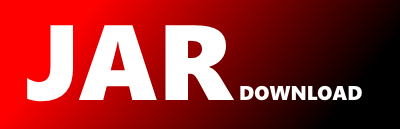
org.objectweb.jonas_ws.wsgen.WsGen Maven / Gradle / Ivy
The newest version!
/**
* JOnAS: Java(TM) Open Application Server
* Copyright (C) 1999-2005 Bull S.A.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA
*
* --------------------------------------------------------------------------
* $Id: WsGen.java 10556 2007-06-06 16:52:41Z sauthieg $
* --------------------------------------------------------------------------
*/
package org.objectweb.jonas_ws.wsgen;
import java.io.File;
import java.util.StringTokenizer;
import org.objectweb.jonas_lib.genbase.NoJ2EEWebservicesException;
import org.objectweb.jonas_lib.genbase.archive.Archive;
import org.objectweb.jonas_lib.genbase.generator.Config;
import org.objectweb.jonas_lib.genbase.generator.GeneratorFactories;
import org.objectweb.jonas_lib.genbase.modifier.ArchiveModifier;
import org.objectweb.jonas_lib.genbase.utils.TempRepository;
import org.objectweb.jonas_ws.wsgen.finder.GeneralWSFinder;
import org.objectweb.jonas_ws.wsgen.finder.J2EEWebServicesFinder;
import org.objectweb.jonas_ws.wsgen.generator.GeneratorFactory;
import org.objectweb.jonas_ws.wsgen.modifier.ModifierFactory;
import org.objectweb.jonas.common.Log;
import org.objectweb.util.monolog.api.BasicLevel;
import org.objectweb.util.monolog.api.Logger;
/**
* Main WsGen Class.
* @author Guillaume Sauthier
*/
public class WsGen {
/** logger */
private static Logger logger = Log.getLogger(Log.JONAS_WSGEN_PREFIX);
/**
* Set to false if input file cannot be processed by WsGen (DTDs).
*/
private boolean inputModified = true;
/**
* Private Constructor for Utility class WsGen.
*/
public WsGen() { }
/**
* Main method.
* @param args Commend line args
* @throws Exception When the execute method fails
*/
public static void main(String[] args) throws Exception {
WsGen wsgen = new WsGen();
wsgen.execute(args);
}
/**
* Method used by main method and by external class to retrieve the name of
* the modified or created file by WsGen
* @param args command line arguments
* @return the name of the modified or built
* @throws Exception When a failure occurs
*/
public String execute(String[] args) throws Exception {
GeneratorFactory gf = GeneratorFactory.getInstance();
// store configuration properties
Config config = parseInput(args);
if (config.isHelp() || config.isError() || config.getInputname() == null) {
// display usage message
usage();
return null;
}
// Make sure that DTD is turned to off
config.setDTDsAllowed(false);
// setup configuration
gf.setConfiguration(config);
GeneratorFactories.setCurrentFactory(gf);
Archive modifiedArchive = null;
ArchiveModifier am = null;
// get the ArchiveModifier
try {
am = ModifierFactory.getModifier(config.getInputname(), false);
J2EEWebServicesFinder finder = new GeneralWSFinder(am.getArchive());
// Do not touch the archive unless necessary !
if (finder.find()) {
logger.log(BasicLevel.INFO, "WebServices generation for '" + config.getInputname() + "'");
am.getArchive().initialize();
// modify the given archive
modifiedArchive = am.modify();
return modifiedArchive.getRootFile().getCanonicalPath();
}
} catch (NoJ2EEWebservicesException e) {
logger.log(BasicLevel.WARN, config.getInputname() + " is using DTDs, WsGen needs Schema only : "
+ e.getMessage());
inputModified = false;
return config.getInputname();
} finally {
// close archive
if (modifiedArchive != null) {
modifiedArchive.close();
}
modifiedArchive = null;
am = null;
// delete all the temporary files created
TempRepository.getInstance().deleteAll();
}
// no changes needed ...
inputModified = false;
return config.getInputname();
}
/**
* Parse Command Line input and returns a Config object
* @param args Command Lines params
* @return a Config object
*/
private static Config parseInput(String[] args) {
Config config = new Config();
// Get args
for (int argn = 0; argn < args.length; argn++) {
String arg = args[argn];
if (arg.equals("-help") || arg.equals("-?")) {
config.setHelp(true);
continue;
}
if (arg.equals("-verbose")) {
config.setVerbose(true);
continue;
}
if (arg.equals("-debug")) {
config.setDebug(true);
config.setVerbose(true);
continue;
}
if (arg.equals("-keepgenerated")) {
config.setKeepGenerated(true);
continue;
}
if (arg.equals("-noconfig")) {
config.setNoConfig(true);
continue;
}
if (arg.equals("-novalidation")) {
config.setParseWithValidation(false);
continue;
}
if (arg.equals("-javac")) {
config.setNameJavac(args[++argn]);
continue;
}
if (arg.equals("-javacopts")) {
argn++;
if (argn < args.length) {
StringTokenizer st = new StringTokenizer(args[argn]);
while (st.hasMoreTokens()) {
config.getJavacOpts().add(st.nextToken());
}
} else {
config.setError(true);
}
continue;
}
if (arg.equals("-d")) {
argn++;
if (argn < args.length) {
config.setOut(new File(args[argn]));
} else {
config.setError(true);
}
continue;
}
if (arg.equals("-unpacked")) {
config.setSaveUnpacked();
continue;
}
if (args[argn] != null) {
config.setInputname(args[argn]);
} else {
config.setError(true);
}
}
return config;
}
/**
* Display the usage
*/
public static void usage() {
StringBuffer msg = new StringBuffer();
msg.append("Usage: java org.objectweb.jonas_ws.wsgen.WsGen -help \n");
msg.append(" to print this help message \n");
msg.append(" or java org.objectweb.jonas_ws.wsgen.WsGen \n");
msg.append("Options include: \n");
msg.append(" -d specify where to place the generated files \n");
msg.append(" -novalidation parse the XML deployment descriptors without \n");
msg.append(" validation \n");
msg.append(" -javac specify the java compiler to use \n");
msg.append(" -javacopts specify the options to pass to the java compiler \n");
msg.append(" -keepgenerated do not delete intermediate generated files \n");
msg.append(" -noconfig do not generate configuration files (require \n");
msg.append(" user provided files) \n");
msg.append(" -verbose \n");
msg.append(" -debug \n");
msg.append(" \n");
msg.append(" Input_File the ejb-jar, war or ear filename\n");
System.out.println(msg.toString());
}
/**
* @return Returns the inputModified.
*/
public boolean isInputModified() {
return inputModified;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy