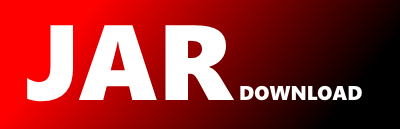
org.nuiton.scmwebeditor.svn.SvnFileManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scmwebeditor-svn Show documentation
Show all versions of scmwebeditor-svn Show documentation
Adds the support of SVN repositories to ScmWebEditor.
The newest version!
/*
* #%L
* ScmWebEditor
* %%
* Copyright (C) 2009 - 2015 CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.scmwebeditor.svn;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.nuiton.scmwebeditor.api.ScmFileManager;
import org.nuiton.scmwebeditor.api.dto.*;
import org.nuiton.scmwebeditor.api.dto.result.*;
import org.tmatesoft.svn.core.SVNAuthenticationException;
import org.tmatesoft.svn.core.SVNDepth;
import org.tmatesoft.svn.core.SVNException;
import org.tmatesoft.svn.core.SVNURL;
import org.tmatesoft.svn.core.wc.SVNCommitClient;
import org.tmatesoft.svn.core.wc.SVNCopyClient;
import org.tmatesoft.svn.core.wc.SVNCopySource;
import org.tmatesoft.svn.core.wc.SVNRevision;
/**
* Implementation of the SVN's features related to file management
*/
public class SvnFileManager implements ScmFileManager {
private static final Log log = LogFactory.getLog(SvnFileManager.class);
/** the connection to the Git repository */
SvnConnection connection;
public SvnFileManager(SvnConnection connection) throws SVNException {
if(log.isDebugEnabled()) {
log.debug("SVN repository");
}
this.connection = connection;
}
@Override
public UploadFileResultDto uploadFile(UploadFileDto dto) {
UploadFileResultDto resultDto = new UploadFileResultDto();
if (dto.getUsername() == null) {
dto.setUsername("anonymous");
}
if (dto.getPassword() == null) {
dto.setPassword("anonymous");
}
resultDto.setScmRoot(connection.getSvnRoot(dto.getUsername(), dto.getPassword()));
if (resultDto.getScmRoot() == null) {
resultDto.setScmRoot(connection.getSvnPath());
}
if (connection.getSvnPath().endsWith("/")) {
resultDto.setFileRoot(connection.getSvnPath().substring(0, connection.getSvnPath().lastIndexOf('/')));
} else {
resultDto.setFileRoot(connection.getSvnPath());
}
connection.updateAuthentication(dto.getUsername(), dto.getPassword());
try {
connection.testConnection();
} catch (SVNException e) {
if (log.isDebugEnabled()) {
log.debug("Test connection fail", e);
}
resultDto.setError(UploadFileResultDto.CONNECTION_FAILED);
return resultDto;
}
// if there is no file to upload we get back to the upload form
if (dto.getUpload() == null) {
resultDto.setError(UploadFileResultDto.REDIRECT);
return resultDto;
}
if (log.isDebugEnabled()) {
log.debug("FileName : " + dto.getUploadFileName());
log.debug("ContentType : " + dto.getUploadContentType());
}
SVNCommitClient commitClient = new SVNCommitClient(connection.getManager(), connection.getSvnOption());
try {
commitClient.doImport(dto.getUpload(), SVNURL.parseURIEncoded(dto.getScmPath() + "/" + dto.getUploadFileName()),
"From scmwebeditor -- add the file : " + dto.getUploadFileName(), null, false, false, SVNDepth.EMPTY);
} catch (SVNAuthenticationException authexep) {
if (log.isErrorEnabled()) {
log.error("authentication fail", authexep);
}
resultDto.setError(UploadFileResultDto.AUTH_ERROR);
return resultDto;
} catch (SVNException e) {
if (log.isErrorEnabled()) {
log.error("Error SVN import", e);
}
resultDto.setError(UploadFileResultDto.ERROR);
return resultDto;
}
return resultDto;
}
@Override
public RemoveFileResultDto removeFile(RemoveFileDto dto) {
RemoveFileResultDto resultDto = new RemoveFileResultDto();
if (dto.getUsername() == null) {
dto.setUsername("anonymous");
}
if (dto.getPassword() == null) {
dto.setPassword("anonymous");
}
resultDto.setScmRoot(connection.getSvnRoot(dto.getUsername(), dto.getPassword()));
if (resultDto.getScmRoot() == null) {
resultDto.setScmRoot(connection.getSvnPath());
}
if (connection.getSvnPath().endsWith("/")) {
resultDto.setFileRoot(connection.getSvnPath().substring(0, connection.getSvnPath().lastIndexOf('/')));
} else {
resultDto.setFileRoot(connection.getSvnPath());
}
connection.updateAuthentication(dto.getUsername(), dto.getPassword());
try {
connection.testConnection();
} catch (SVNException e) {
if (log.isDebugEnabled()) {
log.debug("Test connection fail", e);
}
resultDto.setError(RemoveFileResultDto.CONNECTION_FAILED);
return resultDto;
}
// if there is no file to remove we get back to the remove form
if (dto.getScmPath() == null) {
resultDto.setError(RemoveFileResultDto.REDIRECT);
return resultDto;
}
SVNCommitClient commitClient = new SVNCommitClient(connection.getManager(), connection.getSvnOption());
try {
SVNURL scmPathTab[] = new SVNURL[1];
scmPathTab[0] = SVNURL.parseURIEncoded(dto.getScmPath());
commitClient.doDelete(scmPathTab, "From scmwebeditor -- remove the file : " + dto.getScmPath());
} catch (SVNAuthenticationException authexep) {
if (log.isErrorEnabled()) {
log.error("authentication fail", authexep);
}
resultDto.setError(RemoveFileResultDto.AUTH_ERROR);
return resultDto;
} catch (SVNException e) {
if (log.isErrorEnabled()) {
log.error("Error SVN import", e);
}
resultDto.setError(RemoveFileResultDto.ERROR);
return resultDto;
}
return resultDto;
}
@Override
public CreateDirectoryResultDto createDirectory(CreateDirectoryDto dto) {
CreateDirectoryResultDto resultDto = new CreateDirectoryResultDto();
if (dto.getUsername() == null) {
dto.setUsername("anonymous");
}
if (dto.getPassword() == null) {
dto.setPassword("anonymous");
}
resultDto.setScmRoot(connection.getSvnRoot(dto.getUsername(), dto.getPassword()));
if (resultDto.getScmRoot() == null) {
resultDto.setScmRoot(connection.getSvnPath());
}
if (connection.getSvnPath().endsWith("/")) {
resultDto.setFileRoot(connection.getSvnPath().substring(0, connection.getSvnPath().lastIndexOf('/')));
} else {
resultDto.setFileRoot(connection.getSvnPath());
}
connection.updateAuthentication(dto.getUsername(), dto.getPassword());
try {
connection.testConnection();
} catch (SVNException e) {
if (log.isDebugEnabled()) {
log.debug("Test connection fail", e);
}
resultDto.setError(CreateDirectoryResultDto.CONNECTION_FAILED);
return resultDto;
}
// if the name of the new directory is empty we get back to the create directory form
if (dto.getDirectoryName() == null) {
resultDto.setError(CreateDirectoryResultDto.REDIRECT);
return resultDto;
}
if (dto.getDirectoryName().equals("")) {
resultDto.setError(CreateDirectoryResultDto.REDIRECT);
return resultDto;
}
SVNCommitClient commitClient = new SVNCommitClient(connection.getManager(), connection.getSvnOption());
try {
SVNURL[] urls = new SVNURL[1];
urls[0] = SVNURL.parseURIEncoded(dto.getParentDirectory() + "/" + dto.getDirectoryName());
commitClient.doMkDir(urls, "From scmwebeditor -- create the directory : " + dto.getDirectoryName());
} catch (SVNAuthenticationException authexep) {
if (log.isErrorEnabled()) {
log.error("authentication fail", authexep);
}
resultDto.setError(CreateDirectoryResultDto.AUTH_ERROR);
return resultDto;
} catch (SVNException e) {
if (log.isErrorEnabled()) {
log.error("Error SVN import", e);
}
resultDto.setError(CreateDirectoryResultDto.ERROR);
return resultDto;
}
return resultDto;
}
@Override
public RemoveDirectoryResultDto removeDirectory(RemoveDirectoryDto dto) {
RemoveDirectoryResultDto resultDto = new RemoveDirectoryResultDto();
if (dto.getUsername() == null) {
dto.setUsername("anonymous");
}
if (dto.getPassword() == null) {
dto.setPassword("anonymous");
}
resultDto.setScmRoot(connection.getSvnRoot(dto.getUsername(), dto.getPassword()));
if (resultDto.getScmRoot() == null) {
resultDto.setScmRoot(connection.getSvnPath());
}
if (connection.getSvnPath().endsWith("/")) {
resultDto.setFileRoot(connection.getSvnPath().substring(0, connection.getSvnPath().lastIndexOf('/')));
} else {
resultDto.setFileRoot(connection.getSvnPath());
}
connection.updateAuthentication(dto.getUsername(), dto.getPassword());
try {
connection.testConnection();
} catch (SVNException e) {
if (log.isDebugEnabled()) {
log.debug("Test connection fail", e);
}
resultDto.setError(RemoveDirectoryResultDto.CONNECTION_FAILED);
return resultDto;
}
// if the name of the directory to remove is empty we get back to the remove directory form
if (dto.getDirectoryToRemove() == null) {
resultDto.setError(RemoveDirectoryResultDto.REDIRECT);
return resultDto;
}
if (dto.getDirectoryToRemove().equals("") || dto.getDirectoryToRemove().equals(resultDto.getFileRoot())) {
resultDto.setError(RemoveDirectoryResultDto.REDIRECT);
return resultDto;
}
SVNCommitClient commitClient = new SVNCommitClient(connection.getManager(), connection.getSvnOption());
try {
SVNURL[] urls = new SVNURL[1];
urls[0] = SVNURL.parseURIEncoded(dto.getDirectoryToRemove());
commitClient.doDelete(urls, "From scmwebeditor -- remove the directory : " + dto.getDirectoryToRemove());
} catch (SVNAuthenticationException authexep) {
if (log.isErrorEnabled()) {
log.error("authentication fail", authexep);
}
resultDto.setError(RemoveDirectoryResultDto.AUTH_ERROR);
return resultDto;
} catch (SVNException e) {
if (log.isErrorEnabled()) {
log.error("Error SVN import", e);
}
resultDto.setError(RemoveDirectoryResultDto.ERROR);
return resultDto;
}
return resultDto;
}
@Override
public MoveFileResultDto moveFile(MoveFileDto dto) {
MoveFileResultDto resultDto = new MoveFileResultDto();
if (dto.getUsername() == null) {
dto.setUsername("anonymous");
}
if (dto.getPassword() == null) {
dto.setPassword("anonymous");
}
resultDto.setScmRoot(connection.getSvnRoot(dto.getUsername(), dto.getPassword()));
if (resultDto.getScmRoot() == null) {
resultDto.setScmRoot(connection.getSvnPath());
}
if (connection.getSvnPath().endsWith("/")) {
resultDto.setFileRoot(connection.getSvnPath().substring(0, connection.getSvnPath().lastIndexOf('/')));
} else {
resultDto.setFileRoot(connection.getSvnPath());
}
connection.updateAuthentication(dto.getUsername(), dto.getPassword());
try {
connection.testConnection();
} catch (SVNException e) {
if (log.isDebugEnabled()) {
log.debug("Test connection fail", e);
}
resultDto.setError(MoveFileResultDto.CONNECTION_FAILED);
return resultDto;
}
// if the name of the file to move is empty we get back to the move a file form
if (dto.getFileToMove() == null) {
resultDto.setError(MoveFileResultDto.REDIRECT);
return resultDto;
}
if (dto.getFileToMove().equals("") || dto.getFileToMove().equals(resultDto.getFileRoot())) {
resultDto.setError(MoveFileResultDto.REDIRECT);
return resultDto;
}
// if the name of the destination directory is empty we get back to the move a file form
if (dto.getDestinationDirectory() == null) {
resultDto.setError(MoveFileResultDto.REDIRECT);
return resultDto;
}
if (dto.getDestinationDirectory().equals("")) {
resultDto.setError(MoveFileResultDto.REDIRECT);
return resultDto;
}
// getting thr URLs
String fileName = dto.getFileToMove().substring(dto.getFileToMove().lastIndexOf('/') + 1);
SVNURL sourceUrl;
try {
sourceUrl = SVNURL.parseURIEncoded(dto.getFileToMove());
} catch (SVNException e) {
if (log.isErrorEnabled()) {
log.error("Can not get source file URL " + dto.getFileToMove(), e);
}
return resultDto;
}
SVNURL destUrl;
try {
destUrl = SVNURL.parseURIEncoded(dto.getDestinationDirectory() + "/" + fileName);
} catch (SVNException e) {
if (log.isErrorEnabled()) {
log.error("Can not get destination file URL " + dto.getDestinationDirectory() + "/" + fileName, e);
}
return resultDto;
}
SVNCopySource[] sourceFileTab = new SVNCopySource[1];
sourceFileTab[0] = new SVNCopySource(SVNRevision.HEAD, SVNRevision.HEAD, sourceUrl);
// moving the file
SVNCopyClient copyClient = new SVNCopyClient(connection.getManager(), connection.getSvnOption());
try {
copyClient.doCopy(sourceFileTab, destUrl, true, false, true, "From scmwebeditor -- move the file : "
+ sourceUrl.getPath() + " to : " + destUrl.getPath(), null);
} catch (SVNAuthenticationException authexep) {
if (log.isErrorEnabled()) {
log.error("authentication fail", authexep);
}
resultDto.setError(MoveFileResultDto.AUTH_ERROR);
return resultDto;
} catch (SVNException e) {
if (log.isErrorEnabled()) {
log.error("Error SVN import", e);
}
resultDto.setError(MoveFileResultDto.ERROR);
return resultDto;
}
return resultDto;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy