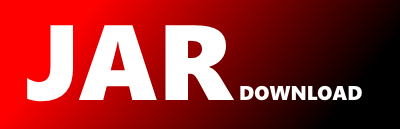
org.objectweb.fractal.julia.control.binding.OptimizedContainerBindingMixin Maven / Gradle / Ivy
The newest version!
/***
* Julia: France Telecom's implementation of the Fractal API
* Copyright (C) 2001-2011 France Telecom R&D
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* Contact: [email protected]
*
* Author: Eric Bruneton
*/
package org.objectweb.fractal.julia.control.binding;
import org.objectweb.fractal.api.control.BindingController;
import org.objectweb.fractal.api.control.IllegalBindingException;
import org.objectweb.fractal.api.control.IllegalLifeCycleException;
import org.objectweb.fractal.api.NoSuchInterfaceException;
import org.objectweb.fractal.julia.ComponentInterface;
import java.util.Map;
import java.util.HashMap;
/**
* Provides an optimized container based implementation of the {@link
* BindingController} interface. This mixin is designed to override a basic
* container binding mixin: indeed it implements the {@link BindingController}
* methods by using a hash map, and calls the overriden method to optimized
* bindings in the encapsulated component (the bindings are optimized by binding
* the encapsulated component to
* "((ComponentInterface)serverItf).getFcItfImpl()", instead of "serverItf").
*
*
* Requirements
*
* - TODO.
*
*/
public abstract class OptimizedContainerBindingMixin
implements BindingController
{
// -------------------------------------------------------------------------
// Private constructor
// -------------------------------------------------------------------------
private OptimizedContainerBindingMixin () {
}
// -------------------------------------------------------------------------
// Fields and methods added and overriden by the mixin class
// -------------------------------------------------------------------------
/**
* The map used to store the bindings of this component. This map associate
* server interface references to client interface names. A client interface
* name is associated with 'fcBindings' means that this interface is unbound.
*/
public Map fcBindings;
/**
* Returns the names of the client interfaces of the component to which this
* interface belongs. This method returns the fcBindings keys.
*
* @return the names of the client interfaces of the component to which this
* interface belongs.
*/
public String[] listFc () {
Map fcBindings = getFcBindings();
return (String[])fcBindings.keySet().toArray(new String[fcBindings.size()]);
}
/**
* Returns the interface to which the given client interface is bound. More
* precisely, returns the server interface to which the client interface whose
* name is given is bound. This server interface is necessarily in the same
* address space as the client interface (see {@link #bindFc bindFc}). This
* method uses the fcBindings map to return its result.
*
* @param clientItfName the name of a client interface of the component to
* which this interface belongs.
* @return the server interface to which the given interface is bound, or
* null if it is not bound.
* @throws NoSuchInterfaceException if the component to which this interface
* belongs does not have a client interface whose name is equal to the
* given name.
*/
public Object lookupFc (final String clientItfName)
throws NoSuchInterfaceException
{
Map fcBindings = getFcBindings();
Object result = fcBindings.get(clientItfName);
if (result == fcBindings) {
result = null;
}
return result;
}
/**
* Binds the client interface whose name is given to a server interface. More
* precisely, binds the client interface of the component to which this
* interface belongs, and whose name is equal to the given name, to the given
* server interface. The given server interface must be in the same address
* space as the client interface. This method updates the fcBindings map,
* and also called the overriden method, with serverItf.getFcItfImpl as second
* parameter, in order to save an indirection.
*
* @param clientItfName the name of a client interface of the component to
* which this interface belongs.
* @param serverItf a server interface.
* @throws NoSuchInterfaceException if there is no such client interface.
* @throws IllegalBindingException if the binding cannot be created.
* @throws IllegalLifeCycleException if this component has a {@link
* org.objectweb.fractal.api.control.LifeCycleController} interface, but it is not in an appropriate state
* to perform this operation.
*/
public void bindFc (final String clientItfName, final Object serverItf) throws
NoSuchInterfaceException,
IllegalBindingException,
IllegalLifeCycleException
{
Object o = serverItf;
if (o instanceof ComponentInterface) {
o = ((ComponentInterface)o).getFcItfImpl();
}
if (o != null) {
_super_bindFc(clientItfName, o);
}
Map fcBindings = getFcBindings();
fcBindings.put(clientItfName, serverItf);
}
/**
* Unbinds the given client interface. More precisely, unbinds the client
* interface of the component to which this interface belongs, and whose name
* is equal to the given name. This method updates the fcBindings map, and
* also calls the overriden method.
*
* @param clientItfName the name of a client interface of the component to
* which this interface belongs.
* @throws NoSuchInterfaceException if there is no such client interface.
* @throws IllegalBindingException if the binding cannot be removed.
* @throws IllegalLifeCycleException if this component has a {@link
* org.objectweb.fractal.api.control.LifeCycleController} interface, but it is not in an appropriate state
* to perform this operation.
*/
public void unbindFc (final String clientItfName) throws
NoSuchInterfaceException,
IllegalBindingException,
IllegalLifeCycleException
{
_super_unbindFc(clientItfName);
if (fcBindings != null) {
fcBindings.put(clientItfName, fcBindings);
}
}
/**
* Returns the fcBindings map. This map is created and initialized if
* necessary.
*
* @return the fcBindings map.
*/
private Map getFcBindings () {
if (fcBindings == null) {
fcBindings = new HashMap();
String[] names = _super_listFc();
for (int i = 0; i < names.length; ++i) {
fcBindings.put(names[i], fcBindings);
}
}
return fcBindings;
}
// -------------------------------------------------------------------------
// Fields and methods required by the mixin class in the base class
// -------------------------------------------------------------------------
/**
* The {@link BindingController#listFc listFc} method overriden by this
* mixin.
*
* @return the names of the client interfaces of the component to which this
* interface belongs.
*/
public abstract String[] _super_listFc ();
/**
* The {@link BindingController#lookupFc lookupFc} method overriden by this
* mixin.
*
* @param clientItfName the name of a client interface of the component to
* which this interface belongs.
* @return the server interface to which the given interface is bound, or
* null if it is not bound.
* @throws NoSuchInterfaceException if the component to which this interface
* belongs does not have a client interface whose name is equal to the
* given name.
*/
public abstract Object _super_lookupFc (String clientItfName) throws
NoSuchInterfaceException;
/**
* The {@link BindingController#bindFc bindFc} method overriden by this mixin.
*
* @param clientItfName the name of a client interface of the component to
* which this interface belongs.
* @param serverItf a server interface.
* @throws NoSuchInterfaceException if there is no such client interface.
* @throws IllegalBindingException if the binding cannot be created.
* @throws IllegalLifeCycleException if this component has a {@link
* org.objectweb.fractal.api.control.LifeCycleController} interface, but it is not in an appropriate state
* to perform this operation.
*/
public abstract void _super_bindFc (String clientItfName, Object serverItf) throws
NoSuchInterfaceException,
IllegalBindingException,
IllegalLifeCycleException;
/**
* The {@link BindingController#unbindFc unbindFc} method overriden by this
* mixin.
*
* @param clientItfName the name of a client interface of the component to
* which this interface belongs.
* @throws NoSuchInterfaceException if there is no such client interface.
* @throws IllegalBindingException if the binding cannot be removed.
* @throws IllegalLifeCycleException if this component has a {@link
* org.objectweb.fractal.api.control.LifeCycleController} interface, but it is not in an appropriate state
* to perform this operation.
*/
public abstract void _super_unbindFc (String clientItfName) throws
NoSuchInterfaceException,
IllegalBindingException,
IllegalLifeCycleException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy