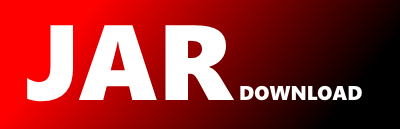
org.nutz.plugins.cache.impl.ehcache.EhcacheCache Maven / Gradle / Ivy
package org.nutz.plugins.cache.impl.ehcache;
import java.util.Collection;
import java.util.HashSet;
import java.util.Set;
import org.apache.shiro.cache.Cache;
import net.sf.ehcache.Element;
@SuppressWarnings("unchecked")
public class EhcacheCache implements Cache {
protected net.sf.ehcache.Cache cache;
public EhcacheCache(net.sf.ehcache.Cache cache) {
this.cache = cache;
}
@Override
public V get(K key) {
Element ele = cache.get(key);
if (ele == null)
return null;
return (V) ele.getObjectValue();
}
@Override
public V put(K key, V value) {
cache.put(new Element(key, value));
return null;
}
@Override
public V remove(K key) {
cache.remove(key);
return null;
}
@Override
public void clear() {
cache.removeAll();
}
@Override
public int size() {
return cache.getSize();
}
@Override
public Set keys() {
return new HashSet(cache.getKeys());
}
@Override
public Collection values() {
return (Collection) cache.getAll(cache.getKeys()).values();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy