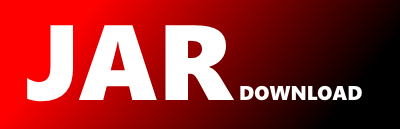
org.objectweb.fractal.mind.adl.parameter.ExtendsParametricDefinitionReferenceResolver Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of adl-frontend Show documentation
Show all versions of adl-frontend Show documentation
Front-end components for the ADL language of the Mind programming model.
The newest version!
/**
* Copyright (C) 2009 STMicroelectronics
*
* This file is part of "Mind Compiler" is free software: you can redistribute
* it and/or modify it under the terms of the GNU Lesser General Public License
* as published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more
* details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*
* Contact: [email protected]
*
* Authors: Matthieu Leclercq
* Contributors:
*/
package org.objectweb.fractal.mind.adl.parameter;
import static org.objectweb.fractal.adl.NodeUtil.cloneGraph;
import java.util.HashMap;
import java.util.Map;
import org.objectweb.fractal.adl.ADLException;
import org.objectweb.fractal.adl.Definition;
import org.objectweb.fractal.adl.NodeUtil;
import org.objectweb.fractal.mind.adl.AbstractDefinitionReferenceResolver;
import org.objectweb.fractal.mind.adl.DefinitionReferenceResolver;
import org.objectweb.fractal.mind.adl.ExtendsLoader;
import org.objectweb.fractal.mind.adl.SubComponentResolverLoader;
import org.objectweb.fractal.mind.adl.ast.DefinitionReference;
import org.objectweb.fractal.mind.adl.ast.Attribute;
import org.objectweb.fractal.mind.adl.ast.AttributeContainer;
import org.objectweb.fractal.mind.adl.ast.Component;
import org.objectweb.fractal.mind.adl.ast.ComponentContainer;
import org.objectweb.fractal.mind.adl.parameter.ast.Argument;
import org.objectweb.fractal.mind.adl.parameter.ast.ArgumentContainer;
import org.objectweb.fractal.mind.adl.parameter.ast.FormalParameter;
import org.objectweb.fractal.mind.adl.parameter.ast.FormalParameterContainer;
import org.objectweb.fractal.mind.value.ast.Reference;
import org.objectweb.fractal.mind.value.ast.Value;
/**
* This delegating {@link DefinitionReferenceResolver} replaces, in the resolved
* definition, every parameter occurrences by its corresponding value. This
* DefinitionReferenceResolver
should be used only by
* {@link ExtendsLoader} and should not be used by
* {@link SubComponentResolverLoader}.
*/
public class ExtendsParametricDefinitionReferenceResolver
extends
AbstractDefinitionReferenceResolver {
// ---------------------------------------------------------------------------
// Implementation of the DefinitionReferenceResolver interface
// ---------------------------------------------------------------------------
public Definition resolve(final DefinitionReference reference,
final Definition encapsulatingDefinition,
final Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy