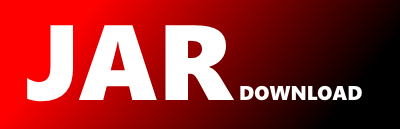
org.numenta.nupic.algorithms.AnomalyLikelihoodMetrics Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of htm.java Show documentation
Show all versions of htm.java Show documentation
The Java version of Numenta's HTM technology
/* ---------------------------------------------------------------------
* Numenta Platform for Intelligent Computing (NuPIC)
* Copyright (C) 2014, Numenta, Inc. Unless you have an agreement
* with Numenta, Inc., for a separate license for this software code, the
* following terms and conditions apply:
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License version 3 as
* published by the Free Software Foundation.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
* See the GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see http://www.gnu.org/licenses.
*
* http://numenta.org/licenses/
* ---------------------------------------------------------------------
*/
package org.numenta.nupic.algorithms;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import org.numenta.nupic.algorithms.Anomaly.AveragedAnomalyRecordList;
import org.numenta.nupic.algorithms.AnomalyLikelihood.AnomalyParams;
/**
* Container class to hold the results of {@link AnomalyLikelihood} estimations
* and updates.
*
* @author David Ray
* @see AnomalyLikelihood
* @see AnomalyLikelihoodTest
*/
public class AnomalyLikelihoodMetrics {
private AnomalyParams params;
private AveragedAnomalyRecordList aggRecordList;
private double[] likelihoods;
/**
* Constructs a new {@code AnomalyLikelihoodMetrics}
*
* @param likelihoods array of pre-computed estimations
* @param aggRecordList List of {@link Sample}s which are basically a set of date, value, average score,
* a list of historical values, and a running total.
* @param params {@link AnomalyParams} which are a {@link Statistic}, array of likelihoods,
* and a {@link MovingAverage}
*/
public AnomalyLikelihoodMetrics(double[] likelihoods, AveragedAnomalyRecordList aggRecordList, AnomalyParams params) {
this.params = params;
this.aggRecordList = aggRecordList;
this.likelihoods = likelihoods;
}
/**
* Utility method to copy this {@link AnomalyLikelihoodMetrics} object.
* @return
*/
public AnomalyLikelihoodMetrics copy() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy