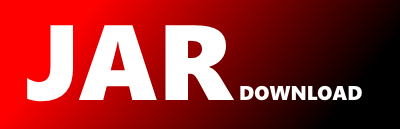
org.objectweb.jonas.server.Bootstrap Maven / Gradle / Ivy
/**
* JOnAS: Java(TM) Open Application Server
* Copyright (C) 1999-2004 Bull S.A.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA
*
* --------------------------------------------------------------------------
* $Id: Bootstrap.java 10822 2007-07-04 08:26:06Z durieuxp $
* --------------------------------------------------------------------------
*/
package org.objectweb.jonas.server;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import org.objectweb.jonas.common.JProp;
/**
* This class load all the jars needed to start JOnAS and after this, it launch
* the JOnAS server (Server class).
* @author Ludovic Bert (initial developer)
* @author Florent Benoit (initial developer)
* @author Philippe Durieux (new jonas jar files architecture)
*/
public class Bootstrap {
/**
* Server class name
*/
private static final String JONAS_SERVER_CLASS = "org.objectweb.jonas.server.Server";
/**
* Required JVM version
*/
private static final String REQUIRED_JVM_VERSION = "1.5";
/**
* Empty, private Constructor for Utility Class
*/
private Bootstrap() {
}
/**
* Server main routine (Load all the jars needed to start jonas)
* @param args the list of the args to give to the bootstrap class.
*/
public static void main(String[] args) {
String classToRun = args[0];
// JDK 1.4 or higher is required
String specVersion = System.getProperty("java.specification.version");
if (specVersion.compareTo(REQUIRED_JVM_VERSION) < 0) {
String implVersion = System.getProperty("java.vm.version");
System.err.println("A '" + REQUIRED_JVM_VERSION + "' JVM version or higher is required for JOnAS. Current JVM implementation version is '"
+ implVersion + "' with specification '" + specVersion + "'");
System.exit(0);
}
try {
LoaderManager lm = LoaderManager.getInstance();
lm.init(JProp.getInstance());
JClassLoader jonasLoader = null;
// TODO : Can specify classloader to use ?
if (classToRun.equals(JONAS_SERVER_CLASS)) {
jonasLoader = lm.getAppsLoader();
} else {
jonasLoader = lm.getToolsLoader();
}
Thread.currentThread().setContextClassLoader(jonasLoader);
//jonasLoader.printURLs(); // DEBUG
// Launch the "class_to_run" by using our classloader.
Class clazz = jonasLoader.loadClass(classToRun);
Class[] argList = new Class[] {args.getClass()};
Method meth = clazz.getMethod("main", argList);
String[] newArgs = new String[args.length - 1];
System.arraycopy(args, 1, newArgs, 0, newArgs.length);
meth.invoke(null, new Object[] {newArgs});
} catch (InvocationTargetException ite) {
Throwable t = ite.getTargetException();
String message = t.getMessage();
if (t instanceof Error) {
System.err.println("Error during execution of " + classToRun + " : " + message);
} else if (t instanceof Exception) {
System.err.println("Exception during execution of " + classToRun + " : " + message);
}
t.printStackTrace(System.err);
System.exit(2);
} catch (Exception e) {
System.err.println(classToRun + " reflection error : " + e);
e.printStackTrace();
System.exit(2);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy