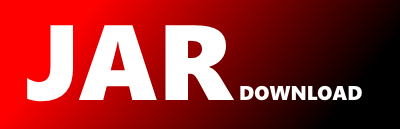
org.objectweb.jonas_domain.api.DomainMap Maven / Gradle / Ivy
/**
* JOnAS: Java(TM) Open Application Server
* Copyright (C) 2005 Bull S.A.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA
*
* --------------------------------------------------------------------------
* $Id: DomainMap.java 9256 2006-07-28 06:35:47Z durieuxp $
* --------------------------------------------------------------------------
*/
package org.objectweb.jonas_domain.api;
import java.util.Enumeration;
import java.util.Iterator;
import java.util.Vector;
import org.objectweb.jonas_domain.xml.Cluster;
import org.objectweb.jonas_domain.xml.ClusterDaemon;
import org.objectweb.jonas_domain.xml.Domain;
import org.objectweb.jonas_domain.xml.Server;
import org.objectweb.jonas_lib.deployment.api.AbsDeploymentDesc;
/**
* This class provides the map of the domain as described by the
* domain description file.
* It extends the AbsDeploymentDesc which requires to implement toString()
* and provides getSAXMsg() method (displayName is not used by DomainMap).
* @author Adriana Danes
*/
public class DomainMap extends AbsDeploymentDesc {
/**
* the domain name
*/
private String name = null;
/**
* the domain description
*/
private String description = null;
/**
* the clusterDaemons in the domain
*/
private Vector clusterDaemons = null;
/**
* the clusters in the domain
*/
private Vector clusters = null;
/**
* the servers in the domain
*/
private Vector servers = null;
/**
* @return Returns the clusterDaemons.
*/
public Vector getClusterDaemons() {
return clusterDaemons;
}
/**
* @param clusters The clusterDaemons to set.
*/
public void setClusterDaemons(Vector clusterDaemons) {
this.clusterDaemons = clusterDaemons;
}
/**
* @return Returns the clusters.
*/
public Vector getClusters() {
return clusters;
}
/**
* @param clusters The clusters to set.
*/
public void setClusters(Vector clusters) {
this.clusters = clusters;
}
/**
* @return Returns the description.
*/
public String getDescription() {
return description;
}
/**
* @param description The description to set.
*/
public void setDescription(String description) {
this.description = description;
}
/**
* @return Returns the name.
*/
public String getName() {
return name;
}
/**
* @param name The name to set.
*/
public void setName(String name) {
this.name = name;
}
/**
* @return Returns the servers.
*/
public Vector getServers() {
return servers;
}
/**
* @param servers The servers to set.
*/
public void setServers(Vector servers) {
this.servers = servers;
}
/**
* Constructor
* @param domain domain
*/
public DomainMap(Domain domain) {
name = domain.getName();
description = domain.getDescription();
clusterDaemons = new Vector();
clusters = new Vector();
servers = new Vector();
for (Iterator i = domain.getClusterDaemonList().iterator(); i.hasNext();) {
ClusterDaemon clusterDaemon = (ClusterDaemon) i.next();
clusterDaemons.add(clusterDaemon);
}
for (Iterator i = domain.getClusterList().iterator(); i.hasNext();) {
Cluster cluster = (Cluster) i.next();
clusters.add(cluster);
}
for (Iterator i = domain.getServerList().iterator(); i.hasNext();) {
Server server = (Server) i.next();
servers.add(server);
}
}
/**
* Return a String representation of the DomainMap
* @return a String representation of the DomainMap
*/
public String toString() {
StringBuffer ret = new StringBuffer();
ret.append("\nname=" + name);
ret.append("\ndescription=" + description);
ret.append("\nclusterDaemons=");
for (Enumeration e = clusterDaemons.elements(); e.hasMoreElements();) {
ret.append(e.nextElement() + ",");
}
ret.append("\nservers=");
for (Enumeration e = servers.elements(); e.hasMoreElements();) {
ret.append(e.nextElement() + ",");
}
ret.append("\nclusters=");
for (Enumeration e = clusters.elements(); e.hasMoreElements();) {
ret.append(e.nextElement() + ",");
}
return ret.toString();
}
}