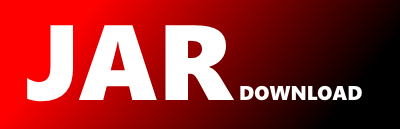
org.objectweb.celtix.bus.configuration.security.SSLServerPolicy Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.0-hudson-3037-ea3
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2006.05.08 at 07:49:35 AM EDT
//
package org.objectweb.celtix.bus.configuration.security;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.AccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
import org.objectweb.celtix.bus.configuration.security.SSLServerPolicy;
/**
*
* New policies for controlling SSL encryption
*
*
* Java class for SSLServerPolicy complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="SSLServerPolicy">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Keystore" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="KeystoreType" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="KeystorePassword" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="KeyPassword" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="WantClientAuthentication" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="RequireClientAuthentication" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="KeystoreAlgorithm" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="Ciphersuites" type="{http://www.w3.org/2001/XMLSchema}string" maxOccurs="unbounded" minOccurs="0"/>
* <element name="TrustStore" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="TrustStoreType" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="TrustStoreAlgorithm" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="SecureSocketProtocol" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="SessionCaching" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="SessionCacheKey" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="MaxChainLength" type="{http://www.w3.org/2001/XMLSchema}long" minOccurs="0"/>
* <element name="CertValidator" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(AccessType.FIELD)
@XmlType(name = "SSLServerPolicy", propOrder = {
"keystore",
"keystoreType",
"keystorePassword",
"keyPassword",
"wantClientAuthentication",
"requireClientAuthentication",
"keystoreAlgorithm",
"ciphersuites",
"trustStore",
"trustStoreType",
"trustStoreAlgorithm",
"secureSocketProtocol",
"sessionCaching",
"sessionCacheKey",
"maxChainLength",
"certValidator"
})
public class SSLServerPolicy {
@XmlElement(name = "Keystore", namespace = "http://celtix.objectweb.org/bus/configuration/security")
protected String keystore;
@XmlElement(name = "KeystoreType", namespace = "http://celtix.objectweb.org/bus/configuration/security")
protected String keystoreType;
@XmlElement(name = "KeystorePassword", namespace = "http://celtix.objectweb.org/bus/configuration/security")
protected String keystorePassword;
@XmlElement(name = "KeyPassword", namespace = "http://celtix.objectweb.org/bus/configuration/security")
protected String keyPassword;
@XmlElement(name = "WantClientAuthentication", namespace = "http://celtix.objectweb.org/bus/configuration/security")
protected Boolean wantClientAuthentication;
@XmlElement(name = "RequireClientAuthentication", namespace = "http://celtix.objectweb.org/bus/configuration/security")
protected Boolean requireClientAuthentication;
@XmlElement(name = "KeystoreAlgorithm", namespace = "http://celtix.objectweb.org/bus/configuration/security")
protected String keystoreAlgorithm;
@XmlElement(name = "Ciphersuites", namespace = "http://celtix.objectweb.org/bus/configuration/security")
protected List ciphersuites;
@XmlElement(name = "TrustStore", namespace = "http://celtix.objectweb.org/bus/configuration/security")
protected String trustStore;
@XmlElement(name = "TrustStoreType", namespace = "http://celtix.objectweb.org/bus/configuration/security")
protected String trustStoreType;
@XmlElement(name = "TrustStoreAlgorithm", namespace = "http://celtix.objectweb.org/bus/configuration/security")
protected String trustStoreAlgorithm;
@XmlElement(name = "SecureSocketProtocol", namespace = "http://celtix.objectweb.org/bus/configuration/security")
protected String secureSocketProtocol;
@XmlElement(name = "SessionCaching", namespace = "http://celtix.objectweb.org/bus/configuration/security")
protected Boolean sessionCaching;
@XmlElement(name = "SessionCacheKey", namespace = "http://celtix.objectweb.org/bus/configuration/security")
protected String sessionCacheKey;
@XmlElement(name = "MaxChainLength", namespace = "http://celtix.objectweb.org/bus/configuration/security")
protected Long maxChainLength;
@XmlElement(name = "CertValidator", namespace = "http://celtix.objectweb.org/bus/configuration/security")
protected String certValidator;
/**
* Gets the value of the keystore property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getKeystore() {
return keystore;
}
/**
* Sets the value of the keystore property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKeystore(String value) {
this.keystore = value;
}
public boolean isSetKeystore() {
return (this.keystore!= null);
}
/**
* Gets the value of the keystoreType property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getKeystoreType() {
return keystoreType;
}
/**
* Sets the value of the keystoreType property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKeystoreType(String value) {
this.keystoreType = value;
}
public boolean isSetKeystoreType() {
return (this.keystoreType!= null);
}
/**
* Gets the value of the keystorePassword property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getKeystorePassword() {
return keystorePassword;
}
/**
* Sets the value of the keystorePassword property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKeystorePassword(String value) {
this.keystorePassword = value;
}
public boolean isSetKeystorePassword() {
return (this.keystorePassword!= null);
}
/**
* Gets the value of the keyPassword property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getKeyPassword() {
return keyPassword;
}
/**
* Sets the value of the keyPassword property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKeyPassword(String value) {
this.keyPassword = value;
}
public boolean isSetKeyPassword() {
return (this.keyPassword!= null);
}
/**
* Gets the value of the wantClientAuthentication property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isWantClientAuthentication() {
return wantClientAuthentication;
}
/**
* Sets the value of the wantClientAuthentication property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setWantClientAuthentication(Boolean value) {
this.wantClientAuthentication = value;
}
public boolean isSetWantClientAuthentication() {
return (this.wantClientAuthentication!= null);
}
/**
* Gets the value of the requireClientAuthentication property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isRequireClientAuthentication() {
return requireClientAuthentication;
}
/**
* Sets the value of the requireClientAuthentication property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setRequireClientAuthentication(Boolean value) {
this.requireClientAuthentication = value;
}
public boolean isSetRequireClientAuthentication() {
return (this.requireClientAuthentication!= null);
}
/**
* Gets the value of the keystoreAlgorithm property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getKeystoreAlgorithm() {
return keystoreAlgorithm;
}
/**
* Sets the value of the keystoreAlgorithm property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKeystoreAlgorithm(String value) {
this.keystoreAlgorithm = value;
}
public boolean isSetKeystoreAlgorithm() {
return (this.keystoreAlgorithm!= null);
}
/**
* Gets the value of the ciphersuites property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the ciphersuites property.
*
*
* For example, to add a new item, do as follows:
*
* getCiphersuites().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getCiphersuites() {
if (ciphersuites == null) {
ciphersuites = new ArrayList();
}
return this.ciphersuites;
}
public boolean isSetCiphersuites() {
return ((this.ciphersuites!= null)&&(!this.ciphersuites.isEmpty()));
}
public void unsetCiphersuites() {
this.ciphersuites = null;
}
/**
* Gets the value of the trustStore property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTrustStore() {
return trustStore;
}
/**
* Sets the value of the trustStore property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTrustStore(String value) {
this.trustStore = value;
}
public boolean isSetTrustStore() {
return (this.trustStore!= null);
}
/**
* Gets the value of the trustStoreType property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTrustStoreType() {
return trustStoreType;
}
/**
* Sets the value of the trustStoreType property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTrustStoreType(String value) {
this.trustStoreType = value;
}
public boolean isSetTrustStoreType() {
return (this.trustStoreType!= null);
}
/**
* Gets the value of the trustStoreAlgorithm property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTrustStoreAlgorithm() {
return trustStoreAlgorithm;
}
/**
* Sets the value of the trustStoreAlgorithm property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTrustStoreAlgorithm(String value) {
this.trustStoreAlgorithm = value;
}
public boolean isSetTrustStoreAlgorithm() {
return (this.trustStoreAlgorithm!= null);
}
/**
* Gets the value of the secureSocketProtocol property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSecureSocketProtocol() {
return secureSocketProtocol;
}
/**
* Sets the value of the secureSocketProtocol property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSecureSocketProtocol(String value) {
this.secureSocketProtocol = value;
}
public boolean isSetSecureSocketProtocol() {
return (this.secureSocketProtocol!= null);
}
/**
* Gets the value of the sessionCaching property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isSessionCaching() {
return sessionCaching;
}
/**
* Sets the value of the sessionCaching property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setSessionCaching(Boolean value) {
this.sessionCaching = value;
}
public boolean isSetSessionCaching() {
return (this.sessionCaching!= null);
}
/**
* Gets the value of the sessionCacheKey property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSessionCacheKey() {
return sessionCacheKey;
}
/**
* Sets the value of the sessionCacheKey property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSessionCacheKey(String value) {
this.sessionCacheKey = value;
}
public boolean isSetSessionCacheKey() {
return (this.sessionCacheKey!= null);
}
/**
* Gets the value of the maxChainLength property.
*
* @return
* possible object is
* {@link Long }
*
*/
public Long getMaxChainLength() {
return maxChainLength;
}
/**
* Sets the value of the maxChainLength property.
*
* @param value
* allowed object is
* {@link Long }
*
*/
public void setMaxChainLength(Long value) {
this.maxChainLength = value;
}
public boolean isSetMaxChainLength() {
return (this.maxChainLength!= null);
}
/**
* Gets the value of the certValidator property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCertValidator() {
return certValidator;
}
/**
* Sets the value of the certValidator property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCertValidator(String value) {
this.certValidator = value;
}
public boolean isSetCertValidator() {
return (this.certValidator!= null);
}
}