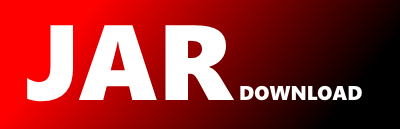
org.objectweb.jonas_ejb.deployment.api.EntityJdbcCmp2Desc Maven / Gradle / Ivy
The newest version!
/**
* JOnAS: Java(TM) Open Application Server
* Copyright (C) 1999-2004 Bull S.A.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA
*
* --------------------------------------------------------------------------
* $Id: EntityJdbcCmp2Desc.java 10290 2007-04-25 16:11:47Z durieuxp $
* --------------------------------------------------------------------------
*/
package org.objectweb.jonas_ejb.deployment.api;
import java.util.Iterator;
import org.objectweb.jonas_ejb.deployment.xml.*;
import org.objectweb.jonas_lib.deployment.api.DeploymentDescException;
import org.objectweb.jonas_lib.deployment.xml.JLinkedList;
/**
* Class to hold meta-information related to an CMP v2 entity bean with jdbc data store.
* @author Christophe Ney [[email protected]] : Initial developer
* @author Helene Joanin on May 2003: code cleanup
* @author Helene Joanin on May 2003: complement for legacy first version
*/
// TODO : Review this class, many methods are common with EntityJdbcCmp1Desc
public class EntityJdbcCmp2Desc extends EntityCmp2Desc {
protected String dsname;
protected String jdbcTableName = null;
/**
* constructor: called when the DeploymentDescriptor is read.
* Currently, called by both GenIC and createContainer.
*/
public EntityJdbcCmp2Desc(ClassLoader classLoader,
Entity ent,
AssemblyDescriptor asd,
JonasEntity jEnt,
DeploymentDescEjb2 dc2d,
JLinkedList jMDRList,
String fileName)
throws DeploymentDescException {
super(classLoader, ent, asd, jEnt, dc2d, jMDRList, fileName);
// check for jdbcMapping
JdbcMapping jm = jEnt.getJdbcMapping();
if (jm == null) {
throw new DeploymentDescException("jdbc-mapping missing for bean " + ent.getEjbName());
}
// jndi name of the datasource
dsname = jm.getJndiName();
// jdbc table name
if (jm.getJdbcTableName() != null) {
if (jm.getJdbcTableName().length() != 0) {
jdbcTableName = jm.getJdbcTableName();
}
}
if (jdbcTableName == null) {
// Default value
jdbcTableName = getAbstractSchemaName().toUpperCase() + "_";
}
// Default mapping information for cmp fields
for (Iterator i = fieldDesc.keySet().iterator();i.hasNext();) {
String fn = (String) i.next();
((FieldJdbcDesc)(fieldDesc.get(fn))).setJdbcFieldName(fn + "_");
}
// mapping information for cmp fields from jonas DD
for (Iterator i = jm.getCmpFieldJdbcMappingList().iterator();i.hasNext();) {
CmpFieldJdbcMapping fm = (CmpFieldJdbcMapping) i.next();
String fn = fm.getFieldName();
String cn = fm.getJdbcFieldName();
String ct = null;
if (fm.getSqlType() != null) {
ct = fm.getSqlType();
}
FieldJdbcDesc fdesc = (FieldJdbcDesc) fieldDesc.get(fn);
if (fdesc == null) {
throw new DeploymentDescException("field-name " + fn
+ " listed in cmp-field-jdbc-mapping is not of cmp-field of bean " + ent.getEjbName());
}
fdesc.setJdbcFieldName(cn);
if (ct != null) {
fdesc.setSqlType(ct);
}
}
// Specific mapping for primary key auto generated (type = java.lang.Object) if tag is specified
if (isUndefinedPK() && this.getJdbcAutomaticPkFieldName()!= null) {
((FieldJdbcDesc)(fieldDesc.get("JONASAUTOPKFIELD"))).setJdbcFieldName(this.getJdbcAutomaticPkFieldName());
}
}
/**
* field descriptor factory method
*/
protected FieldDesc newFieldDescInstance() {
return new FieldJdbcDesc();
}
/**
* Get the datasource jndi name
* @return String representation of the jndi name
*/
public String getDatasourceJndiName() {
return dsname;
}
/**
* Get the associated DataBase table name.
* @return Name of the database table where entity bean is stored
*/
public String getJdbcTableName() {
return jdbcTableName;
}
/**
* String representation of the object for test purpose
* @return String representation of this object
*/
public String toString() {
StringBuffer ret = new StringBuffer();
ret.append(super.toString());
ret.append("\ngetDatasourceJndiName()=" + getDatasourceJndiName());
ret.append("\ngetJdbcTableName()=" + getJdbcTableName());
return ret.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy