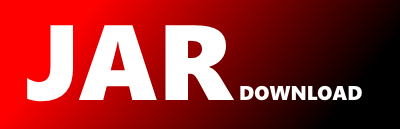
org.objectweb.jonas_rar.deployment.xml.PoolParams Maven / Gradle / Ivy
/**
* JOnAS: Java(TM) Open Application Server
* Copyright (C) 1999 Bull S.A.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
*
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or 1any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA
*
* Initial developer: Florent BENOIT
* --------------------------------------------------------------------------
* $Id: PoolParams.java 5701 2004-11-02 00:24:44Z ehardesty $
* --------------------------------------------------------------------------
*/
package org.objectweb.jonas_rar.deployment.xml;
import org.objectweb.jonas_lib.deployment.xml.AbsElement;
/**
* This class defines the implementation of the element pool-params
*
* @author Florent Benoit
*/
public class PoolParams extends AbsElement {
/**
* pool-init
*/
private String poolInit = null;
/**
* pool-min
*/
private String poolMin = null;
/**
* pool-max
*/
private String poolMax = null;
/**
* pool-max-age
*/
private String poolMaxAge = null;
/**
* pstmt-max
*/
private String pstmtMax = null;
/**
* pool-max-age-minutes
*/
private String poolMaxAgeMinutes = null;
/**
* pool-max-opentime
*/
private String poolMaxOpentime = null;
/**
* pool-max-waiters
*/
private String poolMaxWaiters = null;
/**
* pool-max-waittime
*/
private String poolMaxWaittime = null;
/**
* pool-sampling-period
*/
private String poolSamplingPeriod = null;
/**
* Constructor
*/
public PoolParams() {
super();
}
/**
* Gets the pool-init
* @return the pool-init
*/
public String getPoolInit() {
return poolInit;
}
/**
* Set the pool-init
* @param poolInit poolInit
*/
public void setPoolInit(String poolInit) {
this.poolInit = poolInit;
}
/**
* Gets the pool-min
* @return the pool-min
*/
public String getPoolMin() {
return poolMin;
}
/**
* Set the pool-min
* @param poolMin poolMin
*/
public void setPoolMin(String poolMin) {
this.poolMin = poolMin;
}
/**
* Gets the pool-max
* @return the pool-max
*/
public String getPoolMax() {
return poolMax;
}
/**
* Set the pool-max
* @param poolMax poolMax
*/
public void setPoolMax(String poolMax) {
this.poolMax = poolMax;
}
/**
* Gets the pool-max-age
* @return the pool-max-age
*/
public String getPoolMaxAge() {
return poolMaxAge;
}
/**
* Set the pool-max-age
* @param poolMaxAge poolMaxAge
*/
public void setPoolMaxAge(String poolMaxAge) {
this.poolMaxAge = poolMaxAge;
}
/**
* Gets the pstmt-max
* @return the pstmt-max
*/
public String getPstmtMax() {
return pstmtMax;
}
/**
* Set the pstmt-max
* @param pstmtMax pstmtMax
*/
public void setPstmtMax(String pstmtMax) {
this.pstmtMax = pstmtMax;
}
/**
* Gets the pool-max-age-minutes
* @return the pool-max-age-minutes
*/
public String getPoolMaxAgeMinutes() {
return poolMaxAgeMinutes;
}
/**
* Set the pool-max-age-minutes
* @param val pool-max-age-minutes
*/
public void setPoolMaxAgeMinutes(String val) {
poolMaxAgeMinutes = val;
}
/**
* Gets the pool-max-opentime
* @return the pool-max-opentime
*/
public String getPoolMaxOpentime() {
return poolMaxOpentime;
}
/**
* Set the pool-max-opentime
* @param val pool-max-opentime
*/
public void setPoolMaxOpentime(String val) {
poolMaxOpentime = val;
}
/**
* Gets the pool-max-waiters
* @return the pool-max-waiters
*/
public String getPoolMaxWaiters() {
return poolMaxWaiters;
}
/**
* Set the pool-max-waiters
* @param val pool-max-waiters
*/
public void setPoolMaxWaiters(String val) {
poolMaxWaiters = val;
}
/**
* Gets the pool-max-waittime
* @return pool-max-waittime
*/
public String getPoolMaxWaittime() {
return poolMaxWaittime;
}
/**
* Set the pool-max-waittime
* @param val pool-max-waittime
*/
public void setPoolMaxWaittime(String val) {
poolMaxWaittime = val;
}
/**
* Gets the pool-sampling-period
* @return pool-sampling-period
*/
public String getPoolSamplingPeriod() {
return poolSamplingPeriod;
}
/**
* Set the pool-sampling-period
* @param val pool-sampling-period
*/
public void setPoolSamplingPeriod(String val) {
poolSamplingPeriod = val;
}
/**
* Represents this element by it's XML description.
* @param indent use this indent for prefixing XML representation.
* @return the XML description of this object.
*/
public String toXML(int indent) {
StringBuffer sb = new StringBuffer();
sb.append(indent(indent));
sb.append("\n");
indent += 2;
// pool-init
sb.append(xmlElement(poolInit, "pool-init", indent));
// pool-min
sb.append(xmlElement(poolMin, "pool-min", indent));
// pool-max
sb.append(xmlElement(poolMax, "pool-max", indent));
if (poolMaxAge != null) {
// pool-max-age
sb.append(xmlElement(poolMaxAge, "pool-max-age", indent));
} else {
// pool-max-minutes
sb.append(xmlElement(poolMaxAgeMinutes, "pool-max-age-minutes", indent));
}
// pstmt-max
sb.append(xmlElement(pstmtMax, "pstmt-max", indent));
// pool-max-opentime
sb.append(xmlElement(poolMaxOpentime, "pool-max-opentime", indent));
// pool-max-waiters
sb.append(xmlElement(poolMaxWaiters, "pool-max-waiters", indent));
// pool-max-waittime
sb.append(xmlElement(poolMaxWaittime, "pool-max-waittime", indent));
// pool-sampling-period
sb.append(xmlElement(poolSamplingPeriod, "pool-sampling-period", indent));
indent -= 2;
sb.append(indent(indent));
sb.append(" \n");
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy