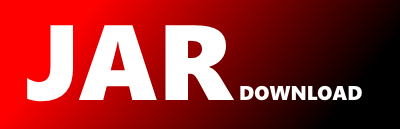
org.objectweb.fractal.bf.hdl.reader.NetMLUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fractal-bf-hdl-reader Show documentation
Show all versions of fractal-bf-hdl-reader Show documentation
Support input/output operations on HDL entities
The newest version!
/**
* Author: Valerio Schiavoni
*/
package org.objectweb.fractal.bf.hdl.reader;
import java.util.List;
import org.xmlnetwork.Interface;
import org.xmlnetwork.NetML;
import org.xmlnetwork.RouterConf;
/**
* @author Valerio Schiavoni
*
*/
public class NetMLUtils
{
/**
* Return the name of the host that has one of its interfaces configured with the specified IP address.
* @param input the {@link NetML} object
* @param ip the string representation of the IP
* @return the name of the host, null if not found
*/
public static String findHostByIpAddress(NetML input, String ip) {
List routerConf = input.getRouters().getRouterConf();
for (RouterConf rc : routerConf)
{
List interfaces = rc.getInterface();
for (Interface i : interfaces)
{
List ipAddresses = i.getIpAddress();
for (String ipaddr : ipAddresses)
{
if (ipaddr.equalsIgnoreCase(ip))
{
return rc.getHostname();
}
}
}
}
return null;
}
/**
* Assume 'eth0' as the only available ethernet interface
*
* @param input
* @param hostName
* @return
*/
public static String findIpAddressByHostName(NetML input, String hostName)
{
return findIpAddressByHostName(input, hostName, "eth0");
}
/**
* Assume 'eth0' as the only available ethernet interface
*
* @param input
* @param hostNameAndIp
* @param itfName interface name, as specified in the NetML file
* @return
*/
public static String findIpAddressByHostName(NetML input, String hostNameAndIp,
String itfName)
{
String ip = null;
/**
* The weights attribute from_host and to_host are expected in the following form:
* {hostname}-{ip_address}:{port}
*
*/
String hostName = hostNameAndIp.split("-")[0];
RouterConf rc = findRouterConfByHostName(
input.getRouters().getRouterConf(), hostName);
if (rc == null) {
throw new RuntimeException("Cannot find a router configuration for host: "+hostName);
}
for (Interface routerItf : rc.getInterface())
{
if (routerItf.getName().equalsIgnoreCase(itfName))
{
ip = routerItf.getIpAddress().get(0);
break;
}
}
return ip;
}
public static RouterConf findRouterConfByHostName(List confs,
String hostName)
{
RouterConf res = null;
for (RouterConf rc : confs)
{
if (rc.getHostname().equals(hostName))
{
res = rc;
break;
}
}
return res;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy