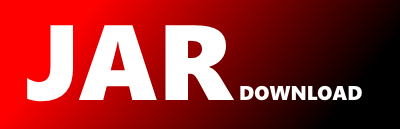
org.xmlnetwork.List Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.0.2-b01-fcs
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2008.06.03 at 04:27:27 PM CEST
//
package org.xmlnetwork;
import java.math.BigInteger;
import java.util.ArrayList;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="id" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <choice>
* <element name="PrefixList">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="prefix" type="{http://www.xmlnetwork.org}ipPrefix" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="RouteList">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="routeFilter" maxOccurs="unbounded">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="prefix" type="{http://www.xmlnetwork.org}ipPrefix"/>
* <element name="matchType">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <choice>
* <element name="OrShorter" type="{http://www.w3.org/2001/XMLSchema}anyType"/>
* <element name="Shorter" type="{http://www.w3.org/2001/XMLSchema}anyType"/>
* <element name="Exact" type="{http://www.w3.org/2001/XMLSchema}anyType"/>
* <element name="Longer" type="{http://www.w3.org/2001/XMLSchema}anyType"/>
* <element name="OrLonger" type="{http://www.w3.org/2001/XMLSchema}anyType"/>
* <element name="PrefixLengthRange">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attribute name="lower_bound" type="{http://www.w3.org/2001/XMLSchema}integer" />
* <attribute name="upper_bound" type="{http://www.w3.org/2001/XMLSchema}integer" />
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="Through" type="{http://www.xmlnetwork.org}ipPrefix"/>
* <element name="Upto">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="prefixLength" type="{http://www.xmlnetwork.org}prefixLength"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* </choice>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="target">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <enumeration value="deny"/>
* <enumeration value="permit"/>
* </restriction>
* </simpleType>
* </element>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="CommunityList">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Community" maxOccurs="unbounded">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attribute name="CommunityValue" type="{http://www.w3.org/2001/XMLSchema}integer" />
* <attribute name="asNumber" type="{http://www.w3.org/2001/XMLSchema}integer" />
* </restriction>
* </complexContent>
* </complexType>
* </element>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="ASPathList">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="RegExpr" type="{http://www.w3.org/2001/XMLSchema}string" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* </choice>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"id",
"prefixList",
"routeList",
"communityList",
"asPathList"
})
@XmlRootElement(name = "List")
public class List {
@XmlElement(required = true)
protected String id;
@XmlElement(name = "PrefixList")
protected List.PrefixList prefixList;
@XmlElement(name = "RouteList")
protected List.RouteList routeList;
@XmlElement(name = "CommunityList")
protected List.CommunityList communityList;
@XmlElement(name = "ASPathList")
protected List.ASPathList asPathList;
/**
* Gets the value of the id property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getId() {
return id;
}
/**
* Sets the value of the id property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setId(String value) {
this.id = value;
}
/**
* Gets the value of the prefixList property.
*
* @return
* possible object is
* {@link List.PrefixList }
*
*/
public List.PrefixList getPrefixList() {
return prefixList;
}
/**
* Sets the value of the prefixList property.
*
* @param value
* allowed object is
* {@link List.PrefixList }
*
*/
public void setPrefixList(List.PrefixList value) {
this.prefixList = value;
}
/**
* Gets the value of the routeList property.
*
* @return
* possible object is
* {@link List.RouteList }
*
*/
public List.RouteList getRouteList() {
return routeList;
}
/**
* Sets the value of the routeList property.
*
* @param value
* allowed object is
* {@link List.RouteList }
*
*/
public void setRouteList(List.RouteList value) {
this.routeList = value;
}
/**
* Gets the value of the communityList property.
*
* @return
* possible object is
* {@link List.CommunityList }
*
*/
public List.CommunityList getCommunityList() {
return communityList;
}
/**
* Sets the value of the communityList property.
*
* @param value
* allowed object is
* {@link List.CommunityList }
*
*/
public void setCommunityList(List.CommunityList value) {
this.communityList = value;
}
/**
* Gets the value of the asPathList property.
*
* @return
* possible object is
* {@link List.ASPathList }
*
*/
public List.ASPathList getASPathList() {
return asPathList;
}
/**
* Sets the value of the asPathList property.
*
* @param value
* allowed object is
* {@link List.ASPathList }
*
*/
public void setASPathList(List.ASPathList value) {
this.asPathList = value;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="RegExpr" type="{http://www.w3.org/2001/XMLSchema}string" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"regExpr"
})
public static class ASPathList {
@XmlElement(name = "RegExpr", required = true)
protected java.util.List regExpr;
/**
* Gets the value of the regExpr property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the regExpr property.
*
*
* For example, to add a new item, do as follows:
*
* getRegExpr().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public java.util.List getRegExpr() {
if (regExpr == null) {
regExpr = new ArrayList();
}
return this.regExpr;
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Community" maxOccurs="unbounded">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attribute name="CommunityValue" type="{http://www.w3.org/2001/XMLSchema}integer" />
* <attribute name="asNumber" type="{http://www.w3.org/2001/XMLSchema}integer" />
* </restriction>
* </complexContent>
* </complexType>
* </element>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"community"
})
public static class CommunityList {
@XmlElement(name = "Community", required = true)
protected java.util.List community;
/**
* Gets the value of the community property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the community property.
*
*
* For example, to add a new item, do as follows:
*
* getCommunity().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link List.CommunityList.Community }
*
*
*/
public java.util.List getCommunity() {
if (community == null) {
community = new ArrayList();
}
return this.community;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attribute name="CommunityValue" type="{http://www.w3.org/2001/XMLSchema}integer" />
* <attribute name="asNumber" type="{http://www.w3.org/2001/XMLSchema}integer" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "")
public static class Community {
@XmlAttribute(name = "CommunityValue")
protected BigInteger communityValue;
@XmlAttribute
protected BigInteger asNumber;
/**
* Gets the value of the communityValue property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getCommunityValue() {
return communityValue;
}
/**
* Sets the value of the communityValue property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setCommunityValue(BigInteger value) {
this.communityValue = value;
}
/**
* Gets the value of the asNumber property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getAsNumber() {
return asNumber;
}
/**
* Sets the value of the asNumber property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setAsNumber(BigInteger value) {
this.asNumber = value;
}
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="prefix" type="{http://www.xmlnetwork.org}ipPrefix" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"prefix"
})
public static class PrefixList {
@XmlElement(required = true)
protected java.util.List prefix;
/**
* Gets the value of the prefix property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the prefix property.
*
*
* For example, to add a new item, do as follows:
*
* getPrefix().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public java.util.List getPrefix() {
if (prefix == null) {
prefix = new ArrayList();
}
return this.prefix;
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="routeFilter" maxOccurs="unbounded">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="prefix" type="{http://www.xmlnetwork.org}ipPrefix"/>
* <element name="matchType">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <choice>
* <element name="OrShorter" type="{http://www.w3.org/2001/XMLSchema}anyType"/>
* <element name="Shorter" type="{http://www.w3.org/2001/XMLSchema}anyType"/>
* <element name="Exact" type="{http://www.w3.org/2001/XMLSchema}anyType"/>
* <element name="Longer" type="{http://www.w3.org/2001/XMLSchema}anyType"/>
* <element name="OrLonger" type="{http://www.w3.org/2001/XMLSchema}anyType"/>
* <element name="PrefixLengthRange">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attribute name="lower_bound" type="{http://www.w3.org/2001/XMLSchema}integer" />
* <attribute name="upper_bound" type="{http://www.w3.org/2001/XMLSchema}integer" />
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="Through" type="{http://www.xmlnetwork.org}ipPrefix"/>
* <element name="Upto">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="prefixLength" type="{http://www.xmlnetwork.org}prefixLength"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* </choice>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="target">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <enumeration value="deny"/>
* <enumeration value="permit"/>
* </restriction>
* </simpleType>
* </element>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"routeFilter"
})
public static class RouteList {
@XmlElement(required = true)
protected java.util.List routeFilter;
/**
* Gets the value of the routeFilter property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the routeFilter property.
*
*
* For example, to add a new item, do as follows:
*
* getRouteFilter().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link List.RouteList.RouteFilter }
*
*
*/
public java.util.List getRouteFilter() {
if (routeFilter == null) {
routeFilter = new ArrayList();
}
return this.routeFilter;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="prefix" type="{http://www.xmlnetwork.org}ipPrefix"/>
* <element name="matchType">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <choice>
* <element name="OrShorter" type="{http://www.w3.org/2001/XMLSchema}anyType"/>
* <element name="Shorter" type="{http://www.w3.org/2001/XMLSchema}anyType"/>
* <element name="Exact" type="{http://www.w3.org/2001/XMLSchema}anyType"/>
* <element name="Longer" type="{http://www.w3.org/2001/XMLSchema}anyType"/>
* <element name="OrLonger" type="{http://www.w3.org/2001/XMLSchema}anyType"/>
* <element name="PrefixLengthRange">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attribute name="lower_bound" type="{http://www.w3.org/2001/XMLSchema}integer" />
* <attribute name="upper_bound" type="{http://www.w3.org/2001/XMLSchema}integer" />
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="Through" type="{http://www.xmlnetwork.org}ipPrefix"/>
* <element name="Upto">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="prefixLength" type="{http://www.xmlnetwork.org}prefixLength"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* </choice>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="target">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <enumeration value="deny"/>
* <enumeration value="permit"/>
* </restriction>
* </simpleType>
* </element>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"prefix",
"matchType",
"target"
})
public static class RouteFilter {
@XmlElement(required = true)
protected String prefix;
@XmlElement(required = true)
protected List.RouteList.RouteFilter.MatchType matchType;
@XmlElement(required = true)
protected String target;
/**
* Gets the value of the prefix property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPrefix() {
return prefix;
}
/**
* Sets the value of the prefix property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPrefix(String value) {
this.prefix = value;
}
/**
* Gets the value of the matchType property.
*
* @return
* possible object is
* {@link List.RouteList.RouteFilter.MatchType }
*
*/
public List.RouteList.RouteFilter.MatchType getMatchType() {
return matchType;
}
/**
* Sets the value of the matchType property.
*
* @param value
* allowed object is
* {@link List.RouteList.RouteFilter.MatchType }
*
*/
public void setMatchType(List.RouteList.RouteFilter.MatchType value) {
this.matchType = value;
}
/**
* Gets the value of the target property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTarget() {
return target;
}
/**
* Sets the value of the target property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTarget(String value) {
this.target = value;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <choice>
* <element name="OrShorter" type="{http://www.w3.org/2001/XMLSchema}anyType"/>
* <element name="Shorter" type="{http://www.w3.org/2001/XMLSchema}anyType"/>
* <element name="Exact" type="{http://www.w3.org/2001/XMLSchema}anyType"/>
* <element name="Longer" type="{http://www.w3.org/2001/XMLSchema}anyType"/>
* <element name="OrLonger" type="{http://www.w3.org/2001/XMLSchema}anyType"/>
* <element name="PrefixLengthRange">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attribute name="lower_bound" type="{http://www.w3.org/2001/XMLSchema}integer" />
* <attribute name="upper_bound" type="{http://www.w3.org/2001/XMLSchema}integer" />
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="Through" type="{http://www.xmlnetwork.org}ipPrefix"/>
* <element name="Upto">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="prefixLength" type="{http://www.xmlnetwork.org}prefixLength"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* </choice>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"orShorter",
"shorter",
"exact",
"longer",
"orLonger",
"prefixLengthRange",
"through",
"upto"
})
public static class MatchType {
@XmlElement(name = "OrShorter")
protected Object orShorter;
@XmlElement(name = "Shorter")
protected Object shorter;
@XmlElement(name = "Exact")
protected Object exact;
@XmlElement(name = "Longer")
protected Object longer;
@XmlElement(name = "OrLonger")
protected Object orLonger;
@XmlElement(name = "PrefixLengthRange")
protected List.RouteList.RouteFilter.MatchType.PrefixLengthRange prefixLengthRange;
@XmlElement(name = "Through")
protected String through;
@XmlElement(name = "Upto")
protected List.RouteList.RouteFilter.MatchType.Upto upto;
/**
* Gets the value of the orShorter property.
*
* @return
* possible object is
* {@link Object }
*
*/
public Object getOrShorter() {
return orShorter;
}
/**
* Sets the value of the orShorter property.
*
* @param value
* allowed object is
* {@link Object }
*
*/
public void setOrShorter(Object value) {
this.orShorter = value;
}
/**
* Gets the value of the shorter property.
*
* @return
* possible object is
* {@link Object }
*
*/
public Object getShorter() {
return shorter;
}
/**
* Sets the value of the shorter property.
*
* @param value
* allowed object is
* {@link Object }
*
*/
public void setShorter(Object value) {
this.shorter = value;
}
/**
* Gets the value of the exact property.
*
* @return
* possible object is
* {@link Object }
*
*/
public Object getExact() {
return exact;
}
/**
* Sets the value of the exact property.
*
* @param value
* allowed object is
* {@link Object }
*
*/
public void setExact(Object value) {
this.exact = value;
}
/**
* Gets the value of the longer property.
*
* @return
* possible object is
* {@link Object }
*
*/
public Object getLonger() {
return longer;
}
/**
* Sets the value of the longer property.
*
* @param value
* allowed object is
* {@link Object }
*
*/
public void setLonger(Object value) {
this.longer = value;
}
/**
* Gets the value of the orLonger property.
*
* @return
* possible object is
* {@link Object }
*
*/
public Object getOrLonger() {
return orLonger;
}
/**
* Sets the value of the orLonger property.
*
* @param value
* allowed object is
* {@link Object }
*
*/
public void setOrLonger(Object value) {
this.orLonger = value;
}
/**
* Gets the value of the prefixLengthRange property.
*
* @return
* possible object is
* {@link List.RouteList.RouteFilter.MatchType.PrefixLengthRange }
*
*/
public List.RouteList.RouteFilter.MatchType.PrefixLengthRange getPrefixLengthRange() {
return prefixLengthRange;
}
/**
* Sets the value of the prefixLengthRange property.
*
* @param value
* allowed object is
* {@link List.RouteList.RouteFilter.MatchType.PrefixLengthRange }
*
*/
public void setPrefixLengthRange(List.RouteList.RouteFilter.MatchType.PrefixLengthRange value) {
this.prefixLengthRange = value;
}
/**
* Gets the value of the through property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getThrough() {
return through;
}
/**
* Sets the value of the through property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setThrough(String value) {
this.through = value;
}
/**
* Gets the value of the upto property.
*
* @return
* possible object is
* {@link List.RouteList.RouteFilter.MatchType.Upto }
*
*/
public List.RouteList.RouteFilter.MatchType.Upto getUpto() {
return upto;
}
/**
* Sets the value of the upto property.
*
* @param value
* allowed object is
* {@link List.RouteList.RouteFilter.MatchType.Upto }
*
*/
public void setUpto(List.RouteList.RouteFilter.MatchType.Upto value) {
this.upto = value;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <attribute name="lower_bound" type="{http://www.w3.org/2001/XMLSchema}integer" />
* <attribute name="upper_bound" type="{http://www.w3.org/2001/XMLSchema}integer" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "")
public static class PrefixLengthRange {
@XmlAttribute(name = "lower_bound")
protected BigInteger lowerBound;
@XmlAttribute(name = "upper_bound")
protected BigInteger upperBound;
/**
* Gets the value of the lowerBound property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getLowerBound() {
return lowerBound;
}
/**
* Sets the value of the lowerBound property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setLowerBound(BigInteger value) {
this.lowerBound = value;
}
/**
* Gets the value of the upperBound property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getUpperBound() {
return upperBound;
}
/**
* Sets the value of the upperBound property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setUpperBound(BigInteger value) {
this.upperBound = value;
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="prefixLength" type="{http://www.xmlnetwork.org}prefixLength"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"prefixLength"
})
public static class Upto {
protected int prefixLength;
/**
* Gets the value of the prefixLength property.
*
*/
public int getPrefixLength() {
return prefixLength;
}
/**
* Sets the value of the prefixLength property.
*
*/
public void setPrefixLength(int value) {
this.prefixLength = value;
}
}
}
}
}
}