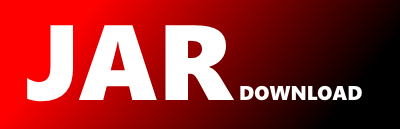
org.objectstyle.cayenne.query.DeleteQuery Maven / Gradle / Ivy
/*
* ==================================================================== The ObjectStyle
* Group Software License, version 1.1 ObjectStyle Group - http://objectstyle.org/
* Copyright (c) 2002-2005, Andrei (Andrus) Adamchik and individual authors of the
* software. All rights reserved. Redistribution and use in source and binary forms, with
* or without modification, are permitted provided that the following conditions are met:
* 1. Redistributions of source code must retain the above copyright notice, this list of
* conditions and the following disclaimer. 2. Redistributions in binary form must
* reproduce the above copyright notice, this list of conditions and the following
* disclaimer in the documentation and/or other materials provided with the distribution.
* 3. The end-user documentation included with the redistribution, if any, must include
* the following acknowlegement: "This product includes software developed by independent
* contributors and hosted on ObjectStyle Group web site (http://objectstyle.org/)."
* Alternately, this acknowlegement may appear in the software itself, if and wherever
* such third-party acknowlegements normally appear. 4. The names "ObjectStyle Group" and
* "Cayenne" must not be used to endorse or promote products derived from this software
* without prior written permission. For written permission, email "andrus at objectstyle
* dot org". 5. Products derived from this software may not be called "ObjectStyle" or
* "Cayenne", nor may "ObjectStyle" or "Cayenne" appear in their names without prior
* written permission. THIS SOFTWARE IS PROVIDED ``AS IS'' AND ANY EXPRESSED OR IMPLIED
* WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY
* AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE OBJECTSTYLE
* GROUP OR ITS CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL,
* EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION)
* HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
* OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
* SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
* ==================================================================== This software
* consists of voluntary contributions made by many individuals and hosted on ObjectStyle
* Group web site. For more information on the ObjectStyle Group, please see
* .
*/
package org.objectstyle.cayenne.query;
import org.objectstyle.cayenne.exp.Expression;
import org.objectstyle.cayenne.map.ObjEntity;
public class DeleteQuery extends QualifiedQuery {
/** Creates empty DeleteQuery. */
public DeleteQuery() {
super();
}
private void init(Object root, Expression qualifier) {
this.setRoot(root);
this.setQualifier(qualifier);
}
/**
* Creates a DeleteQuery with null qualifier, for the specifed ObjEntity
*
* @param root the ObjEntity this DeleteQuery is for.
*/
public DeleteQuery(ObjEntity root) {
this(root, null);
}
/**
* Creates a DeleteQuery for the specifed ObjEntity with the given qualifier
*
* @param root the ObjEntity this DeleteQuery is for.
* @param qualifier an Expression indicating which objects should be deleted
*/
public DeleteQuery(ObjEntity root, Expression qualifier) {
this();
this.init(root, qualifier);
}
/**
* Creates a DeleteQuery with null qualifier, for the entity which uses the given
* class
*
* @param rootClass the Class of objects this DeleteQuery is for.
*/
public DeleteQuery(Class rootClass) {
this(rootClass, null);
}
/**
* Creates a DeleteQuery for the entity which uses the given class, with the given
* qualifier
*
* @param rootClass the Class of objects this DeleteQuery is for.
* @param qualifier an Expression indicating which objects should be deleted
*/
public DeleteQuery(Class rootClass, Expression qualifier) {
this.init(rootClass, qualifier);
}
/** Creates DeleteQuery with objEntityName
parameter. */
public DeleteQuery(String objEntityName) {
this(objEntityName, null);
}
/**
* Creates DeleteQuery with objEntityName
and qualifier
* parameters.
*/
public DeleteQuery(String objEntityName, Expression qualifier) {
this.init(objEntityName, qualifier);
}
/**
* Calls "makeUpdate" on the visitor.
*
* @since 1.2
*/
public SQLAction createSQLAction(SQLActionVisitor visitor) {
return visitor.updateAction(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy