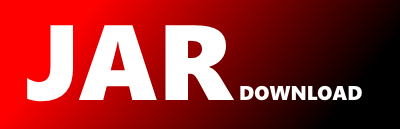
org.objectweb.jonas.web.War Maven / Gradle / Ivy
/**
* JOnAS: Java(TM) Open Application Server
* Copyright (C) 1999-2005 Bull S.A.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA
*
* --------------------------------------------------------------------------
* $Id: War.java 10539 2007-06-05 17:33:33Z benoitf $
* --------------------------------------------------------------------------
*/
package org.objectweb.jonas.web;
import java.net.URL;
import org.objectweb.jonas.web.lib.PermissionManager;
/**
* This class is representing a War (a web application) structure which is
* composed of :
* - the URL of the war file.
* - the URL of the ear containing this war.
* - the name of the host on which this war is deployed.
* - the context root of this war.
* - compliance to the java 2 delegation model.
* - Content of the web.xml file
* - Content of the jonas-web.xml file
* - List of the servlets name
* - A permission manager
* @author Florent Benoit
*/
public class War implements WarMBean {
/**
* The URL of the war file.
*/
private URL warURL = null;
/**
* The URL of the ear containing this war.
*/
private URL earURL = null;
/**
* The name of the host on which this war is deployed.
*/
private String hostName = null;
/**
* The context root where this war is deployed.
*/
private String contextRoot = null;
/**
* Compliance to the java 2 delegation model.
*/
private boolean java2DelegationModel = true;
/**
* Content of the web.xml file
*/
private String xmlContent = null;
/**
* Content of the jonas-web.xml file
*/
private String jonasXmlContent = null;
/**
* Names of the servlet
*/
private String[] servletsName = null;
/**
* Permission Manager used
*/
private PermissionManager permissionManager = null;
/**
* Construct a War with the specified name, of the specified
* ear. (Used in the case of an ear application).
* @param warURL the URL of the war.
* @param earURL the URL of the ear containing this war.
* @param hostName the name of the host on which this war is deployed.
* @param contextRoot the context root of this war.
* @param java2DelegationModel the java2 delegation model compliance
* @param xmlContent content of the web.xml file
* @param jonasXmlContent content of the jonas-web.xml file
* @param servletsName name of the servlets
*/
public War(final URL warURL, final URL earURL,
final String hostName, final String contextRoot,
final boolean java2DelegationModel,
final String xmlContent,
final String jonasXmlContent,
final String[] servletsName) {
this.warURL = warURL;
this.earURL = earURL;
this.hostName = hostName;
this.contextRoot = contextRoot;
this.java2DelegationModel = java2DelegationModel;
this.xmlContent = xmlContent;
this.jonasXmlContent = jonasXmlContent;
this.servletsName = servletsName;
}
/**
* Return true if only if this war is in an ear file.
* @return true if only if this war is in an ear file.
*/
public boolean isInEarCase() {
return earURL != null;
}
/**
* Get the URL of the war file.
* @return the URL of the war file.
*/
public URL getWarURL() {
return warURL;
}
/**
* Get the URL of the ear containing this war.
* @return the URL of the war file.
*/
public URL getEarURL() {
return earURL;
}
/**
* Get the name of the host on which this war is deployed.
* @return the name of the host on which this war is deployed.
*/
public String getHostName() {
return hostName;
}
/**
* Get the context root of this war.
* @return the context root of this war.
*/
public String getContextRoot() {
return contextRoot;
}
/**
* Context classloader must follow the java2 delegation model ?
* @return true if the context's classloader must follow the java 2 delegation model.
*/
public boolean getJava2DelegationModel() {
return java2DelegationModel;
}
/**
* Return the standard xml file
* @return the standard xml file
*/
public String getXmlContent() {
return xmlContent;
}
/**
* Return the jonas-specific xml file
* @return the jonas-specific xml file
*/
public String getJOnASXmlContent() {
return jonasXmlContent;
}
/**
* Return a list of all servlets available
* @return a list of all servlets available
*/
public String[] getServletsName() {
return servletsName;
}
/**
* Set the permission manager
* @param permissionManager permission manager to set
*/
public void setPermissionManager(PermissionManager permissionManager) {
this.permissionManager = permissionManager;
}
/**
* Gets the permission manager
* @return permission manager
*/
public PermissionManager getPermissionManager() {
return permissionManager;
}
/**
* Gets a contextId for this module
* @return contextId
*/
public String getContextId() {
return getWarURL().getFile() + contextRoot;
}
/**
* Return true if only if the specified war is equal to this war.
* @param war the war to compare for the equality.
* @return true if only if the specified war is equal to this war.
*/
public boolean equals(War war) {
return war.getWarURL().equals(warURL)
&& war.getEarURL().equals(earURL)
&& war.getHostName().equals(hostName)
&& war.getContextRoot().equals(contextRoot)
&& (war.getJava2DelegationModel() == java2DelegationModel);
}
/**
* @return an hashcode for this object
* @see java.lang.Object#hashCode()
*/
public int hashCode() {
return warURL.hashCode();
}
/**
* Return a string representation of the war. Used for debug.
* @return a string representation of the war.
*/
public String toString() {
StringBuffer ret = new StringBuffer();
ret.append("WAR=\n");
ret.append("\twarURL=" + getWarURL() + "\n");
ret.append("\tearURL=" + getEarURL() + "\n");
ret.append("\thostName=" + getHostName() + "\n");
ret.append("\tcontextRoot=" + getContextRoot());
ret.append("\tjava2DelegationModel=" + getJava2DelegationModel());
return ret.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy