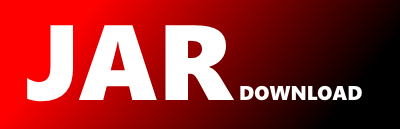
org.xmlnetwork.ObjectFactory Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.0.2-b01-fcs
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2008.06.03 at 04:27:27 PM CEST
//
package org.xmlnetwork;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.namespace.QName;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the org.xmlnetwork package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
*
*/
@XmlRegistry
public class ObjectFactory {
private final static QName _General_QNAME = new QName("http://www.xmlnetwork.org", "General");
private final static QName _Redistribution_QNAME = new QName("http://www.xmlnetwork.org", "Redistribution");
private final static QName _NatTypeTranslate_QNAME = new QName("http://www.xmlnetwork.org", "translate");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: org.xmlnetwork
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link LogType }
*
*/
public LogType createLogType() {
return new LogType();
}
/**
* Create an instance of {@link PrefixLimit.Teardown }
*
*/
public PrefixLimit.Teardown createPrefixLimitTeardown() {
return new PrefixLimit.Teardown();
}
/**
* Create an instance of {@link Port }
*
*/
public Port createPort() {
return new Port();
}
/**
* Create an instance of {@link PrefixLimit }
*
*/
public PrefixLimit createPrefixLimit() {
return new PrefixLimit();
}
/**
* Create an instance of {@link BGPConf.AggregateRoutes.Route }
*
*/
public BGPConf.AggregateRoutes.Route createBGPConfAggregateRoutesRoute() {
return new BGPConf.AggregateRoutes.Route();
}
/**
* Create an instance of {@link MasqueradeType }
*
*/
public MasqueradeType createMasqueradeType() {
return new MasqueradeType();
}
/**
* Create an instance of {@link Firewalls }
*
*/
public Firewalls createFirewalls() {
return new Firewalls();
}
/**
* Create an instance of {@link IntNets.N }
*
*/
public IntNets.N createIntNetsN() {
return new IntNets.N();
}
/**
* Create an instance of {@link IntNets }
*
*/
public IntNets createIntNets() {
return new IntNets();
}
/**
* Create an instance of {@link Confederations.C }
*
*/
public Confederations.C createConfederationsC() {
return new Confederations.C();
}
/**
* Create an instance of {@link BGPConf.StaticRoutes.S }
*
*/
public BGPConf.StaticRoutes.S createBGPConfStaticRoutesS() {
return new BGPConf.StaticRoutes.S();
}
/**
* Create an instance of {@link GeneralRules.SetRules.List }
*
*/
public GeneralRules.SetRules.List createGeneralRulesSetRulesList() {
return new GeneralRules.SetRules.List();
}
/**
* Create an instance of {@link Group.Authentication }
*
*/
public Group.Authentication createGroupAuthentication() {
return new Group.Authentication();
}
/**
* Create an instance of {@link Neighbor }
*
*/
public Neighbor createNeighbor() {
return new Neighbor();
}
/**
* Create an instance of {@link org.xmlnetwork.List.CommunityList.Community }
*
*/
public org.xmlnetwork.List.CommunityList.Community createListCommunityListCommunity() {
return new org.xmlnetwork.List.CommunityList.Community();
}
/**
* Create an instance of {@link RipConf }
*
*/
public RipConf createRipConf() {
return new RipConf();
}
/**
* Create an instance of {@link Neighbor.NextHopSelf }
*
*/
public Neighbor.NextHopSelf createNeighborNextHopSelf() {
return new Neighbor.NextHopSelf();
}
/**
* Create an instance of {@link Group }
*
*/
public Group createGroup() {
return new Group();
}
/**
* Create an instance of {@link GeneralRules.MatchConditions.PacketLength }
*
*/
public GeneralRules.MatchConditions.PacketLength createGeneralRulesMatchConditionsPacketLength() {
return new GeneralRules.MatchConditions.PacketLength();
}
/**
* Create an instance of {@link BGPConf }
*
*/
public BGPConf createBGPConf() {
return new BGPConf();
}
/**
* Create an instance of {@link GeneralRules.SetRules.MetricIgp }
*
*/
public GeneralRules.SetRules.MetricIgp createGeneralRulesSetRulesMetricIgp() {
return new GeneralRules.SetRules.MetricIgp();
}
/**
* Create an instance of {@link InterfType }
*
*/
public InterfType createInterfType() {
return new InterfType();
}
/**
* Create an instance of {@link org.xmlnetwork.Authentication.ApplyToInterface }
*
*/
public org.xmlnetwork.Authentication.ApplyToInterface createAuthenticationApplyToInterface() {
return new org.xmlnetwork.Authentication.ApplyToInterface();
}
/**
* Create an instance of {@link GeneralRules.MatchConditions.List }
*
*/
public GeneralRules.MatchConditions.List createGeneralRulesMatchConditionsList() {
return new GeneralRules.MatchConditions.List();
}
/**
* Create an instance of {@link Group.NextHopSelf }
*
*/
public Group.NextHopSelf createGroupNextHopSelf() {
return new Group.NextHopSelf();
}
/**
* Create an instance of {@link org.xmlnetwork.Authentication.KeyChain.KeyList }
*
*/
public org.xmlnetwork.Authentication.KeyChain.KeyList createAuthenticationKeyChainKeyList() {
return new org.xmlnetwork.Authentication.KeyChain.KeyList();
}
/**
* Create an instance of {@link Routers }
*
*/
public Routers createRouters() {
return new Routers();
}
/**
* Create an instance of {@link Group.DefaultOriginate }
*
*/
public Group.DefaultOriginate createGroupDefaultOriginate() {
return new Group.DefaultOriginate();
}
/**
* Create an instance of {@link Peerings.P }
*
*/
public Peerings.P createPeeringsP() {
return new Peerings.P();
}
/**
* Create an instance of {@link Peerings.P.PeeringSide }
*
*/
public Peerings.P.PeeringSide createPeeringsPPeeringSide() {
return new Peerings.P.PeeringSide();
}
/**
* Create an instance of {@link ConstructType }
*
*/
public ConstructType createConstructType() {
return new ConstructType();
}
/**
* Create an instance of {@link org.xmlnetwork.Authentication.KeyChain.KeyList.Key }
*
*/
public org.xmlnetwork.Authentication.KeyChain.KeyList.Key createAuthenticationKeyChainKeyListKey() {
return new org.xmlnetwork.Authentication.KeyChain.KeyList.Key();
}
/**
* Create an instance of {@link org.xmlnetwork.List.RouteList.RouteFilter.MatchType.PrefixLengthRange }
*
*/
public org.xmlnetwork.List.RouteList.RouteFilter.MatchType.PrefixLengthRange createListRouteListRouteFilterMatchTypePrefixLengthRange() {
return new org.xmlnetwork.List.RouteList.RouteFilter.MatchType.PrefixLengthRange();
}
/**
* Create an instance of {@link RoutingPols.P }
*
*/
public RoutingPols.P createRoutingPolsP() {
return new RoutingPols.P();
}
/**
* Create an instance of {@link GeneralRules }
*
*/
public GeneralRules createGeneralRules() {
return new GeneralRules();
}
/**
* Create an instance of {@link AclType }
*
*/
public AclType createAclType() {
return new AclType();
}
/**
* Create an instance of {@link Multiport }
*
*/
public Multiport createMultiport() {
return new Multiport();
}
/**
* Create an instance of {@link Latencies.Latency }
*
*/
public Latencies.Latency createLatenciesLatency() {
return new Latencies.Latency();
}
/**
* Create an instance of {@link NetML }
*
*/
public NetML createNetML() {
return new NetML();
}
/**
* Create an instance of {@link org.xmlnetwork.StaticRoutes }
*
*/
public org.xmlnetwork.StaticRoutes createStaticRoutes() {
return new org.xmlnetwork.StaticRoutes();
}
/**
* Create an instance of {@link GeneralRules.MatchConditions }
*
*/
public GeneralRules.MatchConditions createGeneralRulesMatchConditions() {
return new GeneralRules.MatchConditions();
}
/**
* Create an instance of {@link BGPConf.StaticRoutes }
*
*/
public BGPConf.StaticRoutes createBGPConfStaticRoutes() {
return new BGPConf.StaticRoutes();
}
/**
* Create an instance of {@link RoutingPols }
*
*/
public RoutingPols createRoutingPols() {
return new RoutingPols();
}
/**
* Create an instance of {@link IpPref }
*
*/
public IpPref createIpPref() {
return new IpPref();
}
/**
* Create an instance of {@link RoutingPols.P.Filter }
*
*/
public RoutingPols.P.Filter createRoutingPolsPFilter() {
return new RoutingPols.P.Filter();
}
/**
* Create an instance of {@link Networks.N.CollisionDomains.C.Iface }
*
*/
public Networks.N.CollisionDomains.C.Iface createNetworksNCollisionDomainsCIface() {
return new Networks.N.CollisionDomains.C.Iface();
}
/**
* Create an instance of {@link RipConf.StaticRoute }
*
*/
public RipConf.StaticRoute createRipConfStaticRoute() {
return new RipConf.StaticRoute();
}
/**
* Create an instance of {@link BGPConf.AggregateRoutes }
*
*/
public BGPConf.AggregateRoutes createBGPConfAggregateRoutes() {
return new BGPConf.AggregateRoutes();
}
/**
* Create an instance of {@link Lifetime }
*
*/
public Lifetime createLifetime() {
return new Lifetime();
}
/**
* Create an instance of {@link Latencies }
*
*/
public Latencies createLatencies() {
return new Latencies();
}
/**
* Create an instance of {@link NatType }
*
*/
public NatType createNatType() {
return new NatType();
}
/**
* Create an instance of {@link GeneralRules.SetRules.CommunityList }
*
*/
public GeneralRules.SetRules.CommunityList createGeneralRulesSetRulesCommunityList() {
return new GeneralRules.SetRules.CommunityList();
}
/**
* Create an instance of {@link Peerings }
*
*/
public Peerings createPeerings() {
return new Peerings();
}
/**
* Create an instance of {@link org.xmlnetwork.Interface }
*
*/
public org.xmlnetwork.Interface createInterface() {
return new org.xmlnetwork.Interface();
}
/**
* Create an instance of {@link RteReflect.Cluster }
*
*/
public RteReflect.Cluster createRteReflectCluster() {
return new RteReflect.Cluster();
}
/**
* Create an instance of {@link PolicyCall }
*
*/
public PolicyCall createPolicyCall() {
return new PolicyCall();
}
/**
* Create an instance of {@link As }
*
*/
public As createAs() {
return new As();
}
/**
* Create an instance of {@link org.xmlnetwork.List.CommunityList }
*
*/
public org.xmlnetwork.List.CommunityList createListCommunityList() {
return new org.xmlnetwork.List.CommunityList();
}
/**
* Create an instance of {@link Neighbor.Authentication }
*
*/
public Neighbor.Authentication createNeighborAuthentication() {
return new Neighbor.Authentication();
}
/**
* Create an instance of {@link org.xmlnetwork.List.RouteList }
*
*/
public org.xmlnetwork.List.RouteList createListRouteList() {
return new org.xmlnetwork.List.RouteList();
}
/**
* Create an instance of {@link GeneralRules.SetRules }
*
*/
public GeneralRules.SetRules createGeneralRulesSetRules() {
return new GeneralRules.SetRules();
}
/**
* Create an instance of {@link BgpTimers }
*
*/
public BgpTimers createBgpTimers() {
return new BgpTimers();
}
/**
* Create an instance of {@link Distribution }
*
*/
public Distribution createDistribution() {
return new Distribution();
}
/**
* Create an instance of {@link Neighbor.PeerAS }
*
*/
public Neighbor.PeerAS createNeighborPeerAS() {
return new Neighbor.PeerAS();
}
/**
* Create an instance of {@link org.xmlnetwork.List.RouteList.RouteFilter.MatchType }
*
*/
public org.xmlnetwork.List.RouteList.RouteFilter.MatchType createListRouteListRouteFilterMatchType() {
return new org.xmlnetwork.List.RouteList.RouteFilter.MatchType();
}
/**
* Create an instance of {@link RteReflect.Cluster.Client }
*
*/
public RteReflect.Cluster.Client createRteReflectClusterClient() {
return new RteReflect.Cluster.Client();
}
/**
* Create an instance of {@link GeneralType }
*
*/
public GeneralType createGeneralType() {
return new GeneralType();
}
/**
* Create an instance of {@link Networks.N.CollisionDomains.C }
*
*/
public Networks.N.CollisionDomains.C createNetworksNCollisionDomainsC() {
return new Networks.N.CollisionDomains.C();
}
/**
* Create an instance of {@link BGPConf.Authentication }
*
*/
public BGPConf.Authentication createBGPConfAuthentication() {
return new BGPConf.Authentication();
}
/**
* Create an instance of {@link Neighbour }
*
*/
public Neighbour createNeighbour() {
return new Neighbour();
}
/**
* Create an instance of {@link Networks.N }
*
*/
public Networks.N createNetworksN() {
return new Networks.N();
}
/**
* Create an instance of {@link GeneralRules.SetRules.Characteristics }
*
*/
public GeneralRules.SetRules.Characteristics createGeneralRulesSetRulesCharacteristics() {
return new GeneralRules.SetRules.Characteristics();
}
/**
* Create an instance of {@link Networks }
*
*/
public Networks createNetworks() {
return new Networks();
}
/**
* Create an instance of {@link RoutingPols.P.PeeringSpecification }
*
*/
public RoutingPols.P.PeeringSpecification createRoutingPolsPPeeringSpecification() {
return new RoutingPols.P.PeeringSpecification();
}
/**
* Create an instance of {@link org.xmlnetwork.List }
*
*/
public org.xmlnetwork.List createList() {
return new org.xmlnetwork.List();
}
/**
* Create an instance of {@link org.xmlnetwork.List.RouteList.RouteFilter.MatchType.Upto }
*
*/
public org.xmlnetwork.List.RouteList.RouteFilter.MatchType.Upto createListRouteListRouteFilterMatchTypeUpto() {
return new org.xmlnetwork.List.RouteList.RouteFilter.MatchType.Upto();
}
/**
* Create an instance of {@link RuleType }
*
*/
public RuleType createRuleType() {
return new RuleType();
}
/**
* Create an instance of {@link Neighbor.LocalAS }
*
*/
public Neighbor.LocalAS createNeighborLocalAS() {
return new Neighbor.LocalAS();
}
/**
* Create an instance of {@link Networks.N.CollisionDomains }
*
*/
public Networks.N.CollisionDomains createNetworksNCollisionDomains() {
return new Networks.N.CollisionDomains();
}
/**
* Create an instance of {@link GeneralRules.SetRules.Interface }
*
*/
public GeneralRules.SetRules.Interface createGeneralRulesSetRulesInterface() {
return new GeneralRules.SetRules.Interface();
}
/**
* Create an instance of {@link Confederations }
*
*/
public Confederations createConfederations() {
return new Confederations();
}
/**
* Create an instance of {@link BorderRouters.R }
*
*/
public BorderRouters.R createBorderRoutersR() {
return new BorderRouters.R();
}
/**
* Create an instance of {@link RouterConf }
*
*/
public RouterConf createRouterConf() {
return new RouterConf();
}
/**
* Create an instance of {@link org.xmlnetwork.StaticRoute }
*
*/
public org.xmlnetwork.StaticRoute createStaticRoute() {
return new org.xmlnetwork.StaticRoute();
}
/**
* Create an instance of {@link RteReflect }
*
*/
public RteReflect createRteReflect() {
return new RteReflect();
}
/**
* Create an instance of {@link Neighbor.UpdateSource }
*
*/
public Neighbor.UpdateSource createNeighborUpdateSource() {
return new Neighbor.UpdateSource();
}
/**
* Create an instance of {@link BGPConf.DefaultOriginate }
*
*/
public BGPConf.DefaultOriginate createBGPConfDefaultOriginate() {
return new BGPConf.DefaultOriginate();
}
/**
* Create an instance of {@link FwallConf }
*
*/
public FwallConf createFwallConf() {
return new FwallConf();
}
/**
* Create an instance of {@link Group.PeerAS }
*
*/
public Group.PeerAS createGroupPeerAS() {
return new Group.PeerAS();
}
/**
* Create an instance of {@link NatRedirect }
*
*/
public NatRedirect createNatRedirect() {
return new NatRedirect();
}
/**
* Create an instance of {@link org.xmlnetwork.List.ASPathList }
*
*/
public org.xmlnetwork.List.ASPathList createListASPathList() {
return new org.xmlnetwork.List.ASPathList();
}
/**
* Create an instance of {@link RedistributionType }
*
*/
public RedistributionType createRedistributionType() {
return new RedistributionType();
}
/**
* Create an instance of {@link org.xmlnetwork.List.RouteList.RouteFilter }
*
*/
public org.xmlnetwork.List.RouteList.RouteFilter createListRouteListRouteFilter() {
return new org.xmlnetwork.List.RouteList.RouteFilter();
}
/**
* Create an instance of {@link org.xmlnetwork.Policy }
*
*/
public org.xmlnetwork.Policy createPolicy() {
return new org.xmlnetwork.Policy();
}
/**
* Create an instance of {@link BGPConf.Dampening }
*
*/
public BGPConf.Dampening createBGPConfDampening() {
return new BGPConf.Dampening();
}
/**
* Create an instance of {@link BorderRouters }
*
*/
public BorderRouters createBorderRouters() {
return new BorderRouters();
}
/**
* Create an instance of {@link Group.LocalAS }
*
*/
public Group.LocalAS createGroupLocalAS() {
return new Group.LocalAS();
}
/**
* Create an instance of {@link Group.UpdateSource }
*
*/
public Group.UpdateSource createGroupUpdateSource() {
return new Group.UpdateSource();
}
/**
* Create an instance of {@link org.xmlnetwork.Authentication }
*
*/
public org.xmlnetwork.Authentication createAuthentication() {
return new org.xmlnetwork.Authentication();
}
/**
* Create an instance of {@link Confederations.C.ASMember }
*
*/
public Confederations.C.ASMember createConfederationsCASMember() {
return new Confederations.C.ASMember();
}
/**
* Create an instance of {@link RipConf.Policy }
*
*/
public RipConf.Policy createRipConfPolicy() {
return new RipConf.Policy();
}
/**
* Create an instance of {@link Portrange }
*
*/
public Portrange createPortrange() {
return new Portrange();
}
/**
* Create an instance of {@link org.xmlnetwork.List.PrefixList }
*
*/
public org.xmlnetwork.List.PrefixList createListPrefixList() {
return new org.xmlnetwork.List.PrefixList();
}
/**
* Create an instance of {@link OptionType }
*
*/
public OptionType createOptionType() {
return new OptionType();
}
/**
* Create an instance of {@link ListCall }
*
*/
public ListCall createListCall() {
return new ListCall();
}
/**
* Create an instance of {@link AsList }
*
*/
public AsList createAsList() {
return new AsList();
}
/**
* Create an instance of {@link org.xmlnetwork.Authentication.KeyChain }
*
*/
public org.xmlnetwork.Authentication.KeyChain createAuthenticationKeyChain() {
return new org.xmlnetwork.Authentication.KeyChain();
}
/**
* Create an instance of {@link Group.RemovePrivateAS }
*
*/
public Group.RemovePrivateAS createGroupRemovePrivateAS() {
return new Group.RemovePrivateAS();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link GeneralType }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://www.xmlnetwork.org", name = "General")
public JAXBElement createGeneral(GeneralType value) {
return new JAXBElement(_General_QNAME, GeneralType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link RedistributionType }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://www.xmlnetwork.org", name = "Redistribution")
public JAXBElement createRedistribution(RedistributionType value) {
return new JAXBElement(_Redistribution_QNAME, RedistributionType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link MasqueradeType }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://www.xmlnetwork.org", name = "translate", scope = NatType.class)
public JAXBElement createNatTypeTranslate(MasqueradeType value) {
return new JAXBElement(_NatTypeTranslate_QNAME, MasqueradeType.class, NatType.class, value);
}
}