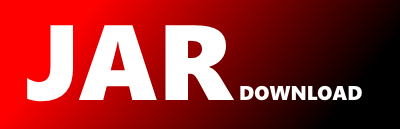
org.objectweb.jonas_lib.deployment.xml.AbsEnvironmentElement Maven / Gradle / Ivy
/**
* JOnAS: Java(TM) Open Application Server
* Copyright (C) 1999 Bull S.A.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or 1any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA
*
* Initial developer: Florent BENOIT
* --------------------------------------------------------------------------
* $Id: AbsEnvironmentElement.java 10290 2007-04-25 16:11:47Z durieuxp $
* --------------------------------------------------------------------------
*/
package org.objectweb.jonas_lib.deployment.xml;
/**
* This class defines an abstract implementation for all environment element
*
* Entity, Session, WebApp, Application-client elements must extend this class
* @author Florent Benoit
*/
public abstract class AbsEnvironmentElement extends AbsDescriptionElement implements JndiEnvRefsGroupXml {
/**
* List of ejb-local-ref
*/
private JLinkedList ejbLocalRefList = null;
/**
* List of ejb-ref
*/
private JLinkedList ejbRefList = null;
/**
* List of env-entry
*/
private JLinkedList envEntryList = null;
/**
* List of resource-env-ref
*/
private JLinkedList resourceEnvRefList = null;
/**
* List of resource-ref
*/
private JLinkedList resourceRefList = null;
/**
* List of service-ref
*/
private JLinkedList serviceRefList = null;
/**
* List of message-destination-ref
*/
private JLinkedList messageDestinationRefList = null;
/**
* Constructor : build a new object for environment
*/
public AbsEnvironmentElement() {
super();
ejbLocalRefList = new JLinkedList("ejb-local-ref");
ejbRefList = new JLinkedList("ejb-ref");
envEntryList = new JLinkedList("env-entry");
resourceEnvRefList = new JLinkedList("resource-env-ref");
resourceRefList = new JLinkedList("resource-ref");
serviceRefList = new JLinkedList("service-ref");
messageDestinationRefList = new JLinkedList("message-destination-ref");
}
// Setters
/**
* Add a new ejb-local-ref element to this object
* @param ejbLocalRef the ejb-local-ref object
*/
public void addEjbLocalRef(EjbLocalRef ejbLocalRef) {
ejbLocalRefList.add(ejbLocalRef);
}
/**
* Add a new ejb-ref element to this object
* @param ejbRef the ejb-ref object
*/
public void addEjbRef(EjbRef ejbRef) {
ejbRefList.add(ejbRef);
}
/**
* Add a new env-entry element to this object
* @param envEntry the ejb-ref object
*/
public void addEnvEntry(EnvEntry envEntry) {
envEntryList.add(envEntry);
}
/**
* Add a new resource-env-ref element to this object
* @param resourceEnvRef the resource-env-ref object
*/
public void addResourceEnvRef(ResourceEnvRef resourceEnvRef) {
resourceEnvRefList.add(resourceEnvRef);
}
/**
* Add a new resource-ref element to this object
* @param resourceRef the resource-ref object
*/
public void addResourceRef(ResourceRef resourceRef) {
resourceRefList.add(resourceRef);
}
/**
* Add a new service-ref element to this object
* @param serviceRef the service-ref object
*/
public void addServiceRef(ServiceRef serviceRef) {
serviceRefList.add(serviceRef);
}
/**
* Add a new message-destination-ref element to this object
* @param messageDestinationRef the message-destination-ref object
*/
public void addMessageDestinationRef(MessageDestinationRef messageDestinationRef) {
messageDestinationRefList.add(messageDestinationRef);
}
// Getters
/**
* @return the list of all ejb-local-ref elements
*/
public JLinkedList getEjbLocalRefList() {
return ejbLocalRefList;
}
/**
* @return the list of all ejb-ref elements
*/
public JLinkedList getEjbRefList() {
return ejbRefList;
}
/**
* @return the list of all env-entry elements
*/
public JLinkedList getEnvEntryList() {
return envEntryList;
}
/**
* @return the list of all resource-env-ref elements
*/
public JLinkedList getResourceEnvRefList() {
return resourceEnvRefList;
}
/**
* @return the list of all resource-ref elements
*/
public JLinkedList getResourceRefList() {
return resourceRefList;
}
/**
* @return the list of all service-ref elements
*/
public JLinkedList getServiceRefList() {
return serviceRefList;
}
/**
* @return the list of all messageDestination-ref elements
*/
public JLinkedList getMessageDestinationRefList() {
return messageDestinationRefList;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy