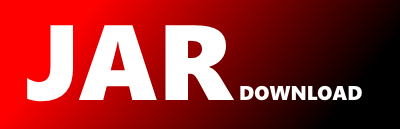
org.objectweb.jonas_ejb.deployment.xml.JonasEntity Maven / Gradle / Ivy
/**
* JOnAS: Java(TM) Open Application Server
* Copyright (C) 1999 Bull S.A.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
*
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or 1any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA
*
* Initial developer: JOnAS team
* --------------------------------------------------------------------------
* $Id: JonasEntity.java 10290 2007-04-25 16:11:47Z durieuxp $
* --------------------------------------------------------------------------
*/
package org.objectweb.jonas_ejb.deployment.xml;
/**
* This class defines the implementation of the element jonas-entity
*
* @author JOnAS team
*/
public class JonasEntity extends JonasCommonEjb {
/**
* is-modified-method-name
*/
private String isModifiedMethodName = null;
/**
* passivation-timeout
*/
private String passivationTimeout = null;
/**
* inactivity-timeout
*/
private String inactivityTimeout = null;
/**
* deadlock-timeout
*/
private String deadlockTimeout = null;
/**
* read-timeout
*/
private String readTimeout = null;
/**
* max-wait-time
*/
private String maxWaitTime = null;
/**
* shared
*/
private String shared = null;
/**
* prefetch
*/
private String prefetch = null;
/**
* hard-limit
*/
private String hardLimit = null;
/**
* cleanup
*/
private String cleanup = null;
/**
* lock policy
*/
private String lockPolicy = null;
/**
* jdbc-mapping
*/
private JdbcMapping jdbcMapping = null;
/**
* Constructor
*/
public JonasEntity() {
super();
}
/**
* Gets the is-modified-method-name
* @return the is-modified-method-name
*/
public String getIsModifiedMethodName() {
return isModifiedMethodName;
}
/**
* Set the is-modified-method-name
* @param isModifiedMethodName isModifiedMethodName
*/
public void setIsModifiedMethodName(String isModifiedMethodName) {
this.isModifiedMethodName = isModifiedMethodName;
}
/**
* Gets the passivation-timeout
* @return the passivation-timeout
*/
public String getPassivationTimeout() {
return passivationTimeout;
}
/**
* Set the passivation-timeout
* @param passivationTimeout passivationTimeout
*/
public void setPassivationTimeout(String passivationTimeout) {
this.passivationTimeout = passivationTimeout;
}
/**
* Gets the inactivity-timeout
* @return the inactivity-timeout
*/
public String getInactivityTimeout() {
return inactivityTimeout;
}
/**
* Set the inactivity-timeout
* @param inactivityTimeout inactivityTimeout
*/
public void setInactivityTimeout(String inactivityTimeout) {
this.inactivityTimeout = inactivityTimeout;
}
/**
* Set the deadlock-timeout
* @param deadlockTimeout deadlockTimeout
*/
public void setDeadlockTimeout(String deadlockTimeout) {
this.deadlockTimeout = deadlockTimeout;
}
/**
* Gets the deadlock-timeout
* @return the deadlock-timeout
*/
public String getDeadlockTimeout() {
return deadlockTimeout;
}
/**
* Gets the read-timeout
* @return the read-timeout
*/
public String getReadTimeout() {
return readTimeout;
}
/**
* Set the read-timeout
* @param readTimeout readTimeout
*/
public void setReadTimeout(String readTimeout) {
this.readTimeout = readTimeout;
}
/**
* Gets the max-wait-time
* @return the max-wait-time
*/
public String getMaxWaitTime() {
return maxWaitTime;
}
/**
* Set the max-wait-time
* @param maxWaitTime max wait time in seconds
*/
public void setMaxWaitTime(String maxWaitTime) {
this.maxWaitTime = maxWaitTime;
}
/**
* Gets the shared
* @return the shared
*/
public String getShared() {
return shared;
}
/**
* Set the shared
* @param shared shared
*/
public void setShared(String shared) {
this.shared = shared;
}
/**
* Gets the prefetch
* @return the prefetch
*/
public String getPrefetch() {
return prefetch;
}
/**
* Set the prefetch
* @param prefetch prefetch
*/
public void setPrefetch(String prefetch) {
this.prefetch = prefetch;
}
/**
* Gets the hard-limit
* @return the hard limit flag
*/
public String getHardLimit() {
return hardLimit;
}
/**
* Set the hard-limit flag
* @param hardLimit The hard limit flag
*/
public void setHardLimit(String hardLimit) {
this.hardLimit = hardLimit;
}
/**
* Gets the cleanup
* @return the cleanup
*/
public String getCleanup() {
return cleanup;
}
/**
* Set the cleanup
* @param cleanup cleanup
*/
public void setCleanup(String cleanup) {
this.cleanup = cleanup;
}
/**
* Gets the lock Policy
* @return the lockPolicy
*/
public String getLockPolicy() {
return lockPolicy;
}
/**
* Set the lock Policy
* @param lockPolicy lock Policy
*/
public void setLockPolicy(String lockPolicy) {
this.lockPolicy = lockPolicy;
}
/**
* Gets the jdbc-mapping
* @return the jdbc-mapping
*/
public JdbcMapping getJdbcMapping() {
return jdbcMapping;
}
/**
* Set the jdbc-mapping
* @param jdbcMapping jdbcMapping
*/
public void setJdbcMapping(JdbcMapping jdbcMapping) {
this.jdbcMapping = jdbcMapping;
}
/**
* Represents this element by it's XML description.
* @param indent use this indent for prexifing XML representation.
* @return the XML description of this object.
*/
public String toXML(int indent) {
StringBuffer sb = new StringBuffer();
sb.append(indent(indent));
sb.append("\n");
indent += 2;
// ejb-name
sb.append(xmlElement(getEjbName(), "ejb-name", indent));
// jndi-name
sb.append(xmlElement(getJndiName(), "jndi-name", indent));
// jonas-ejb-ref
sb.append(getJonasEjbRefList().toXML(indent));
// jonas-resource
sb.append(getJonasResourceList().toXML(indent));
// jonas-resource-env
sb.append(getJonasResourceEnvList().toXML(indent));
// jonas-service-ref
sb.append(getJonasServiceRefList().toXML(indent));
// jonas-message-destination-ref
sb.append(getJonasMessageDestinationRefList().toXML(indent));
// is-modified-method-name
if (isModifiedMethodName != null && !isModifiedMethodName.equals("")) {
sb.append(xmlElement(isModifiedMethodName, "is-modified-method-name", indent));
}
// passivation-timeout
if (passivationTimeout != null && !passivationTimeout.equals("")) {
sb.append(xmlElement(passivationTimeout, "passivation-timeout", indent));
}
// inactivity-timeout
if (inactivityTimeout != null && !inactivityTimeout.equals("")) {
sb.append(xmlElement(inactivityTimeout, "inactivity-timeout", indent));
}
// deadlock-timeout
if (deadlockTimeout != null && !deadlockTimeout.equals("")) {
sb.append(xmlElement(deadlockTimeout, "deadlock-timeout", indent));
}
// read-timeout
if (readTimeout != null && !readTimeout.equals("")) {
sb.append(xmlElement(readTimeout, "read-timeout", indent));
}
// max-wait-time
if (maxWaitTime != null && !maxWaitTime.equals("")) {
sb.append(xmlElement(maxWaitTime, "max-wait-time", indent));
}
// shared
if (shared != null && !shared.equals("")) {
sb.append(xmlElement(shared, "shared", indent));
}
// prefetch
if (prefetch != null && !prefetch.equals("")) {
sb.append(xmlElement(prefetch, "prefetch", indent));
}
// hard-limit
if (hardLimit != null && !hardLimit.equals("")) {
sb.append(xmlElement(hardLimit, "hard-limit", indent));
}
// max-cache-size
if (getMaxCacheSize() != null && !getMaxCacheSize().equals("")) {
sb.append(xmlElement(getMaxCacheSize(), "max-cache-size", indent));
}
// min-pool-size
if (getMinPoolSize() != null && !getMinPoolSize().equals("")) {
sb.append(xmlElement(getMinPoolSize(), "min-pool-size", indent));
}
// cleanup
if (cleanup != null && !cleanup.equals("")) {
sb.append(xmlElement(cleanup, "cleanup", indent));
}
// lock policy
if (lockPolicy != null && !lockPolicy.equals("")) {
sb.append(xmlElement(lockPolicy, "lock-policy", indent));
}
// jdbc-mapping
if (jdbcMapping != null && !jdbcMapping.equals("")) {
sb.append(jdbcMapping.toXML(indent));
}
// run-as
if (getRunAsPrincipalName() != null) {
sb.append(indent(indent));
sb.append("\n");
indent += 2;
sb.append(xmlElement(getRunAsPrincipalName(), "principal-name", indent));
indent -= 2;
sb.append(indent(indent));
sb.append(" \n");
}
// ior-security-config
if (getIorSecurityConfig() != null) {
sb.append(getIorSecurityConfig().toXML(indent));
}
indent -= 2;
sb.append(indent(indent));
sb.append(" \n");
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy