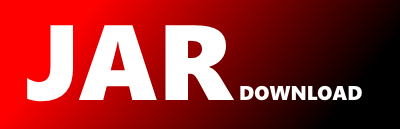
org.objectweb.jonas.common.JProp Maven / Gradle / Ivy
The newest version!
/**
* JOnAS: Java(TM) Open Application Server
* Copyright (C) 1999 Bull S.A.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA
*
* Initial developer(s): ____________________________________.
* Contributor(s): Adriana Danes
*
* --------------------------------------------------------------------------
* $Id: JProp.java 10822 2007-07-04 08:26:06Z durieuxp $
* --------------------------------------------------------------------------
*/
package org.objectweb.jonas.common;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.FileNotFoundException;
import java.io.FileWriter;
import java.io.IOException;
import java.util.Enumeration;
import java.util.Hashtable;
import java.util.Properties;
import java.util.StringTokenizer;
import javax.naming.Context;
/**
* This class manages configuration properties for a JOnAS Server.
* It adopts the singleton design-pattern. Configuration parameters
* are read from .properties file (jonas.properties or resource.properties).
* In order to support dynamically created resources, JProp also allows for
* a .properties file generation using a java.lang.Properties object content
* @author jonas-team
* @author Adriana Danes
* @author Florent Benoit
*
* -
* ../03/2003 Adriana Danes
*
* - Manage unique instances for resource.properties
*
- Replace 'config' with 'conf'
*
- Change initial configuration policy : read properties file in a sole location : JONAS_BASE/CONFIG_DIR/
*
- Replace method name
getFilesEnv
to getConfigFileEnv
*
* -
* 05/05/2003 Adriana Danes. Support JProp instance creation for dynamically created resources.
*
* 05/2003 Florent Benoit. Add support of xml files and checkstyle
*/
public class JProp {
/**
* File name where JOnAS components versions are stored.
*/
public static final String JONAS_VERSIONS = "VERSIONS";
/**
* Prefix for jonas.properties file
*/
public static final String JONASPREFIX = "jonas";
/**
* Domain name
*/
public static final String DOMAIN_NAME = "domain.name";
/**
* JOnAS server name
*/
public static final String JONAS_NAME = "jonas.name";
/**
* Default server name
*/
public static final String JONAS_DEF_NAME = "jonas";
/**
* -Djonas.base property
*/
public static final String JONAS_BASE = "jonas.base";
/**
* configuration directory name (changed from 'config' to 'conf' !!)
*/
private static final String CONFIG_DIR = "conf";
/**
* System properties
*/
private static Properties systEnv = System.getProperties();
/**
* JONAS_BASE
*/
private static String jonasBase = systEnv.getProperty(JONAS_BASE);
/**
* JONAS_ROOT
*/
private static String installRoot = systEnv.getProperty("install.root");
/**
* Separator of file
*/
private static String fileSeparator = systEnv.getProperty("file.separator");
/**
* Content of the file (xml)
*/
private String configFileXml = null;
/**
* Content of a text file (may be a .properties file
*/
private String versionFileContent = null;
/**
* Properties of the config file
*/
private Properties configFileEnv = new Properties();
/**
* System properties + properties of the file
*/
private Properties allEnv = null;
/**
* Name of the property file
*/
private String propFileName = null;
/**
* The JProp singleton for jonas.properties file
*/
private static JProp unique = null;
/**
* The multiple JProp objects for the resources configuration files (resource.properties)
* the keys are the configuration file names and the values, the JProp objects
*/
private static Hashtable multiple = new Hashtable();
/**
* Private constructor which reads a resource.properties file.
* @param fileName the file name to read (the resource name)
*/
private JProp(String fileName) {
readFile(fileName);
}
/**
* Private constructor which reads the JONAS properties file (jonas.properties).
*/
private JProp() {
readFile(JONASPREFIX);
}
/**
* private constructor which writes properties in the provided file.
* @param fileName the file name in which configuration properties are written
* @param props configuration properties used to initialize the resource
*/
private JProp(String fileName, Properties props) {
writePropsToFile(fileName, props);
}
/**
* private constructor which writes text in the provided file.
* @param fileName the file name in which configuration is written
* @param txt xml configuration for this resource
*/
private JProp(String fileName, String txt) {
writeXmlToFile(fileName, txt);
}
/**
* Get the unique instance corresponding to the JOnAS server.
* Create it at first call.
* @return unique instance corresponding to the JOnAS server.
*/
public static JProp getInstance() {
if (unique == null) {
unique = new JProp();
}
return unique;
}
/**
* Get one of the multiple instances corresponding to a given resource.
* Create it at first call with a given configuration file name
* @param fileName the name of the configuration file which is given by the resource name
* @return one of the multiple instances corresponding to a given resource.
*/
public static JProp getInstance(String fileName) {
if (!multiple.containsKey(fileName)) {
multiple.put(fileName, new JProp(fileName));
}
return (JProp) multiple.get(fileName);
}
/**
* Get one of the multiple instances corresponding to a given resource.
* Create it at first call with a given configuration file name
* @param fileName the name of the configuration file
* @param props the content of the configuration file to be written in fileName
* @return one of the multiple instances corresponding to a given resource.
*/
public static JProp getInstance(String fileName, Properties props) {
if (!multiple.containsKey(fileName)) {
multiple.put(fileName, new JProp(fileName, props));
}
return (JProp) multiple.get(fileName);
}
/**
* Remove the JProp instance corresponding to the given file name from the 'multiple'
* data structure
* @param fileName file name corresponding to the name of the resource to be removed
*/
public static void removeInstance(String fileName) {
if (multiple.containsKey(fileName)) {
multiple.remove(fileName);
}
}
/**
* Remove the JProp instance corresponding to the given file name from the 'multiple'
* data structure and delete the file
* @param fileName file name corresponding to the name of the resource to be removed
* and deleted from the file system
*/
public static void deleteInstance(String fileName) {
if (multiple.containsKey(fileName)) {
multiple.remove(fileName);
// Check the JONAS_BASE environment property
if (jonasBase == null) {
return;
}
jonasBase = jonasBase.trim();
// JONAS_BASE/conf/fileName
String propFileName = jonasBase + fileSeparator + CONFIG_DIR + fileSeparator + fileName + ".properties";
File del = new File(propFileName);
del.delete();
}
}
/**
* Write configuration properties in file getProperty(JONAS_BASE)/conf/fileName
* @param fileName name of the configuration file to write
* @param props Properties to register in the fileName file
* @throws Exception if it can't write to the specified file
*/
private void writePropsToFile(String fileName, Properties props) {
// Check the JONAS_BASE environment property
if (jonasBase == null) {
throw new RuntimeException("JOnAS configuration error: environment property jonas.base not set!");
}
jonasBase = jonasBase.trim();
// JONAS_BASE/conf/fileName
propFileName = jonasBase + fileSeparator + CONFIG_DIR + fileSeparator + fileName + ".properties";
try {
FileOutputStream os = new FileOutputStream(propFileName);
props.store(os, "This file is generated by JOnAS");
os.close();
} catch (FileNotFoundException e) {
// File propFileName could not be opened
propFileName = null;
throw new RuntimeException("Cannot write config file :" + e);
} catch (IOException e) {
throw new RuntimeException("Cannot write config file :" + e);
}
configFileEnv = (Properties) props.clone();
allEnv = configFileEnv;
}
/**
* Write xml configuration in file getProperty(JONAS_BASE)/conf/fileName
* @param fileName name of the configuration file to write
* @param txt text to write
* @throws Exception if it can't write to the specified file
*/
private void writeXmlToFile(String fileName, String txt) {
// Check the JONAS_BASE environment property
if (jonasBase == null) {
throw new RuntimeException("JOnAS configuration error: environment property jonas.base not set!");
}
jonasBase = jonasBase.trim();
// JONAS_BASE/conf/fileName
propFileName = jonasBase + fileSeparator + CONFIG_DIR + fileSeparator + fileName + ".properties";
try {
BufferedWriter out = new BufferedWriter(new FileWriter(new File(propFileName)));
out.write(txt);
out.flush();
out.close();
} catch (FileNotFoundException e) {
// File propFileName could not be opened
propFileName = null;
throw new RuntimeException("Cannot write xml configuration file:" + e);
} catch (IOException e) {
throw new RuntimeException("Cannot write xml configuration file:" + e);
}
}
/**
* Read the content of the specified file. It can be an xml or properties file
* @param fileName name of the properties configuration file to read
* @throws Exception if it fails
*/
private void readFile(String fileName) {
// Check the JONAS_BASE environment property
if (jonasBase == null) {
throw new RuntimeException("JOnAS configuration error: environment property jonas.base not set!");
}
jonasBase = jonasBase.trim();
if (fileName.equals(JONAS_VERSIONS)) {
readVersionFile();
return;
}
// JONAS_BASE/conf/fileName
String fileFullPathname = jonasBase + fileSeparator + CONFIG_DIR + fileSeparator + fileName;
if (fileFullPathname.toLowerCase().endsWith(".xml")) {
readXmlFile(fileFullPathname);
} else {
readPropsFile(fileFullPathname);
}
}
private void readVersionFile() {
String fileFullPathname = installRoot + fileSeparator + JONAS_VERSIONS;
try {
File f = new File(fileFullPathname);
int length = (int) f.length();
FileInputStream fis = new FileInputStream(f);
byte[] buffer = new byte[length];
fis.read(buffer);
fis.close();
versionFileContent = new String(buffer);
} catch (FileNotFoundException e) {
throw new RuntimeException("Cannot find file " + fileFullPathname);
} catch (IOException e) {
throw new RuntimeException("Cannot read file " + fileFullPathname);
}
}
/**
* Read initial configuration properties in file getProperty(JONAS_BASE)/conf/fileName
* These properties may be overridden by system properties, provided on the java command line.
* @param fileName name of the configuration file to read
* @throws Exception if it fails
*/
private void readPropsFile(String fileName) {
// Update filename of this JProp
this.propFileName = fileName;
if (!fileName.endsWith(".properties")) {
propFileName += ".properties";
}
File f = null;
try {
f = new File(propFileName);
FileInputStream is = new FileInputStream(f);
configFileEnv.load(is);
} catch (FileNotFoundException e) {
throw new RuntimeException("Cannot find properties for " + propFileName);
} catch (IOException e) {
throw new RuntimeException("Cannot load properties for " + propFileName);
}
allEnv = (Properties) configFileEnv.clone();
// Overriddes with syst properties
if (f.getName().equalsIgnoreCase("jonas.properties")) {
for (Enumeration e = systEnv.keys(); e.hasMoreElements();) {
Object key = e.nextElement();
String value = ((String) systEnv.get(key)).trim();
allEnv.put(key, (Object) value);
}
String serverName;
if (!systEnv.containsKey(JONAS_NAME)) {
allEnv.put(JONAS_NAME, JONAS_DEF_NAME);
}
serverName = ((String) allEnv.get(JONAS_NAME)).trim();
if (!allEnv.containsKey(DOMAIN_NAME) && !systEnv.containsKey(DOMAIN_NAME)) {
allEnv.put(DOMAIN_NAME, serverName);
}
}
}
/**
* Read initial configuration in file getProperty(JONAS_BASE)/conf/fileName
* @param fileName name of the xml configuration file to read
*/
private void readXmlFile(String fileName) {
// Update filename of this JProp
this.propFileName = fileName;
try {
File f = new File(propFileName);
int length = (int) f.length();
FileInputStream fis = new FileInputStream(f);
byte[] buffer = new byte[length];
fis.read(buffer);
fis.close();
configFileXml = new String(buffer);
} catch (FileNotFoundException e) {
throw new RuntimeException("Cannot find file " + propFileName);
} catch (IOException e) {
throw new RuntimeException("Cannot read file " + propFileName);
}
}
/**
* Static method which return the JOnAS install root value.
* @return the JOnAS install root value.
*/
public static String getInstallRoot() {
// Check install.root system property
if (installRoot == null) {
throw new RuntimeException("JOnAS configuration error: System property install.root not set!");
}
return installRoot;
}
/**
* Static method which return the jonas.base property
* @return the jonas.base property
*/
public static String getJonasBase() {
return jonasBase;
}
/**
* Static method which return the working directory in jonas.base
* @return the jonas.base property
*/
public static String getWorkDir() {
return jonasBase + File.separator + "work";
}
/**
* Static method which return the working directory in jonas.base
* @return the jonas.base property
*/
public static String getConfDir() {
return jonasBase + File.separator + CONFIG_DIR;
}
/**
* Returns properties filename
*
* @return JOnAS properties filename
*/
public String getPropFile() {
return propFileName;
}
/**
* Returns JOnAS environment as configured with configuration file properties content and
* system properties.
* @return JOnAS properties
*/
public Properties getEnv() {
return allEnv;
}
/**
* Returns JOnAS environment as configured with files properties only.
*
* @return JOnAS properties
*/
public Properties getConfigFileEnv() {
return configFileEnv;
}
/**
* Returns xml content of the resource file
*
* @return xml content of the resource file
*/
public String getConfigFileXml() {
return configFileXml;
}
public String getVersionFile() {
return versionFileContent;
}
/**
* Returns the value of the related property. With default values.
* @param key the search key
* @param defaultVal if the key is not found return this default value
* @return property value
*/
public String getValue(String key, String defaultVal) {
String retProperty = allEnv.getProperty(key, defaultVal);
return retProperty.trim();
}
/**
* Returns the value of the related property.
* The method returns null if the property is not found.
* @param key the wanted key
* @return property value, null if not exist
*/
public String getValue(String key) {
String retProperty = allEnv.getProperty(key);
if (retProperty != null) {
retProperty = retProperty.trim();
}
return retProperty;
}
/**
* Returns the value of the related property as boolean.
* @param key the wanted key
* @param def default run if not found
* @return property value, true or false.
*/
public boolean getValueAsBoolean(String key, boolean def) {
boolean ret = def;
String value = this.getValue(key);
if (value != null && value.equalsIgnoreCase("true")) {
ret = true;
}
return ret;
}
/**
* Returns the value of the related property as String [].
* The method returns null if the property is not found.
* @param key the wanted key
* @return property value, null if not exist
*/
public String[] getValueAsArray(String key) {
String [] res = null;
String value = this.getValue(key);
if (value != null) {
StringTokenizer st = new StringTokenizer(value, ",");
res = new String [st.countTokens()];
int i = 0;
while (st.hasMoreTokens()) {
res[i++] = st.nextToken().trim();
}
}
return res;
}
/**
* String representation of the object for trace purpose
* @return String representation of this object
*/
public String toString() {
String s = new String();
for (Enumeration e = this.configFileEnv.keys(); e.hasMoreElements();) {
Object key = e.nextElement();
Object value = this.configFileEnv.get(key);
s = s.concat(" " + key + " = " + value + "\n");
}
if (s.length() > 0) {
// take of the last '\n'
s = s.substring(0, s.length() - 1);
}
return s;
}
/**
* Bind all the properties found in file properties in a naming context
* the naming context must be allocated by the caller
* @param ctx given context for bindings properties
* @throws Exception if it fails
*/
public void env2Ctx(Context ctx) throws Exception {
Enumeration e = configFileEnv.propertyNames();
String key = null;
while (e.hasMoreElements()) {
key = (String) e.nextElement();
ctx.bind(key, configFileEnv.getProperty(key, ""));
}
}
/**
* Displays the JOnAS properties values, as they are set by the
* different property files.
* @param args the arguments for launching this program
*/
public static void main(String args[]) {
JProp jonasProperties = null;
try {
jonasProperties = JProp.getInstance();
} catch (Exception e) {
System.err.println(e);
System.exit(2);
}
for (Enumeration e = jonasProperties.configFileEnv.keys(); e.hasMoreElements();) {
Object key = e.nextElement();
Object value = jonasProperties.configFileEnv.get(key);
System.out.println(key.toString() + "=" + value.toString());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy