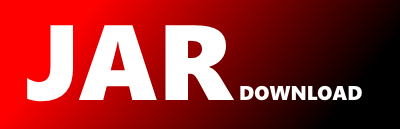
org.objectweb.fractal.mind.adl.ADLErrors Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of adl-frontend Show documentation
Show all versions of adl-frontend Show documentation
Front-end components for the ADL language of the Mind programming model.
The newest version!
/**
* Copyright (C) 2009 STMicroelectronics
*
* This file is part of "Mind Compiler" is free software: you can redistribute
* it and/or modify it under the terms of the GNU Lesser General Public License
* as published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more
* details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*
* Contact: [email protected]
*
* Authors: Matthieu Leclercq
* Contributors:
*/
package org.objectweb.fractal.mind.adl;
import static org.objectweb.fractal.adl.error.ErrorTemplateValidator.validErrorTemplate;
import org.objectweb.fractal.adl.error.ErrorTemplate;
/**
* Enumeration of Errors that can be raised by the ADL-front-end.
*/
public enum ADLErrors implements ErrorTemplate {
/** */
INVALID_DEFINITION_NAME("\"%s\" is not a valid definition name",
""),
/** */
INVALID_REFERENCE_NOT_A_TYPE(
"Invalid reference: %s does not refer to a type definition.",
""),
/** */
INVALID_REFERENCE_NOT_A_COMPOSITE(
"Invalid reference: %s does not refer to a composite.", ""),
/** */
INVALID_REFERENCE_NOT_A_PRIMITIVE(
"Invalid reference: %s does not refer to a primitive.", ""),
/** */
INVALID_REFERENCE_FOR_SUB_COMPONENT(
"Invalid reference: %s refer to a type or an abstract definition.",
""),
/** */
INVALID_SUB_COMPONENT("Invalid sub-component."),
/** */
INVALID_REFERENCE_MISSING_TEMPLATE_VALUE(
"Invalid reference: missing type arguments."),
/** */
INVALID_REFERENCE_TOO_MANY_TEMPLATE_VALUE(
"Invalid reference: too many type arguments."),
/** */
INVALID_REFERENCE_ANY_TEMPLATE_VALUE(
"Invalid reference: \"any\" type argument is not allowed here."),
/** */
INVALID_ANY_TEMPLATE_VALUE(
"Invalid reference: sub-component \"%s\" must be overridden or \"any\" type argument is not allowed here.",
""),
/** */
INVALID_REFERENCE_NO_SUCH_TEMPLATE_VARIABLE(
"Invalid reference: no such type parameter \"%s\".", ""),
/** */
INVALID_REFERENCE_NO_TEMPLATE_VARIABLE(
"Invalid reference: %s has no type parameter.", ""),
/** */
UNDEFINED_TEMPALTE_VARIABLE(
"Type parameter \"%s\" is not defined in current definition",
""),
/** */
DUPLICATED_TEMPALTE_VARIABLE_NAME("Duplicated type parameter \"%s\"",
""),
/** */
INVALID_TEMPLATE_VALUE_TYPE_DEFINITON(
"Invalid type argument: %s is a type or abstract definition.",
""),
/** */
INVALID_TEMPLATE_VALUE_MISSING_SERVER_INTERFACE(
"Invalid type argument: %s must provide a \"%s\" interface.",
"", ""),
/** */
INVALID_TEMPLATE_VALUE_MISSING_CLIENT_INTERFACE(
"Invalid type argument: %s must require a \"%s\" interface.",
"", ""),
/** */
INVALID_TEMPLATE_VALUE_CLIENT_INTERFACE_MUST_BE_OPTIONAL(
"Invalid type argument: required interface \"%s\" must be optional. Interface declared at %s",
"", ""),
/** */
DO_NOT_OVERRIDE("Declaration does not override an inherited declaration."),
/** */
INVALID_INTERFACE_NAME_OVERRIDE_INHERITED_INTERFACE(
"Invalid interface name, an interface with the same name already exist in inherited definition at %s",
""),
/** */
INVALID_ATTRIBUTE_NAME_OVERRIDE_INHERITED_ATTRIBUTE(
"Invalid attribute name, an attribute with the same name already exist in inherited definition at %s",
""),
/** */
DUPLICATED_ATTRIBUTE_NAME(
"Redefinition of attribute \"%s\" (previously defined at \"%s\").",
"", ""),
/** */
INVALID_PATH("Invalid Path \"%s\"", ""),
/** */
SOURCE_NOT_FOUND("Can't find source file \"%s\"", ""),
/** */
MISSING_SOURCE("Primitive component must have source"),
/** */
INVALID_REFERENCE_NO_PARAMETER(
"Invalid reference: referenced definition has no parameter."),
/** */
INVALID_REFERENCE_MISSING_ARGUMENT("Invalid reference: missing argument."),
/** */
INVALID_REFERENCE_TOO_MANY_ARGUMENT("Invalid reference: too many argument."),
/** */
INVALID_REFERENCE_NO_SUCH_PARAMETER(
"Invalid reference: no such parameter \"%s\".", ""),
/** */
UNDEFINED_PARAMETER("Parameter \"%s\" is not defined in current definition.",
""),
/** */
DUPLICATED_ARGUMENT_VARIABLE_NAME("Duplicated argument \"%s\"", ""),
/** */
INCOMPATIBLE_ARGUMENT_TYPE("Incompatible type for argument \"%s\".",
""),
/** */
INCOMPATIBLE_ARGUMENT_VALUE("Incompatible type for argument \"%s\".",
""),
/** */
INCOMPATIBLE_ATTRIBUTE_VALUE("Incompatible type value for attribute \"%s\".",
""),
/** */
INVALID_EXTENDS_TYPE_EXTENDS_PRIMITIVE(
"Type definition cannot extends the primitive defintion \"%s\"",
""),
/** */
INVALID_EXTENDS_TYPE_EXTENDS_COMPOSITE(
"Type definition cannot extends the composite defintion \"%s\"",
""),
/** */
INVALID_EXTENDS_PRIMITIVE_EXTENDS_COMPOSITE(
"Primitive definition cannot extends the composite defintion \"%s\"",
""),
/** */
INVALID_EXTENDS_COMPOSITE_EXTENDS_PRIMITIVE(
"Composite definition cannot extends the primitive defintion \"%s\"",
""),
/** */
INVALID_FACTORY_OF_SINGLETON(
"Cannot make a factory of a singleton definition"),
/** */
INVALID_FACTORY_OF_ABSTRACT("Cannot make a factory of an abstract definition"),
/** */
INVALID_FACTORY_OF_REFERENCED_SINGLETON(
"Cannot make a factory of this definition. Definition references the singleton definition \"%s\"",
""),
/** */
SINGLETON_WITH_DIFFERENT_NAME(
"Singleton definition must always be instantiated with the same name"),
/** */
INSTANTIATE_TYPE_DEFINIITON("Can't instantiate type definition \"%s\".",
""),
/** */
INSTANTIATE_TEMPLATE_DEFINIITON(
"Can't instantiate definition \"%s\", definition contains template variables.",
""),
/** */
INSTANTIATE_ARGUMENT_DEFINIITON(
"Can't instantiate definition \"%s\", definition contains arguments.",
""),
/** */
UNKNOWN_IMPORT("Unknown import."),
;
/** The groupId of ErrorTemplates defined in this enumeration. */
public static final String GROUP_ID = "MADL";
private int id;
private String format;
private ADLErrors(final String format, final Object... args) {
this.id = ordinal();
this.format = format;
assert validErrorTemplate(this, args);
}
public int getErrorId() {
return id;
}
public String getGroupId() {
return GROUP_ID;
}
public String getFormatedMessage(final Object... args) {
return String.format(format, args);
}
public String getFormat() {
return format;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy