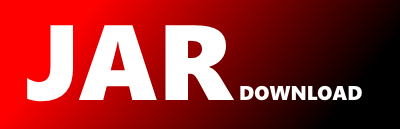
org.objectweb.jonas_client.deployment.xml.JonasClient Maven / Gradle / Ivy
/**
* JOnAS: Java(TM) Open Application Server
* Copyright (C) 1999 Bull S.A.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
*
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or 1any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA
*
* Initial developer: jonas-team
* --------------------------------------------------------------------------
* $Id: JonasClient.java 7467 2005-10-04 12:53:14Z sauthieg $
* --------------------------------------------------------------------------
*/
package org.objectweb.jonas_client.deployment.xml;
import org.objectweb.jonas_client.deployment.api.JonasAppClientSchemas;
import org.objectweb.jonas_lib.deployment.xml.AbsJonasEnvironmentElement;
import org.objectweb.jonas_lib.deployment.xml.JLinkedList;
import org.objectweb.jonas_lib.deployment.xml.JonasMessageDestination;
import org.objectweb.jonas_lib.deployment.xml.TopLevelElement;
/**
* This class defines the implementation of the element jonas-client
* @author jonas-team
*/
public class JonasClient extends AbsJonasEnvironmentElement implements TopLevelElement {
/**
* Header (with right XSD version) for XML
*/
private String header = null;
/**
* jonas-security
*/
private JonasSecurity jonasSecurity = null;
/**
* jonas-message-destination
*/
private JLinkedList jonasMessageDestinationList = null;
/**
* jonas-client element XML header
*/
public static final String JONAS_CLIENT_ELEMENT = "\n"
+ "\n";
/**
* Default constructor
*/
public JonasClient() {
super();
jonasMessageDestinationList = new JLinkedList("jonas-message-destination");
header = JONAS_CLIENT_ELEMENT;
}
/**
* @return the jonas-security
*/
public JonasSecurity getJonasSecurity() {
return jonasSecurity;
}
/**
* Set the jonas-security
* @param jonasSecurity jonasSecurity
*/
public void setJonasSecurity(JonasSecurity jonasSecurity) {
this.jonasSecurity = jonasSecurity;
}
/**
* @return the list of all jonas-message-destination elements
*/
public JLinkedList getJonasMessageDestinationList() {
return jonasMessageDestinationList;
}
/**
* Set the jonas-message-destination
* @param jonasMessageDestinationList jonasMessageDestination
*/
public void setJonasMessageDestinationList(JLinkedList jonasMessageDestinationList) {
this.jonasMessageDestinationList = jonasMessageDestinationList;
}
/**
* Add a new jonas-message-destination element to this object
* @param jonasMessageDestination the jonas-message-destination object
*/
public void addJonasMessageDestination(JonasMessageDestination jonasMessageDestination) {
jonasMessageDestinationList.add(jonasMessageDestination);
}
/**
* Represents this element by it's XML description.
* @param indent use this indent for prexifing XML representation.
* @return the XML description of this object.
*/
public String toXML(int indent) {
StringBuffer sb = new StringBuffer();
sb.append(indent(indent));
if (header != null) {
sb.append(header);
} else {
sb.append("\n");
}
indent += 2;
// jonas-ejb-ref
sb.append(getJonasEjbRefList().toXML(indent));
// jonas-resource
sb.append(getJonasResourceList().toXML(indent));
// jonas-resource-env
sb.append(getJonasResourceEnvList().toXML(indent));
// jonas-security
if (jonasSecurity != null) {
sb.append(jonasSecurity.toXML(indent));
}
// jonas-service-ref
sb.append(getJonasServiceRefList().toXML(indent));
// jonas-message-destination-ref
sb.append(getJonasMessageDestinationRefList().toXML(indent));
// jonas-message-destination
sb.append(jonasMessageDestinationList.toXML(indent));
indent -= 2;
sb.append(indent(indent));
sb.append(" \n");
return sb.toString();
}
/**
* @return the header.
*/
public String getHeader() {
return header;
}
/**
* @param header The header to set.
*/
public void setHeader(String header) {
this.header = header;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy