthenticationManager svnAuthManager = SVNWCUtil.createDefaultAuthenticationManager(username, password);
repository.setAuthenticationManager(svnAuthManager);
SVNNodeKind nodeKind = repository.checkPath(file, -1);
if (nodeKind == SVNNodeKind.NONE) {
if (log.isErrorEnabled()) {
log.error("There is no entry at '" + address + "'.");
}
throw new IllegalArgumentException("There is no entry at '" + address + "'.");
} else if (nodeKind == SVNNodeKind.DIR) {
if (log.isErrorEnabled()) {
log.error("The entry at '" + address + "' is a file while a directory was expected.");
}
throw new IllegalArgumentException("The entry at '" + address + "' is a directory while a file was expected.");
}
Collection fileRevisions;
fileRevisions = repository.getFileRevisions(file, null, 0, repository.getLatestRevision());
// making the value and the key to put in the Map
for (Object fileRev : fileRevisions) {
// getting the commit message
String msg = ((SVNFileRevision) fileRev).getRevisionProperties().getStringValue(SVNRevisionProperty.LOG);
if (msg.length() > MAX_MESSAGE_LENGTH) {
msg = msg.substring(0, MAX_MESSAGE_LENGTH) + "...";
}
long revNum = ((SVNFileRevision) fileRev).getRevision();
msg = " (rev " + revNum + ") - " + msg;
// getting the date
String date = ((SVNFileRevision) fileRev).getRevisionProperties().getStringValue(SVNRevisionProperty.DATE);
DateFormat svnDf = new SimpleDateFormat("yyyy-MM-dd'T'HH:mm:ss.SSSSSS'Z'");
Date commitDate = null;
try {
commitDate = svnDf.parse(date);
} catch (ParseException e) {
if (log.isErrorEnabled()) {
log.error("Can not parse date " + date);
}
}
DateFormat displayDf = new SimpleDateFormat("dd/MM/yyyy HH:mm");
String formattedDate = displayDf.format(commitDate);
msg = formattedDate + " " + msg;
// adding the revision to the Map
ScmRevision scmRev;
if (commitDate != null) {
scmRev = new ScmRevision(String.valueOf(revNum), commitDate.getTime());
revisions.put(scmRev, msg);
}
}
} catch (SVNAuthenticationException e) {
throw new AuthenticationException("Auth fail");
} catch (SVNException e) {
if (log.isErrorEnabled()) {
log.error("Can not get revisions from SVN repository for address " + address, e);
}
}
return revisions;
}
@Override
public File getFileContentAtRevision(String path, String username, String password,
String revision) throws AuthenticationException {
String url = path.substring(0, path.lastIndexOf('/'));
String file = path.substring(path.lastIndexOf('/') + 1);
long rev = Long.parseLong(revision);
// storing the file content to the user's local directory
File localDirectory = new File(pathToLocalRepos);
if (!localDirectory.exists()) {
boolean localDirectoryCreated = localDirectory.mkdir();
if (!localDirectoryCreated && log.isDebugEnabled()) {
log.debug("Could not create directory " + localDirectory.getAbsolutePath());
}
}
String tempFileName = localDirectory.getAbsolutePath() + File.separator + file;
File tempFile = new File(tempFileName);
if (tempFile.exists()) {
boolean tempFileDeleted = tempFile.delete();
if (!tempFileDeleted && log.isDebugEnabled()) {
log.debug("Could not delete temp file " + tempFileName);
}
}
updateAuthentication(username, password);
SVNRepository repository;
try {
repository = SVNRepositoryFactory.create(SVNURL.parseURIEncoded(url));
ISVNAuthenticationManager svnAuthManager = SVNWCUtil.createDefaultAuthenticationManager(username, password);
repository.setAuthenticationManager(svnAuthManager);
SVNNodeKind nodeKind = repository.checkPath(file, rev);
if (nodeKind == SVNNodeKind.NONE) {
if (log.isErrorEnabled()) {
log.error("There is no entry at '" + url + "'.");
}
return null;
} else if (nodeKind == SVNNodeKind.DIR) {
if (log.isErrorEnabled()) {
log.error("The entry at '" + url + "' is a file while a directory was expected.");
}
return null;
}
// writing the result to a temp file
ByteArrayOutputStream baos = new ByteArrayOutputStream();
SVNProperties fileProperties = new SVNProperties();
repository.getFile(file, rev, fileProperties, baos);
fileProperties.getStringValue(SVNProperty.REVISION);
try {
OutputStream fileOutput = new FileOutputStream(tempFileName);
baos.writeTo(fileOutput);
} catch (FileNotFoundException e) {
if (log.isErrorEnabled()) {
log.error("Can not find file " + tempFileName, e);
}
} catch (IOException e) {
if (log.isErrorEnabled()) {
log.error("Can not write to file " + tempFileName, e);
}
}
try {
baos.close();
} catch (IOException e) {
if (log.isDebugEnabled()) {
log.debug("Can't close stream", e);
}
}
} catch (SVNAuthenticationException e) {
throw new AuthenticationException("Auth fail");
} catch (SVNException e) {
if (log.isErrorEnabled()) {
log.error("Can not get file content from SVN repository", e);
}
}
return tempFile;
}
@Override
public File getDiffs(String path, String username, String password,
String revision1, String revision2) throws AuthenticationException {
String url = path.substring(0, path.lastIndexOf('/'));
String file = path.substring(path.lastIndexOf('/') + 1);
long rev1 = Long.parseLong(revision1);
long rev2 = Long.parseLong(revision2);
// storing the file content to the user's local directory
File localDirectory = new File(pathToLocalRepos);
if (!localDirectory.exists()) {
boolean localDirectoryCreated = localDirectory.mkdir();
if (!localDirectoryCreated && log.isDebugEnabled()) {
log.debug("Could not create directory " + localDirectory.getAbsolutePath());
}
}
String tempFileName = localDirectory.getAbsolutePath() + File.separator + file;
File tempFile = new File(tempFileName);
if (tempFile.exists()) {
boolean tempFileDeleted = tempFile.delete();
if (!tempFileDeleted && log.isDebugEnabled()) {
log.debug("Could not delete temp file " + tempFileName);
}
}
updateAuthentication(username, password);
SVNRepository repository;
try {
repository = SVNRepositoryFactory.create(SVNURL.parseURIEncoded(url));
ISVNAuthenticationManager svnAuthManager = SVNWCUtil.createDefaultAuthenticationManager(username, password);
repository.setAuthenticationManager(svnAuthManager);
SVNNodeKind nodeKind1 = repository.checkPath(file, rev1);
SVNNodeKind nodeKind2 = repository.checkPath(file, rev2);
if (nodeKind1 == SVNNodeKind.NONE || nodeKind2 == SVNNodeKind.NONE) {
if (log.isErrorEnabled()) {
log.error("There is no entry at '" + url + "'.");
}
return null;
} else if (nodeKind1 == SVNNodeKind.DIR || nodeKind2 == SVNNodeKind.DIR) {
if (log.isErrorEnabled()) {
log.error("The entry at '" + url + "' is a file while a directory was expected.");
}
return null;
}
// writing the result to a temp file
ByteArrayOutputStream baos = new ByteArrayOutputStream();
SVNDiffClient diffClient = new SVNDiffClient(authManager, null);
String encodedUrl = SVNEncodingUtil.autoURIEncode(addressSvn);
SVNURL fileUrl = SVNURL.parseURIEncoded(encodedUrl);
SVNRevision svnRev1 = SVNRevision.create(rev1);
SVNRevision svnRev2 = SVNRevision.create(rev2);
diffClient.doDiff(fileUrl, SVNRevision.HEAD, svnRev2, svnRev1, SVNDepth.INFINITY, false, baos);
try {
OutputStream fileOutput = new FileOutputStream(tempFileName);
baos.writeTo(fileOutput);
} catch (FileNotFoundException e) {
if (log.isErrorEnabled()) {
log.error("Can not find file " + tempFileName, e);
}
} catch (IOException e) {
if (log.isErrorEnabled()) {
log.error("Can not write to file " + tempFileName, e);
}
}
try {
baos.close();
} catch (IOException e) {
if (log.isDebugEnabled()) {
log.debug("Can't close stream", e);
}
}
} catch (SVNAuthenticationException e) {
throw new AuthenticationException("Auth fail");
} catch (SVNException e) {
if (log.isErrorEnabled()) {
log.error("Can not get file content from SVN repository", e);
}
}
return tempFile;
}
public String getSvnRoot(String username, String password) {
String repositoryRoot;
try {
String encodedUrl = SVNEncodingUtil.autoURIEncode(addressSvn);
SVNRepository repository = SVNRepositoryFactory.create(SVNURL.parseURIEncoded(encodedUrl));
ISVNAuthenticationManager svnAuthManager = SVNWCUtil.createDefaultAuthenticationManager(username, password);
repository.setAuthenticationManager(svnAuthManager);
repositoryRoot = repository.getRepositoryRoot(true).toString();
} catch (SVNException e) {
if (log.isDebugEnabled()) {
log.debug("Can't get SvnRoot for address " + addressSvn, e);
}
return null;
}
return repositoryRoot;
}
public void updateAuthentication(String login, String password) {
authManager = SVNWCUtil.createDefaultAuthenticationManager(login, password);
manager = SVNClientManager.newInstance(svnOption, authManager);
}
public void testConnection() throws SVNException {
String encodedUrl = SVNEncodingUtil.autoURIEncode(addressSvn);
SVNRepository repository = SVNRepositoryFactory.create(SVNURL.parseURIEncoded(encodedUrl));
repository.setAuthenticationManager(authManager);
repository.testConnection();
}
public boolean isDifferent(String text) throws IOException {
ByteArrayOutputStream diff = getDiff(text);
boolean positiveSize = (diff.size() > 0);
return positiveSize;
}
public ByteArrayOutputStream getDiff(String text) throws IOException {
File pathToFile = new File(checkoutdir, fileName);
SVNDiffClient diffClient = new SVNDiffClient(manager, svnOption);
FileUtils.writeStringToFile(pathToFile, text, "UTF-8");
ByteArrayOutputStream diff = new ByteArrayOutputStream();
try {
diffClient.doDiff(pathToFile, SVNRevision.UNDEFINED, SVNRevision.WORKING, SVNRevision.HEAD,
SVNDepth.INFINITY, true, diff, null);
} catch (SVNException e) {
log.error("Diff fail", e);
}
return diff;
}
/**
* @param checkoutdir the directory where the checkout will be done
* @throws SVNException if the checkout could not be done
*/
public void checkout(File checkoutdir) throws SVNException {
SVNUpdateClient upclient = new SVNUpdateClient(manager, svnOption);
if (log.isDebugEnabled()) {
log.debug("Do Checkout of " + remoteUrl);
}
upclient.doCheckout(remoteUrl, checkoutdir,
SVNRevision.HEAD, SVNRevision.HEAD, SVNDepth.FILES, false);
}
public void createCheckoutdir() throws IOException {
File localDirectory = new File(pathToLocalRepos);
if (!localDirectory.exists()) {
boolean localDirectoryCreated = localDirectory.mkdir();
if (!localDirectoryCreated && log.isDebugEnabled()) {
log.debug("Could not create directory " + localDirectory.getAbsolutePath());
}
}
checkoutdir = FileUtil.createTempDirectory("scm_", "", localDirectory);
}
/**
* Use to delete the checkout temp directory
*
* @param checkoutdir The dir temp directory
*/
public void delTempDirectory(File checkoutdir) {
if (checkoutdir != null) {
try {
FileUtils.deleteDirectory(checkoutdir);
} catch (IOException e) {
if (log.isErrorEnabled()) {
log.error("Can't delete temp directory");
}
}
}
}
/**
* Gives the name of the person who made the last commit
* @param address the repository's address
* @param login the username to use to connect to the repository
* @param password the password to use to connect to the repository
* @return the name of the person who made the last commit
* @throws SVNException if there is a problem while accessing the repository
*/
public String getHeadcommiter(String address, String login, String password) throws SVNException {
ISVNAuthenticationManager svnAuthManager = SVNWCUtil.createDefaultAuthenticationManager(login, password);
DefaultSVNOptions svnOptions = new DefaultSVNOptions();
svnOptions.setPropertyValue(SVNProperty.EOL_STYLE, SVNProperty.EOL_STYLE_LF);
SVNWCClient wcClient = new SVNWCClient(svnAuthManager, svnOptions);
SVNInfo info = wcClient.doInfo(SVNURL.parseURIEncoded(address), SVNRevision.HEAD, SVNRevision.HEAD);
String headAuthor = info.getAuthor();
return headAuthor;
}
/**
* Makes a list of the files and directories in the repository
* @param repository the repository to use to fetch information
* @param path the path to the directory (in the repository) to make the list from
* @param address the repository's address
* @param resultDto the DTO which will contain the files and directories found
*/
public void listEntries(SVNRepository repository, String path, String address, BrowseResultDto resultDto) {
Collection entries = null;
try {
entries = repository.getDir(path, -1, null, (Collection) null);
} catch (SVNException e) {
if (log.isErrorEnabled()) {
log.error("Can not reach the repository", e);
}
}
if (entries != null) {
for (Object entry : entries) {
if (log.isDebugEnabled()) {
log.debug("/" + (path.equals("") ? "" : path + "/") + ((SVNDirEntry) entry).getName() + "\n");
}
String file = address + File.separator + (path.equals("") ? "" : path + File.separator) +
((SVNDirEntry) entry).getName();
if (((SVNDirEntry) entry).getKind() == SVNNodeKind.DIR) {
resultDto.getDirectories().put(getAddressUnique(file), file);
} else {
// only adding the files to the files list and not the directories
resultDto.getFiles().add(file);
}
}
}
}
/**
* Gives a unique address for the given file name
* @param fileName the name of the file
* @return a unique address for the file name
*/
public String getAddressUnique(String fileName) {
String result = fileName.replaceAll("/|:", "");
if (log.isDebugEnabled()) {
log.debug("Result of getAddressUnique : " + result);
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy