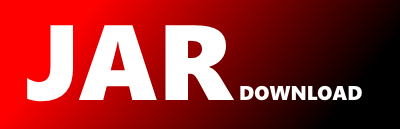
org.nutz.mapl.impl.MaplRebuild Maven / Gradle / Ivy
package org.nutz.mapl.impl;
import java.util.ArrayList;
import java.util.Collection;
import java.util.LinkedHashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import org.nutz.lang.Lang;
import org.nutz.lang.Strings;
/**
* 构建新的MapList结构对象, 根据path重建MapList结构
*
* @author juqkai([email protected])
*/
public class MaplRebuild {
enum Model {
add, del, cell
}
private Model model = Model.add;
private String[] keys;
private Object val;
// 数组索引
private Integer arrayItem;
// 数组栈列表
protected LinkedList arrayIndex = new LinkedList();
// 新MapList结构
private Map newobj = new LinkedHashMap();
private Object cellObj = null;
public MaplRebuild() {
newobj.put("obj", null);
}
public MaplRebuild(Object mapl) {
newobj.put("obj", mapl);
}
/**
* 添加属性
*
* @param path
* 路径
* @param obj
* 值
*/
public void put(String path, Object obj) {
init(path, obj);
inject(newobj, 0);
}
/**
* 添加属性
*
* @param path
* 路径
* @param obj
* 值
* @param arrayIndex
* 索引队列
*/
public void put(String path, Object obj, LinkedList arrayIndex) {
this.arrayIndex = arrayIndex;
put(path, obj);
}
/**
* 删除结点
*
* @param path
*/
public void remove(String path) {
model = Model.del;
init(path, null);
inject(newobj, 0);
}
/**
* 访问结点
*
* @param path
* 路径
*/
public Object cell(String path) {
model = Model.cell;
init(path, null);
inject(newobj, 0);
return cellObj;
}
/**
* 提取重建后的MapList
*/
public Object fetchNewobj() {
return newobj.get("obj");
}
private void init(String keys, Object obj) {
this.keys = Lang.arrayFirst("obj", Strings.split(keys, false, '.'));
this.val = obj;
this.arrayItem = 0;
}
/**
* 注入
*
* @param obj
* @param i
*/
@SuppressWarnings("unchecked")
private Object inject(Object obj, int i) {
String key = keys[i];
// 根数组
if (key.indexOf('[') == 0) {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy