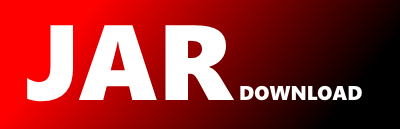
org.objectweb.fractal.bf.hdl.reader.GraphBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fractal-bf-hdl-reader Show documentation
Show all versions of fractal-bf-hdl-reader Show documentation
Support input/output operations on HDL entities
The newest version!
package org.objectweb.fractal.bf.hdl.reader;
import java.util.HashMap;
import java.util.LinkedHashSet;
import java.util.Map;
import java.util.Set;
import jdsl.graph.api.Edge;
import jdsl.graph.api.Graph;
import jdsl.graph.api.Vertex;
import jdsl.graph.ref.IncidenceListGraph;
import org.objectweb.fractal.bf.hdl.graph.Path;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.xmlnetwork.Latencies;
import org.xmlnetwork.NetML;
import org.xmlnetwork.Latencies.Latency;
/**
*A builder for a graph
*
*/
public class GraphBuilder
{
private static Logger logger = LoggerFactory.getLogger(GraphBuilder.class);
/**
* implicit description of graph's edges
*/
private static Map edges = new HashMap();
/**
* keep track of id for each created vertex
*/
private static Map hostMachineToVertex = new HashMap();
/**
* Build a graph from a weights description. For every subsequent request of building a graph, internal data structures
* are initialized, and theGraph is refreshed.
* This means that references to edges and vertices of the constructed graph are lost every time
* a new graph is built.
*
* @param netml the input {@link NetML} object as read by the {@link NetMLReader}
* @return a {@link Graph}
*/
public static Graph build(NetML netml) throws GraphBuilderException
{
if (netml == null) {
throw new GraphBuilderException("Cannot build graph topology from null NetML file");
}
edges.clear();
hostMachineToVertex.clear();
final Latencies latencies = netml.getLatencies();
//new empty graph
Graph theGraph = new IncidenceListGraph();
//ADD VERTICES TO THE GRAPH
addVertices(theGraph, latencies);
//CREATE EDGES BETWEEN VERTICES
addEdges(theGraph, latencies);
logger.debug("GraphBuilder built graph: "+theGraph.toString());
return theGraph;
}
/**
* @param theGraph
* @param latencies
* @throws GraphBuilderException
*/
private static void addEdges(Graph theGraph, Latencies latencies) throws GraphBuilderException
{
for (Latencies.Latency latency : latencies.getLatency())
{
final String xmlFrom = latency.getFrom();
String hostNameFrom = parseHostMachineFromLatencyAttribute(xmlFrom);
Vertex from = hostMachineToVertex.get(hostNameFrom);
final String xmlTo = latency.getTo();
String hostNameTo = parseHostMachineFromLatencyAttribute(xmlTo);
Vertex to = hostMachineToVertex.get(hostNameTo);
String fromIp = parseIpAddressFromLatencyAttribute(xmlFrom);
String toIp = parseIpAddressFromLatencyAttribute(xmlTo);
final Integer edgeLatency = latency.getValue();
PairOfIps poi = new PairOfIps(fromIp, toIp, edgeLatency);
logger.debug("Adding edge between " + from + " and " + to
+ " decorated with: " + poi);
final Edge insertedEdge = theGraph.insertDirectedEdge(from, to, poi); //restore undirected edge in case of problems
insertedEdge.set("latency", edgeLatency);
insertedEdge.set("poi", poi);
/*this might be wrong*/
PairOfIps inversePoi = new PairOfIps(toIp, fromIp, edgeLatency);
final Edge reversedEdge = theGraph.insertDirectedEdge(to, from, inversePoi);
reversedEdge.set("latency", edgeLatency);
reversedEdge.set("poi", inversePoi);
}
}
/**
*
*
* @param theGraph the (empty) graph where vertices are going to be added
* @param latencies xml representation of the vertices
*/
private static void addVertices(Graph theGraph, Latencies latencies)
{
for (Latency l: latencies.getLatency()) {
String from = l.getFrom();
addVertexForHostName(theGraph, from);
String to = l.getTo();
addVertexForHostName(theGraph, to);
}
}
/**
* @param theGraph
* @param hostName
*/
private static void addVertexForHostName(Graph theGraph, String hostName)
{
String hostNameTo = parseHostMachineFromLatencyAttribute(hostName);
if (!hostMachineToVertex.containsKey(hostNameTo))
{
final Vertex vertexTo = theGraph.insertVertex(hostNameTo);
hostMachineToVertex.put(hostNameTo, vertexTo);
}
}
/**
* @param an attribute of the xml element
* @return the host name, identifying a specific node of the graph
*/
private static String parseHostMachineFromLatencyAttribute(String from)
{
String hostMachine = from.split("_")[0];
return hostMachine;
}
/**
* @param an attribute of the xml element
* @return the host name, identifying a specific node of the graph
*/
private static String parseIpAddressFromLatencyAttribute(String latencyAttribute)
{
String ip = latencyAttribute.split("_")[1];
return ip;
}
/**
* @param latencies
* @return
*/
public static Set allNodeIds(Latencies latencies)
{
Set nodes = new LinkedHashSet();
// search all node ids
for (Latency w : latencies.getLatency())
{
final String from = w.getFrom();
nodes.add(from);
final String to = w.getTo();
nodes.add(to);
edges.put(from, to);
}
return nodes;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy