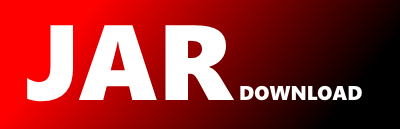
org.objectweb.jonas_rar.deployment.xml.Resourceadapter Maven / Gradle / Ivy
/**
* JOnAS: Java(TM) Open Application Server
* Copyright (C) 1999 Bull S.A.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
*
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or 1any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA
*
* Initial developer: Florent BENOIT
* --------------------------------------------------------------------------
* $Id: Resourceadapter.java 5699 2004-10-29 23:33:37Z ehardesty $
* --------------------------------------------------------------------------
*/
package org.objectweb.jonas_rar.deployment.xml;
import org.objectweb.jonas_lib.deployment.xml.AbsElement;
import org.objectweb.jonas_lib.deployment.xml.JLinkedList;
/**
* This class defines the implementation of the element resourceadapter
*
* @author Florent Benoit
*/
public class Resourceadapter extends AbsElement {
/**
* resourceadapter-class
*/
private String resourceadapterClass = null;
/**
* managedconnectionfactory-class
*/
private String managedconnectionfactoryClass = null;
/**
* connectionfactory-interface
*/
private String connectionfactoryInterface = null;
/**
* connectionfactory-impl-class
*/
private String connectionfactoryImplClass = null;
/**
* connection-interface
*/
private String connectionInterface = null;
/**
* connection-impl-class
*/
private String connectionImplClass = null;
/**
* transaction-support
*/
private String transactionSupport = null;
/**
* config-property
*/
private JLinkedList configPropertyList = null;
/**
* authentication-mechanism
*/
private JLinkedList authenticationMechanismList = null;
/**
* outbound-resourceadapter
*/
private OutboundResourceadapter outboundResourceadapter = null;
/**
* inbound-resourceadapter
*/
private InboundResourceadapter inboundResourceadapter = null;
/**
* adminobject
*/
private JLinkedList adminobjectList = null;
/**
* reauthentication-support
*/
private String reauthenticationSupport = null;
/**
* security-permission
*/
private JLinkedList securityPermissionList = null;
/**
* Constructor
*/
public Resourceadapter() {
super();
configPropertyList = new JLinkedList("config-property");
authenticationMechanismList = new JLinkedList("authentication-mechanism");
adminobjectList = new JLinkedList("adminobject");
securityPermissionList = new JLinkedList("security-permission");
}
/**
* Gets the resourceadapter-class
* @return the resourceadapter-class
*/
public String getResourceadapterClass() {
return resourceadapterClass;
}
/**
* Set the resourceadapter-class
* @param resourceadapterClass resourceadapterClass
*/
public void setResourceadapterClass(String resourceadapterClass) {
this.resourceadapterClass = resourceadapterClass;
}
/**
* Gets the managedconnectionfactory-class
* @return the managedconnectionfactory-class
*/
public String getManagedconnectionfactoryClass() {
return managedconnectionfactoryClass;
}
/**
* Set the managedconnectionfactory-class
* @param managedconnectionfactoryClass managedconnectionfactoryClass
*/
public void setManagedconnectionfactoryClass(String managedconnectionfactoryClass) {
this.managedconnectionfactoryClass = managedconnectionfactoryClass;
}
/**
* Gets the connectionfactory-interface
* @return the connectionfactory-interface
*/
public String getConnectionfactoryInterface() {
return connectionfactoryInterface;
}
/**
* Set the connectionfactory-interface
* @param connectionfactoryInterface connectionfactoryInterface
*/
public void setConnectionfactoryInterface(String connectionfactoryInterface) {
this.connectionfactoryInterface = connectionfactoryInterface;
}
/**
* Gets the connectionfactory-impl-class
* @return the connectionfactory-impl-class
*/
public String getConnectionfactoryImplClass() {
return connectionfactoryImplClass;
}
/**
* Set the connectionfactory-impl-class
* @param connectionfactoryImplClass connectionfactoryImplClass
*/
public void setConnectionfactoryImplClass(String connectionfactoryImplClass) {
this.connectionfactoryImplClass = connectionfactoryImplClass;
}
/**
* Gets the connection-interface
* @return the connection-interface
*/
public String getConnectionInterface() {
return connectionInterface;
}
/**
* Set the connection-interface
* @param connectionInterface connectionInterface
*/
public void setConnectionInterface(String connectionInterface) {
this.connectionInterface = connectionInterface;
}
/**
* Gets the connection-impl-class
* @return the connection-impl-class
*/
public String getConnectionImplClass() {
return connectionImplClass;
}
/**
* Set the connection-impl-class
* @param connectionImplClass connectionImplClass
*/
public void setConnectionImplClass(String connectionImplClass) {
this.connectionImplClass = connectionImplClass;
}
/**
* Gets the transaction-support
* @return the transaction-support
*/
public String getTransactionSupport() {
return transactionSupport;
}
/**
* Set the transaction-support
* @param transactionSupport transactionSupport
*/
public void setTransactionSupport(String transactionSupport) {
this.transactionSupport = transactionSupport;
}
/**
* Gets the config-property
* @return the config-property
*/
public JLinkedList getConfigPropertyList() {
return configPropertyList;
}
/**
* Set the config-property
* @param configPropertyList configProperty
*/
public void setConfigPropertyList(JLinkedList configPropertyList) {
this.configPropertyList = configPropertyList;
}
/**
* Add a new config-property element to this object
* @param configProperty the configPropertyobject
*/
public void addConfigProperty(ConfigProperty configProperty) {
configPropertyList.add(configProperty);
}
/**
* Gets the authentication-mechanism
* @return the authentication-mechanism
*/
public JLinkedList getAuthenticationMechanismList() {
return authenticationMechanismList;
}
/**
* Set the authentication-mechanism
* @param authenticationMechanismList authenticationMechanism
*/
public void setAuthenticationMechanismList(JLinkedList authenticationMechanismList) {
this.authenticationMechanismList = authenticationMechanismList;
}
/**
* Add a new authentication-mechanism element to this object
* @param authenticationMechanism the authenticationMechanismobject
*/
public void addAuthenticationMechanism(AuthenticationMechanism authenticationMechanism) {
authenticationMechanismList.add(authenticationMechanism);
}
/**
* Gets the outbound-resourceadapter
* @return the outbound-resourceadapter
*/
public OutboundResourceadapter getOutboundResourceadapter() {
return outboundResourceadapter;
}
/**
* Set the outbound-resourceadapter
* @param outboundResourceadapter outboundResourceadapter
*/
public void setOutboundResourceadapter(OutboundResourceadapter outboundResourceadapter) {
this.outboundResourceadapter = outboundResourceadapter;
}
/**
* Gets the inbound-resourceadapter
* @return the inbound-resourceadapter
*/
public InboundResourceadapter getInboundResourceadapter() {
return inboundResourceadapter;
}
/**
* Set the inbound-resourceadapter
* @param inboundResourceadapter inboundResourceadapter
*/
public void setInboundResourceadapter(InboundResourceadapter inboundResourceadapter) {
this.inboundResourceadapter = inboundResourceadapter;
}
/**
* Gets the adminobject
* @return the adminobject
*/
public JLinkedList getAdminobjectList() {
return adminobjectList;
}
/**
* Set the adminobject
* @param adminobjectList adminobject
*/
public void setAdminobjectList(JLinkedList adminobjectList) {
this.adminobjectList = adminobjectList;
}
/**
* Add a new adminobject element to this object
* @param adminobject the adminobjectobject
*/
public void addAdminobject(Adminobject adminobject) {
adminobjectList.add(adminobject);
}
/**
* Gets the reauthentication-support
* @return the reauthentication-support
*/
public String getReauthenticationSupport() {
return reauthenticationSupport;
}
/**
* Set the reauthentication-support
* @param reauthenticationSupport reauthenticationSupport
*/
public void setReauthenticationSupport(String reauthenticationSupport) {
this.reauthenticationSupport = reauthenticationSupport;
}
/**
* Gets the security-permission
* @return the security-permission
*/
public JLinkedList getSecurityPermissionList() {
return securityPermissionList;
}
/**
* Set the security-permission
* @param securityPermissionList securityPermission
*/
public void setSecurityPermissionList(JLinkedList securityPermissionList) {
this.securityPermissionList = securityPermissionList;
}
/**
* Add a new security-permission element to this object
* @param securityPermission the securityPermissionobject
*/
public void addSecurityPermission(SecurityPermission securityPermission) {
securityPermissionList.add(securityPermission);
}
/**
* Represents this element by it's XML description.
* @param indent use this indent for prefixing XML representation.
* @return the XML description of this object.
*/
public String toXML(int indent) {
StringBuffer sb = new StringBuffer();
sb.append(indent(indent));
sb.append("\n");
indent += 2;
// resourceadapter-class
sb.append(xmlElement(resourceadapterClass, "resourceadapter-class", indent));
// config-property
sb.append(configPropertyList.toXML(indent));
// outbound-resourceadapter
if (outboundResourceadapter != null) {
sb.append(outboundResourceadapter.toXML(indent));
}
// inbound-resourceadapter
if (inboundResourceadapter != null) {
sb.append(inboundResourceadapter.toXML(indent));
}
// adminobject
sb.append(adminobjectList.toXML(indent));
// security-permission
sb.append(securityPermissionList.toXML(indent));
indent -= 2;
sb.append(indent(indent));
sb.append(" \n");
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy