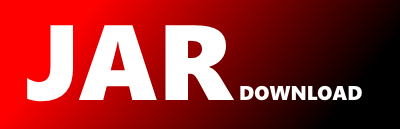
org.objectweb.fractal.julia.control.binding.AutoBindingMixin Maven / Gradle / Ivy
The newest version!
/***
* Julia: France Telecom's implementation of the Fractal API
* Copyright (C) 2001-2011 France Telecom R&D
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* Contact: [email protected]
*
* Author: Eric Bruneton
*/
package org.objectweb.fractal.julia.control.binding;
import org.objectweb.fractal.api.Component;
import org.objectweb.fractal.api.Interface;
import org.objectweb.fractal.api.NoSuchInterfaceException;
import org.objectweb.fractal.api.control.BindingController;
import org.objectweb.fractal.api.control.ContentController;
import org.objectweb.fractal.api.control.IllegalBindingException;
import org.objectweb.fractal.api.control.IllegalLifeCycleException;
import org.objectweb.fractal.api.type.InterfaceType;
/**
* A mixin to simplify the management of collection interfaces with composite
* components.
*
*
* Requirements
*
* - TODO.
*
*/
public abstract class AutoBindingMixin {
// -------------------------------------------------------------------------
// Private constructor
// -------------------------------------------------------------------------
private AutoBindingMixin () {
}
// -------------------------------------------------------------------------
// Fields and methods added and overriden by the mixin class
// -------------------------------------------------------------------------
/**
* Calls the overriden method, and then creates a corresponding internal
* binding, if necessary. This internal binding is created only if the
* interface is an external client collection interface "aXXX", and if there
* is a "model binding" to the internal server collection interface "a".
*
* @param clientItfType the type of the clientItfName interface.
* @param clientItfName the name of a client interface of the component to
* which this interface belongs.
* @param serverItf a server interface.
* @throws NoSuchInterfaceException if there is no such client interface.
* @throws IllegalBindingException if the binding cannot be created.
* @throws IllegalLifeCycleException if this component has a {@link
* org.objectweb.fractal.api.control.LifeCycleController} interface, but
* it is not in an appropriate state to perform this operation.
*/
public void bindFc (
final InterfaceType clientItfType,
final String clientItfName,
final Object serverItf)
throws
NoSuchInterfaceException,
IllegalBindingException,
IllegalLifeCycleException
{
_super_bindFc(clientItfType, clientItfName, serverItf);
if (clientItfType.isFcCollectionItf()) {
String sItfName = clientItfType.getFcItfName();
ContentController cc;
try {
cc = (ContentController)_this_weaveableC.getFcInterface("content-controller");
} catch (NoSuchInterfaceException e) {
cc = null;
}
if (cc != null && !clientItfName.equals(sItfName)) {
Object sItf = cc.getFcInternalInterface(sItfName);
Object itf = cc.getFcInternalInterface(clientItfName);
try {
Object[] itfs = Util.getFcClientItfsBoundTo((Interface)sItf).toArray();
for (int i = 0; i < itfs.length; ++i) {
Interface cItf = (Interface)itfs[i];
InterfaceType cItfType = (InterfaceType)cItf.getFcItfType();
if (cItfType.isFcCollectionItf()) {
Component c = cItf.getFcItfOwner();
BindingController bc =
(BindingController)c.getFcInterface("binding-controller");
String s =
cItfType.getFcItfName() + clientItfName.substring(sItfName.length());
bc.bindFc(s, itf);
}
}
} catch (Exception e) {
throw new ChainedIllegalBindingException(
e, _this_weaveableC, null, clientItfName, null,
"Error during automatic creation of bindings from the model binding");
}
}
if (clientItfName.equals(sItfName)) {
Interface sItf = (Interface)serverItf;
InterfaceType sItfType = (InterfaceType)sItf.getFcItfType();
if (sItf.isFcInternalItf() &&
sItfType.isFcCollectionItf() &&
sItf.getFcItfName().equals(sItfType.getFcItfName()))
{
Component sComp = sItf.getFcItfOwner();
try {
BindingController bc =
(BindingController)sComp.getFcInterface("binding-controller");
cc =
(ContentController)sComp.getFcInterface("content-controller");
String s = sItf.getFcItfName();
String[] itfs = bc.listFc();
for (int i = 0; i < itfs.length; ++i) {
if (itfs[i].length() > s.length() && itfs[i].startsWith(s)) {
_this_bindFc(
clientItfName + itfs[i].substring(s.length()),
cc.getFcInternalInterface(itfs[i]));
}
}
} catch (Exception e) {
throw new ChainedIllegalBindingException(
e, _this_weaveableC, null, clientItfName, null,
"Error during automatic creation of bindings from the model binding");
}
}
}
}
}
/**
* Calls the overriden method, and then removes the corresponding internal
* bindings, if necessary. This internal binding is removed only if the
* interface is an external client collection interface "aXXX", and if there
* is a "model binding" to the internal server collection interface "a".
* @param clientItfType the type of the clientItfName interface.
* @param clientItfName the name of a client interface of the component to
* which this interface belongs.
* @throws NoSuchInterfaceException if there is no such client interface.
* @throws IllegalBindingException if the binding cannot be removed.
* @throws IllegalLifeCycleException if this component has a {@link
* org.objectweb.fractal.api.control.LifeCycleController} interface, but
* it is not in an appropriate state to perform this operation.
*/
public void unbindFc (
final InterfaceType clientItfType,
final String clientItfName)
throws
NoSuchInterfaceException,
IllegalBindingException,
IllegalLifeCycleException
{
_super_unbindFc(clientItfType, clientItfName);
if (clientItfType.isFcCollectionItf()) {
ContentController cc;
try {
cc = (ContentController)_this_weaveableC.getFcInterface("content-controller");
} catch (NoSuchInterfaceException e) {
return;
}
String sItfName = clientItfType.getFcItfName();
Object sItf = cc.getFcInternalInterface(sItfName);
try {
Object[] itfs = Util.getFcClientItfsBoundTo((Interface)sItf).toArray();
for (int i = 0; i < itfs.length; ++i) {
Interface cItf = (Interface)itfs[i];
InterfaceType cItfType = (InterfaceType)cItf.getFcItfType();
if (cItfType.isFcCollectionItf()) {
Component c = cItf.getFcItfOwner();
BindingController bc =
(BindingController)c.getFcInterface("binding-controller");
String s =
cItfType.getFcItfName() + clientItfName.substring(sItfName.length());
bc.unbindFc(s);
}
}
} catch (Exception e) {
throw new ChainedIllegalBindingException(
e, _this_weaveableC, null, clientItfName, null,
"Error during automatic destruction of bindings from the model binding");
}
}
}
// -------------------------------------------------------------------------
// Fields and methods required by the mixin class in the base class
// -------------------------------------------------------------------------
/**
* The weaveableC field required by this mixin. This field is
* supposed to reference the {@link Component} interface of the component to
* which this controller object belongs.
*/
public Component _this_weaveableC;
/**
* The {@link BindingController#bindFc bindFc} method required by this mixin.
*
* @param clientItfName the name of a client interface of the component to
* which this interface belongs.
* @param serverItf a server interface.
* @throws NoSuchInterfaceException if there is no such client interface.
* @throws IllegalBindingException if the binding cannot be created.
* @throws IllegalLifeCycleException if this component has a {@link
* org.objectweb.fractal.api.control.LifeCycleController} interface, but it is not in an appropriate state
* to perform this operation.
*/
public abstract void _this_bindFc (String clientItfName, Object serverItf) throws
NoSuchInterfaceException,
IllegalBindingException,
IllegalLifeCycleException;
/**
* The {@link TypeBindingMixin#bindFc(InterfaceType,String,Object) bindFc}
* method overriden by this mixin.
*
* @param clientItfType the type of the clientItfName interface.
* @param clientItfName the name of a client interface of the component to
* which this interface belongs.
* @param serverItf a server interface.
* @throws NoSuchInterfaceException if there is no such client interface.
* @throws IllegalBindingException if the binding cannot be created.
* @throws IllegalLifeCycleException if this component has a {@link
* org.objectweb.fractal.api.control.LifeCycleController} interface, but
* it is not in an appropriate state to perform this operation.
*/
public abstract void _super_bindFc (
InterfaceType clientItfType,
String clientItfName,
Object serverItf)
throws
NoSuchInterfaceException,
IllegalBindingException,
IllegalLifeCycleException;
/**
* The {@link TypeBindingMixin#unbindFc(InterfaceType,String) unbindFc}
* method overriden by this mixin.
*
* @param clientItfType the type of the clientItfName interface.
* @param clientItfName the name of a client interface of the component to
* which this interface belongs.
* @throws NoSuchInterfaceException if there is no such client interface.
* @throws IllegalBindingException if the binding cannot be removed.
* @throws IllegalLifeCycleException if this component has a {@link
* org.objectweb.fractal.api.control.LifeCycleController} interface, but
* it is not in an appropriate state to perform this operation.
*/
public abstract void _super_unbindFc (
InterfaceType clientItfType,
String clientItfName)
throws
NoSuchInterfaceException,
IllegalBindingException,
IllegalLifeCycleException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy