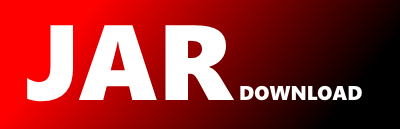
org.objectweb.fractal.julia.control.binding.ContentBindingMixin Maven / Gradle / Ivy
The newest version!
/***
* Julia: France Telecom's implementation of the Fractal API
* Copyright (C) 2001-2011 France Telecom R&D
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* Contact: [email protected]
*
* Author: Eric Bruneton
*/
package org.objectweb.fractal.julia.control.binding;
import org.objectweb.fractal.api.Component;
import org.objectweb.fractal.api.Interface;
import org.objectweb.fractal.api.NoSuchInterfaceException;
import org.objectweb.fractal.api.control.BindingController;
import org.objectweb.fractal.api.control.ContentController;
import org.objectweb.fractal.api.control.IllegalBindingException;
import org.objectweb.fractal.api.control.IllegalLifeCycleException;
import org.objectweb.fractal.api.control.SuperController;
import org.objectweb.fractal.api.type.InterfaceType;
/**
* Provides component hierarchy related checks to a {@link BindingController}.
*
*
* Requirements
*
* - the component to which this controller object belongs must provide the
* {@link Component} and {@link SuperController} interfaces.
* - the type of the component to which this controller object belongs must be
* an instance of {@link org.objectweb.fractal.api.type.ComponentType}.
* - in order to be able to check bindings, this mixin needs the components to
* which this component is bound to provide interface introspection, and to
* provide the {@link SuperController} interface: if this component is bound to
* a component that does not satisfy these requirements, the binding will not be
* checked (and no error will be thrown).
*
*/
public abstract class ContentBindingMixin implements BindingController {
// -------------------------------------------------------------------------
// Private constructor
// -------------------------------------------------------------------------
private ContentBindingMixin () {
}
// -------------------------------------------------------------------------
// Fields and methods added and overriden by the mixin class
// -------------------------------------------------------------------------
/**
* Calls the {@link #checkFcLocalBinding checkFcLocalBinding} method and then
* calls the overriden method.
*
* @param clientItfType the type of the clientItfName interface.
* @param clientItfName the name of a client interface of the component to
* which this interface belongs.
* @param serverItf a server interface.
* @throws NoSuchInterfaceException if there is no such client interface.
* @throws IllegalBindingException if the binding cannot be created.
* @throws IllegalLifeCycleException if this component has a {@link
* org.objectweb.fractal.api.control.LifeCycleController} interface, but
* it is not in an appropriate state to perform this operation.
*/
public void bindFc (
final InterfaceType clientItfType,
final String clientItfName,
final Object serverItf)
throws
NoSuchInterfaceException,
IllegalBindingException,
IllegalLifeCycleException
{
checkFcLocalBinding(clientItfType, clientItfName, serverItf);
_super_bindFc(clientItfType, clientItfName, serverItf);
}
/**
* Checks that the given binding is a local binding.
*
* @param clientItfType the type of the clientItfName interface.
* @param clientItfName the name of a client interface.
* @param serverItf a server interface.
* @throws IllegalBindingException if the given binding is not a local
* binding.
*/
public void checkFcLocalBinding (
final InterfaceType clientItfType,
final String clientItfName,
final Object serverItf) throws IllegalBindingException
{
Interface sItf;
Component sComp;
try {
sItf = (Interface)serverItf;
sComp = sItf.getFcItfOwner();
} catch (ClassCastException e) {
// if the server interface does not provide interface introspection
// functions, the checks below cannot be performed
return;
}
Component[] cParents = _this_weaveableSC.getFcSuperComponents();
String msg;
if (!clientItfType.isFcClientItf()) {
// internal client interface
ContentController cc;
try {
cc = (ContentController)_this_weaveableC.
getFcInterface("content-controller");
} catch (NoSuchInterfaceException e) {
return;
}
// yes, check export binding:
// server component must be a sub component of client component...
Component[] cSubComps = cc.getFcSubComponents();
for (int i = 0; i < cSubComps.length; ++i) {
if (cSubComps[i].equals(sComp)) {
return;
}
}
// ...or equal to client component
Component thisComp;
try {
thisComp = (Component)_this_weaveableC.getFcInterface("component");
} catch (NoSuchInterfaceException e) {
throw new ChainedIllegalBindingException(
e, _this_weaveableC,
sItf.getFcItfOwner(),
clientItfName,
sItf.getFcItfName(),
"Cannot get the Component interface of the client component");
}
if (sComp.equals(thisComp) && sItf.isFcInternalItf()) {
return;
}
msg = "Invalid export binding";
} else if (sItf.isFcInternalItf()) {
// checks that the server component is a parent of the client component
for (int i = 0; i < cParents.length; ++i) {
if (sComp.equals(cParents[i])) {
return;
}
}
msg = "Invalid import binding";
} else {
SuperController sCompSC;
try {
sCompSC = (SuperController)sComp.getFcInterface("super-controller");
} catch (NoSuchInterfaceException e) {
// if the server component does not have a SuperController interface,
// the checks below cannot be performed
return;
}
Component[] sParents = sCompSC.getFcSuperComponents();
// checks that the client and server components have a common parent
for (int i = 0; i < cParents.length; ++i) {
for (int j = 0; j < sParents.length; ++j) {
if (cParents[i].equals(sParents[j])) {
return;
}
}
}
msg = "Not a local binding";
}
throw new ChainedIllegalBindingException(
null,
_this_weaveableC,
sItf.getFcItfOwner(),
clientItfName,
sItf.getFcItfName(),
msg);
}
// -------------------------------------------------------------------------
// Fields and methods required by the mixin class in the base class
// -------------------------------------------------------------------------
/**
* The weaveableC field required by this mixin. This field is
* supposed to reference the {@link Component} interface of the component to
* which this controller object belongs.
*/
public Component _this_weaveableC;
/**
* The weaveableSC field required by this mixin. This field is
* supposed to reference the {@link SuperController} interface of the
* component to which this controller object belongs.
*/
public SuperController _this_weaveableSC;
/**
* The {@link TypeBindingMixin#bindFc(InterfaceType,String,Object) bindFc}
* method overriden by this mixin.
*
* @param clientItfType the type of the clientItfName interface.
* @param clientItfName the name of a client interface of the component to
* which this interface belongs.
* @param serverItf a server interface.
* @throws NoSuchInterfaceException if there is no such client interface.
* @throws IllegalBindingException if the binding cannot be created.
* @throws IllegalLifeCycleException if this component has a {@link
* org.objectweb.fractal.api.control.LifeCycleController} interface, but
* it is not in an appropriate state to perform this operation.
*/
public abstract void _super_bindFc (
InterfaceType clientItfType,
String clientItfName,
Object serverItf)
throws
NoSuchInterfaceException,
IllegalBindingException,
IllegalLifeCycleException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy