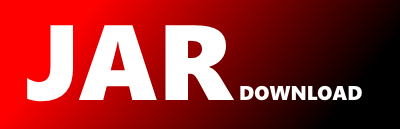
org.objectweb.fractal.mind.adl.generic.TemplateInstanceLoader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of adl-frontend Show documentation
Show all versions of adl-frontend Show documentation
Front-end components for the ADL language of the Mind programming model.
The newest version!
package org.objectweb.fractal.mind.adl.generic;
import static org.objectweb.fractal.mind.BindingControllerImplHelper.checkItfName;
import static org.objectweb.fractal.mind.BindingControllerImplHelper.listFcHelper;
import java.util.Map;
import org.objectweb.fractal.adl.ADLException;
import org.objectweb.fractal.adl.AbstractLoader;
import org.objectweb.fractal.adl.CompilerError;
import org.objectweb.fractal.adl.Definition;
import org.objectweb.fractal.adl.NodeFactory;
import org.objectweb.fractal.api.NoSuchInterfaceException;
import org.objectweb.fractal.api.control.IllegalBindingException;
import org.objectweb.fractal.mind.adl.DefinitionReferenceResolver;
import org.objectweb.fractal.mind.adl.ast.ASTHelper;
import org.objectweb.fractal.mind.adl.ast.DefinitionReference;
import org.objectweb.fractal.mind.adl.generic.DefinitionName.DefinitionNameArgument;
import org.objectweb.fractal.mind.adl.generic.ast.GenericASTHelper;
import org.objectweb.fractal.mind.adl.generic.ast.TypeArgument;
import org.objectweb.fractal.mind.adl.generic.ast.TypeArgumentContainer;
public class TemplateInstanceLoader extends AbstractLoader {
// ---------------------------------------------------------------------------
// Client interfaces
// ---------------------------------------------------------------------------
public static final String RECURSIVE_LOADER_ITF_NAME = "recursive-loader";
public DefinitionReferenceResolver definitionReferenceResolverItf;
public NodeFactory nodeFactoryItf;
// ---------------------------------------------------------------------------
// Implementation of the Loader interface
// ---------------------------------------------------------------------------
public Definition load(final String name, final Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy