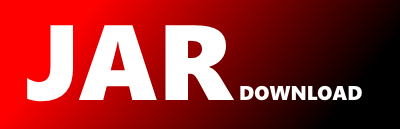
org.nutz.el.opt.arithmetic.NegativeOpt Maven / Gradle / Ivy
package org.nutz.el.opt.arithmetic;
import java.util.Queue;
import org.nutz.el.opt.AbstractOpt;
/**
* 负号:'-'
* @author juqkai([email protected])
*
*/
public class NegativeOpt extends AbstractOpt {
private Object right;
public int fetchPriority() {
return 2;
}
public void wrap(Queue
© 2015 - 2025 Weber Informatics LLC | Privacy Policy