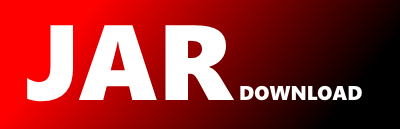
org.nuiton.scmwebeditor.svn.SvnProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scmwebeditor-svn Show documentation
Show all versions of scmwebeditor-svn Show documentation
Adds the support of SVN repositories to ScmWebEditor.
The newest version!
/*
* #%L
* ScmWebEditor
* %%
* Copyright (C) 2009 - 2015 CodeLutin
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.scmwebeditor.svn;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.nuiton.scmwebeditor.api.*;
import org.nuiton.scmwebeditor.api.dto.CreateBranchDto;
import org.tmatesoft.svn.core.SVNException;
import org.tmatesoft.svn.core.internal.io.dav.DAVRepositoryFactory;
import org.tmatesoft.svn.core.internal.io.svn.SVNRepositoryFactoryImpl;
import javax.naming.AuthenticationException;
import java.util.List;
/**
* Implementation of the SVN's specific features
*/
public class SvnProvider implements ScmProvider {
private static final Log log = LogFactory.getLog(SvnProvider.class);
/**
* Initialization of the SVN features
*/
public SvnProvider() {
SVNRepositoryFactoryImpl.setup();
DAVRepositoryFactory.setup();
}
@Override
public boolean supportsBranches() {
return false;
}
@Override
public List listBranches(String address, String username, String password) throws OperationNotSupportedException {
throw new OperationNotSupportedException("The 'list branches' operation is not available for SVN repositories");
}
@Override
public String createBranch(CreateBranchDto dto) throws OperationNotSupportedException,
AuthenticationException, RepositoryNotFoundException {
throw new OperationNotSupportedException("The 'create a branch' operation is not available for SVN repositories");
}
@Override
public String changeBranch(String branchName, String pathToLocalRepos, String username, String password)
throws OperationNotSupportedException {
throw new OperationNotSupportedException("The 'change branch' operation is not available for SVN repositories");
}
@Override
public boolean supportsPush() {
return false;
}
@Override
public boolean filesDirectlyAccessible() {
return true;
}
@Override
public ScmConnection getConnection(String address, String pathToLocalRepos) {
SvnConnection svnConn = null;
try {
svnConn = new SvnConnection(address, pathToLocalRepos);
} catch (SVNException e) {
if (log.isErrorEnabled()) {
log.error("Can not connect to SVN repository at " + address, e);
}
}
return svnConn;
}
@Override
public ScmFileManager getFileManager(ScmConnection connection) {
SvnFileManager fileManager = null;
if (connection instanceof SvnConnection) {
try {
fileManager = new SvnFileManager((SvnConnection) connection);
} catch (SVNException e) {
if (log.isErrorEnabled()) {
log.error("Can not connect to SVN repository", e);
}
}
} else {
throw new SweInternalException("Can not get SVN file manager for a non-SVN connection");
}
return fileManager;
}
@Override
public boolean addressSeemsCompatible(String address) {
if (address.startsWith("svn://") || address.contains("svn.") || address.contains("subversion.")) {
return true;
} else {
return false;
}
}
@Override
public String getDefaultBranchName() throws OperationNotSupportedException {
throw new OperationNotSupportedException("The 'get default branch name' operation is not available for " +
"SVN repositories");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy