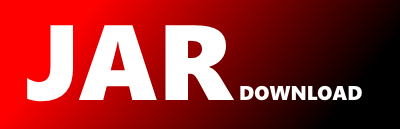
org.xmlnetwork.Group Maven / Gradle / Ivy
Show all versions of fractal-bf-hdl-model Show documentation
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.0.2-b01-fcs
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2008.06.03 at 04:27:27 PM CEST
//
package org.xmlnetwork;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for group complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="group">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Description" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="Local-AS" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attribute name="name" type="{http://www.xmlnetwork.org}asNumInt" />
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="Update-Source" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attribute name="iface" type="{http://www.w3.org/2001/XMLSchema}string" />
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="Peer-AS" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attribute name="name" type="{http://www.xmlnetwork.org}asNumInt" />
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="Default-Originate" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="Multihop" type="{http://www.xmlnetwork.org}ttl" minOccurs="0"/>
* <element name="Prefix-Limit" type="{http://www.xmlnetwork.org}prefixLimit" minOccurs="0"/>
* <element name="Next-Hop-Self" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="Authentication" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attribute name="password" type="{http://www.w3.org/2001/XMLSchema}string" />
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="Remove-Private-AS" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="Timers" type="{http://www.xmlnetwork.org}bgpTimers" minOccurs="0"/>
* <element name="Policy" type="{http://www.xmlnetwork.org}policyCall" maxOccurs="unbounded" minOccurs="0"/>
* <element name="List" type="{http://www.xmlnetwork.org}listCall" maxOccurs="unbounded" minOccurs="0"/>
* <element name="Neighbor" type="{http://www.xmlnetwork.org}neighbor" maxOccurs="unbounded"/>
* </sequence>
* <attribute name="name" type="{http://www.w3.org/2001/XMLSchema}string" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "group", propOrder = {
"description",
"localAS",
"updateSource",
"peerAS",
"defaultOriginate",
"multihop",
"prefixLimit",
"nextHopSelf",
"authentication",
"removePrivateAS",
"timers",
"policy",
"list",
"neighbor"
})
public class Group {
@XmlElement(name = "Description")
protected String description;
@XmlElement(name = "Local-AS")
protected Group.LocalAS localAS;
@XmlElement(name = "Update-Source")
protected Group.UpdateSource updateSource;
@XmlElement(name = "Peer-AS")
protected Group.PeerAS peerAS;
@XmlElement(name = "Default-Originate")
protected Group.DefaultOriginate defaultOriginate;
@XmlElement(name = "Multihop")
protected Integer multihop;
@XmlElement(name = "Prefix-Limit")
protected PrefixLimit prefixLimit;
@XmlElement(name = "Next-Hop-Self")
protected Group.NextHopSelf nextHopSelf;
@XmlElement(name = "Authentication")
protected Group.Authentication authentication;
@XmlElement(name = "Remove-Private-AS")
protected Group.RemovePrivateAS removePrivateAS;
@XmlElement(name = "Timers")
protected BgpTimers timers;
@XmlElement(name = "Policy")
protected List policy;
@XmlElement(name = "List")
protected List list;
@XmlElement(name = "Neighbor", required = true)
protected List neighbor;
@XmlAttribute
protected String name;
/**
* Gets the value of the description property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDescription() {
return description;
}
/**
* Sets the value of the description property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDescription(String value) {
this.description = value;
}
/**
* Gets the value of the localAS property.
*
* @return
* possible object is
* {@link Group.LocalAS }
*
*/
public Group.LocalAS getLocalAS() {
return localAS;
}
/**
* Sets the value of the localAS property.
*
* @param value
* allowed object is
* {@link Group.LocalAS }
*
*/
public void setLocalAS(Group.LocalAS value) {
this.localAS = value;
}
/**
* Gets the value of the updateSource property.
*
* @return
* possible object is
* {@link Group.UpdateSource }
*
*/
public Group.UpdateSource getUpdateSource() {
return updateSource;
}
/**
* Sets the value of the updateSource property.
*
* @param value
* allowed object is
* {@link Group.UpdateSource }
*
*/
public void setUpdateSource(Group.UpdateSource value) {
this.updateSource = value;
}
/**
* Gets the value of the peerAS property.
*
* @return
* possible object is
* {@link Group.PeerAS }
*
*/
public Group.PeerAS getPeerAS() {
return peerAS;
}
/**
* Sets the value of the peerAS property.
*
* @param value
* allowed object is
* {@link Group.PeerAS }
*
*/
public void setPeerAS(Group.PeerAS value) {
this.peerAS = value;
}
/**
* Gets the value of the defaultOriginate property.
*
* @return
* possible object is
* {@link Group.DefaultOriginate }
*
*/
public Group.DefaultOriginate getDefaultOriginate() {
return defaultOriginate;
}
/**
* Sets the value of the defaultOriginate property.
*
* @param value
* allowed object is
* {@link Group.DefaultOriginate }
*
*/
public void setDefaultOriginate(Group.DefaultOriginate value) {
this.defaultOriginate = value;
}
/**
* Gets the value of the multihop property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getMultihop() {
return multihop;
}
/**
* Sets the value of the multihop property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setMultihop(Integer value) {
this.multihop = value;
}
/**
* Gets the value of the prefixLimit property.
*
* @return
* possible object is
* {@link PrefixLimit }
*
*/
public PrefixLimit getPrefixLimit() {
return prefixLimit;
}
/**
* Sets the value of the prefixLimit property.
*
* @param value
* allowed object is
* {@link PrefixLimit }
*
*/
public void setPrefixLimit(PrefixLimit value) {
this.prefixLimit = value;
}
/**
* Gets the value of the nextHopSelf property.
*
* @return
* possible object is
* {@link Group.NextHopSelf }
*
*/
public Group.NextHopSelf getNextHopSelf() {
return nextHopSelf;
}
/**
* Sets the value of the nextHopSelf property.
*
* @param value
* allowed object is
* {@link Group.NextHopSelf }
*
*/
public void setNextHopSelf(Group.NextHopSelf value) {
this.nextHopSelf = value;
}
/**
* Gets the value of the authentication property.
*
* @return
* possible object is
* {@link Group.Authentication }
*
*/
public Group.Authentication getAuthentication() {
return authentication;
}
/**
* Sets the value of the authentication property.
*
* @param value
* allowed object is
* {@link Group.Authentication }
*
*/
public void setAuthentication(Group.Authentication value) {
this.authentication = value;
}
/**
* Gets the value of the removePrivateAS property.
*
* @return
* possible object is
* {@link Group.RemovePrivateAS }
*
*/
public Group.RemovePrivateAS getRemovePrivateAS() {
return removePrivateAS;
}
/**
* Sets the value of the removePrivateAS property.
*
* @param value
* allowed object is
* {@link Group.RemovePrivateAS }
*
*/
public void setRemovePrivateAS(Group.RemovePrivateAS value) {
this.removePrivateAS = value;
}
/**
* Gets the value of the timers property.
*
* @return
* possible object is
* {@link BgpTimers }
*
*/
public BgpTimers getTimers() {
return timers;
}
/**
* Sets the value of the timers property.
*
* @param value
* allowed object is
* {@link BgpTimers }
*
*/
public void setTimers(BgpTimers value) {
this.timers = value;
}
/**
* Gets the value of the policy property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the policy property.
*
*
* For example, to add a new item, do as follows:
*
* getPolicy().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PolicyCall }
*
*
*/
public List getPolicy() {
if (policy == null) {
policy = new ArrayList();
}
return this.policy;
}
/**
* Gets the value of the list property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the list property.
*
*
* For example, to add a new item, do as follows:
*
* getList().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ListCall }
*
*
*/
public List getList() {
if (list == null) {
list = new ArrayList();
}
return this.list;
}
/**
* Gets the value of the neighbor property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the neighbor property.
*
*
* For example, to add a new item, do as follows:
*
* getNeighbor().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Neighbor }
*
*
*/
public List getNeighbor() {
if (neighbor == null) {
neighbor = new ArrayList();
}
return this.neighbor;
}
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(String value) {
this.name = value;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attribute name="password" type="{http://www.w3.org/2001/XMLSchema}string" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "")
public static class Authentication {
@XmlAttribute
protected String password;
/**
* Gets the value of the password property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPassword() {
return password;
}
/**
* Sets the value of the password property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPassword(String value) {
this.password = value;
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "")
public static class DefaultOriginate {
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attribute name="name" type="{http://www.xmlnetwork.org}asNumInt" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "")
public static class LocalAS {
@XmlAttribute
protected Integer name;
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setName(Integer value) {
this.name = value;
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "")
public static class NextHopSelf {
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attribute name="name" type="{http://www.xmlnetwork.org}asNumInt" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "")
public static class PeerAS {
@XmlAttribute
protected Integer name;
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setName(Integer value) {
this.name = value;
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "")
public static class RemovePrivateAS {
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attribute name="iface" type="{http://www.w3.org/2001/XMLSchema}string" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "")
public static class UpdateSource {
@XmlAttribute
protected String iface;
/**
* Gets the value of the iface property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getIface() {
return iface;
}
/**
* Sets the value of the iface property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setIface(String value) {
this.iface = value;
}
}
}