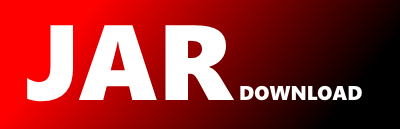
org.ow2.binjiu.description.upnp2model.UPnPParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of upnp2java Show documentation
Show all versions of upnp2java Show documentation
Description generator for convert UPnP XML description to java interfaces.
package org.ow2.binjiu.description.upnp2model;
import java.io.File;
import org.cybergarage.upnp.Device;
import org.cybergarage.upnp.device.InvalidDescriptionException;
import org.ow2.binjiu.description.exception.UnpleasantTypeException;
import org.ow2.binjiu.description.model.Action;
import org.ow2.binjiu.description.model.Component;
import org.ow2.binjiu.description.model.Service;
import org.ow2.binjiu.description.model.Type;
import org.ow2.binjiu.description.model.Variable;
import org.ow2.binjiu.description.resource.Properties;
public class UPnPParser {
private static final String IN = "IN";
private static final String OUT = "OUT";
private Device device;
public UPnPParser(File descriptionFile) throws InvalidDescriptionException {
this.device = new Device(descriptionFile);
}
public Component parse() throws UnpleasantTypeException {
Component component = new Component(device.getFriendlyName());
for (Object upnpService : device.getServiceList()) {
component.addService(parseService((org.cybergarage.upnp.Service) upnpService));
}
return component;
}
private Service parseService(org.cybergarage.upnp.Service upnpService)
throws UnpleasantTypeException {
String serviceType = upnpService.getServiceType();
Service service = new Service(getServiceName(serviceType));
for (Object upnpAction : upnpService.getActionList()) {
service.addAction(parseAction((org.cybergarage.upnp.Action) upnpAction));
}
return service;
}
private Action parseAction(org.cybergarage.upnp.Action upnpAction)
throws UnpleasantTypeException {
Action action = new Action(upnpAction.getName());
for (Object argument : upnpAction.getArgumentList()) {
org.cybergarage.upnp.Argument upnpArgument = (org.cybergarage.upnp.Argument) argument;
// find corresponding datatype
String upnpDatatype = upnpArgument.getRelatedStateVariable()
.getDataType();
Type modelType = U2MProcessor.getAssociatedType(upnpDatatype);
// get direction
String direction = upnpArgument.getDirection();
// we create the variable
Variable variable = new Variable(upnpArgument.getName(), modelType);
// add it to action definition
if (direction.toUpperCase().equals(IN)) {
action.addArgument(variable);
} else if (direction.toUpperCase().equals(OUT)) {
action.addReturn(variable);
}
// --> we believe in Cyberlink so if direction isn't one of them, an
// InvalidDescriptionException should have been threw before
}
return action;
}
private String getServiceName(String serviceType){
//String servicePrefix = Properties.schemas + Properties.serviceType;
String firstCut = serviceType.substring(Properties.serviceNamePrefix.length());
return firstCut.substring(0, firstCut.length()-2);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy