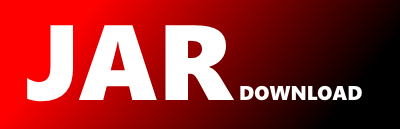
org.objectweb.jonas_ejb.deployment.api.EntityJdbcCmp1Desc Maven / Gradle / Ivy
The newest version!
/**
* JOnAS: Java(TM) Open Application Server
* Copyright (C) 1999-2004 Bull S.A.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA
*
* --------------------------------------------------------------------------
* $Id: EntityJdbcCmp1Desc.java 10290 2007-04-25 16:11:47Z durieuxp $
* --------------------------------------------------------------------------
*/
package org.objectweb.jonas_ejb.deployment.api;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.util.Iterator;
import org.objectweb.jonas_ejb.deployment.xml.AssemblyDescriptor;
import org.objectweb.jonas_ejb.deployment.xml.CmpFieldJdbcMapping;
import org.objectweb.jonas_ejb.deployment.xml.Entity;
import org.objectweb.jonas_ejb.deployment.xml.FinderMethodJdbcMapping;
import org.objectweb.jonas_ejb.deployment.xml.JonasEntity;
import org.objectweb.jonas_ejb.deployment.xml.JdbcMapping;
import org.objectweb.jonas_lib.deployment.xml.JLinkedList;
import org.objectweb.jonas_ejb.deployment.xml.JonasMethod;
import org.objectweb.jonas_lib.deployment.api.DeploymentDescException;
/**
* Class to hold meta-information related to an CMP v1 entity bean with jdbc data store.
* @author Christophe Ney [[email protected]] : Initial developer
* @author Helene Joanin
*/
// TODO : fill mapping information with defaults values (same as CMP 2)
// to accept to have optional mapping information in the jonas-ejb-jar.xml.
// TODO : Review this class, many methods are common with EntityJdbcCmp2yDesc
public class EntityJdbcCmp1Desc extends EntityCmp1Desc {
String jdbcTableName;
String datasourceJndiName;
/**
* constructor: called when the DeploymentDescriptor is read.
* Currently, called by both GenIC and createContainer.
*/
public EntityJdbcCmp1Desc(ClassLoader classLoader, Entity ent,
AssemblyDescriptor asd, JonasEntity jEnt,
JLinkedList jMDRList, String fileName)
throws DeploymentDescException {
super(classLoader, ent, asd, jEnt, jMDRList, fileName);
// check for jdbcMapping
JdbcMapping jm = jEnt.getJdbcMapping();
if (jm == null) {
throw new DeploymentDescException("jdbc-mapping missing for bean " + ent.getEjbName());
}
// populate field descriptor map with jdbc information
for (Iterator i = jm.getCmpFieldJdbcMappingList().iterator(); i.hasNext(); ) {
CmpFieldJdbcMapping cmpFieldJdbcMapping = (CmpFieldJdbcMapping) i.next();
String fn = cmpFieldJdbcMapping.getFieldName();
FieldDesc f = (FieldDesc) fieldDesc.get(fn);
if (f == null) {
throw new DeploymentDescException("field-name " + fn
+ " listed in cmp-field-jdbc-mapping is not of cmp-field of bean " + ent.getEjbName());
}
((FieldJdbcDesc) f).setJdbcFieldName(cmpFieldJdbcMapping.getJdbcFieldName());
}
// check that all cmp fields are jdbc
for (Iterator j = fieldDesc.values().iterator(); j.hasNext();) {
FieldJdbcDesc fd = (FieldJdbcDesc) j.next();
if (fd.getJdbcFieldName() == null) {
throw new DeploymentDescException("field-name " + fd.getName()
+ " is missing in cmp-field-jdbc-mapping for bean " + ent.getEjbName());
}
}
// populate method descriptor map with jdbc information
for (Iterator i = jm.getFinderMethodJdbcMappingList().iterator(); i.hasNext(); ) {
FinderMethodJdbcMapping fmj = ((FinderMethodJdbcMapping) i.next());
JonasMethod m = fmj.getJonasMethod();
for (Iterator j = getMethodDescIterator(); j.hasNext();) {
MethodJdbcCmp1Desc md = (MethodJdbcCmp1Desc) j.next();
int matchStatus = md.matchPattern(null, m.getMethodName(), m.getMethodParams());
md.overwriteJdbcWhereClause(fmj.getJdbcWhereClause(), matchStatus);
}
}
// jndi name of the datasource
datasourceJndiName = jm.getJndiName();
// table name
jdbcTableName = jm.getJdbcTableName();
// optionnal parameter automatic-pk
if (jm.getAutomaticPk() != null) {
jdbcAutomaticPk = jm.getAutomaticPk().equalsIgnoreCase("true");
}
}
/**
* Get the datasource jndi name
* @return String representation of the jndi name
*/
public String getDatasourceJndiName() {
return datasourceJndiName;
}
/**
* Get jdbc specific descriptor for a given field.
* Used by GenIC
* @param field of the bean class
* @return Descriptor for the given field
*/
public FieldJdbcDesc getFieldJdbcDesc(Field field) {
return (FieldJdbcDesc) super.getCmpFieldDesc(field);
}
/**
* Get the associated DataBase table name in case of container persistence type.
* Used by GenIC (This information is JOnAS specific).
* @return Name of the database table where entity bean is stored
*/
public String getJdbcTableName() {
return jdbcTableName;
}
/**
* factory method for method descriptors
*/
protected MethodDesc newMethodDescInstance(Method meth, Class classDef, int index) {
return new MethodJdbcCmp1Desc(this, meth, classDef, index);
}
/**
* String representation of the object for test purpose
* @return String representation of this object
*/
public String toString() {
StringBuffer ret = new StringBuffer();
ret.append(super.toString());
ret.append("\ngetDatasourceJndiName()=" + getDatasourceJndiName());
ret.append("\ngetJdbcTableName()=" + getJdbcTableName());
return ret.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy