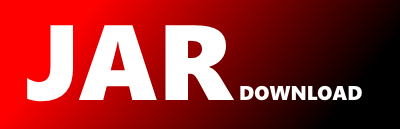
org.nutz.plugins.cache.impl.lcache.LCache Maven / Gradle / Ivy
package org.nutz.plugins.cache.impl.lcache;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import org.apache.shiro.cache.Cache;
import org.nutz.lang.Streams;
import org.nutz.log.Log;
import org.nutz.log.Logs;
import redis.clients.jedis.Jedis;
public class LCache implements Cache {
private static final Log log = Logs.get();
protected List> list = new ArrayList>();
protected String name;
public LCache(String name) {
this.name = name;
}
public void add(Cache cache) {
list.add(cache);
}
public V get(K key) {
for (Cache cache : list) {
V v = cache.get(key);
if (v != null)
return v;
}
return null;
}
@Override
public V put(K key, V value) {
for (Cache cache : list)
cache.put(key, value);
fire(genKey(key));
return null;
}
public V remove(K key) {
for (Cache cache : list)
cache.remove(key);
fire(genKey(key));
return null;
}
@Override
public void clear() {
for (Cache cache : list) {
cache.clear();
}
}
@Override
public int size() {
return keys().size();
}
@Override
public Set keys() {
Set keys = new HashSet();
for (Cache cache : list) {
keys.addAll(cache.keys());
}
return keys;
}
@Override
public Collection values() {
Set values = new HashSet();
for (Cache cache : list) {
values.addAll(cache.values());
}
return values;
}
public String genKey(K k) {
return k.toString();
}
public void fire(String key) {
Jedis jedis = null;
try {
jedis = LCacheManager.me.jedis();
String channel = (LCacheManager.PREFIX + name);
String msg = LCacheManager.me().id + ":" + key;
log.debugf("fire channel=%s msg=%s", channel, msg);
jedis.publish(channel, msg);
} finally {
Streams.safeClose(jedis);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy