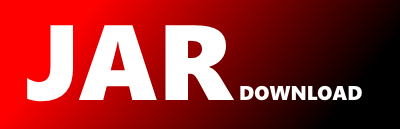
org.objectweb.petals.plugin.jbiplugin.JBIAbstractJMXMojo Maven / Gradle / Ivy
/**
* PETALS - PETALS Services Platform.
* Copyright (c) 2005 EBM Websourcing, http://www.ebmwebsourcing.com/
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* -------------------------------------------------------------------------
* $Id: AntTaskJBI.java 09:01:14 ddesjardins $
* -------------------------------------------------------------------------
*/
package org.objectweb.petals.plugin.jbiplugin;
import java.util.ArrayList;
import java.util.List;
import java.util.logging.Logger;
import org.apache.maven.plugin.MojoExecutionException;
import org.objectweb.petals.jbi.descriptor.ComponentDescription;
import org.objectweb.petals.jbi.descriptor.Identification;
import org.objectweb.petals.jbi.descriptor.JBIDescriptor;
import org.objectweb.petals.jbi.descriptor.ServiceAssembly;
import org.objectweb.petals.jbi.descriptor.ServiceUnit;
import org.objectweb.petals.jbi.descriptor.SharedLibrary;
import org.objectweb.petals.jbi.descriptor.SharedLibraryList;
/**
* This class is used to give the main configuration parameters of all JBI goals
*
* @author cdeneux - Capgemini Sud
*/
public abstract class JBIAbstractJMXMojo extends JBIAbstractMojo {
/**
* Hostname of the Petals server
*
* @parameter expression="localhost"
* @editable true
* @required
* @description Hostname of the Petals server
*/
protected String host;
/**
* JMX Port of the Petals server
*
* @parameter expression="7700"
* @editable true
* @required
* @description Hostname JMX Port of the Petals server
*/
protected Integer port;
/**
* JMX username of the Petals server
*
* @parameter expression=""
* @editable true
* @description JMX username of the Petals server
*/
protected String username;
/**
* Password of the Petals JMX server username
*
* @parameter expression=""
* @editable true
* @description Password of the Petals JMX server username
*/
protected String password;
/**
* Flag to force the goal processing, if needed.
*
* @parameter expression="false"
* @editable true
* @description Flag to force the goal processing, if needed.
*/
protected Boolean force;
protected Logger log = Logger.getLogger(JBIAbstractJMXMojo.class.getName());
/**
* Read component information from the parsed JBI descriptor
*
* @throws MojoExecutionException
*/
protected String getComponentNameFromJBIDescriptor(
final JBIDescriptor jbiDescriptor) throws MojoExecutionException {
ComponentDescription componentDescription = jbiDescriptor
.getComponent();
if (componentDescription == null) {
throw new MojoExecutionException("JBI Component descriptor is null");
}
Identification identification = componentDescription
.getIdentification();
if (identification == null) {
throw new MojoExecutionException(
"JBI Component descriptor: is null");
}
return identification.getName();
}
/**
* Read service assembly information from the parsed JBI descriptor.
*
* @throws MojoExecutionException
*/
protected String getServiceAssemblyNameFromJBIDescriptor(
final JBIDescriptor jbiDescriptor) throws MojoExecutionException {
ServiceAssembly serviceAssemblyDescription = jbiDescriptor
.getServiceAssembly();
if (serviceAssemblyDescription == null) {
throw new MojoExecutionException(
"JBI Service assembly descriptor is null");
}
Identification identification = serviceAssemblyDescription
.getIdentification();
if (identification == null) {
throw new MojoExecutionException(
"JBI Service assembly descriptor: is null");
}
return identification.getName();
}
/**
* Read shared-library information from the parsed JBI descriptor.
*
* @throws MojoExecutionException
*/
protected String getSharedLibraryNameFromJBIDescriptor(
final JBIDescriptor jbiDescriptor) throws MojoExecutionException {
SharedLibrary sharedLibraryDescription = jbiDescriptor
.getSharedLibrary();
if (sharedLibraryDescription == null) {
throw new MojoExecutionException(
"JBI Service assembly descriptor is null");
}
Identification identification = sharedLibraryDescription
.getIdentification();
if (identification == null) {
throw new MojoExecutionException(
"JBI Shared-library descriptor: is null");
}
return identification.getName();
}
/**
* Retreive all components declared in the service assembly JBI Descriptor
*
* @throws MojoExecutionException
*/
protected List getComponentsFromServiceAssemblyJBIDescriptor(
final JBIDescriptor jbiDescriptor) throws MojoExecutionException {
ServiceAssembly serviceAssemblyDescription = jbiDescriptor
.getServiceAssembly();
if (serviceAssemblyDescription == null) {
throw new MojoExecutionException(
"JBI Service assembly descriptor is null");
}
List usedComponents = new ArrayList();
List serviceUnits = serviceAssemblyDescription
.getServiceUnits();
for (ServiceUnit serviceUnit : serviceUnits) {
String componentName = serviceUnit.getTargetComponentName();
if (!usedComponents.contains(componentName)) {
usedComponents.add(componentName);
}
}
return usedComponents;
}
/**
* Retreive all shared-libraries declared in a component JBI Descriptor
*
* @throws MojoExecutionException
*/
protected List getSharedLibrariesFromComponentJBIDescriptor(
final JBIDescriptor jbiDEscriptor) throws MojoExecutionException {
ComponentDescription componentDescription = jbiDEscriptor
.getComponent();
if (componentDescription == null) {
throw new MojoExecutionException(
"JBI component descriptor is null");
}
List usedSharedLibraies = new ArrayList();
List sharedLibraries = componentDescription
.getSharedLibraryList();
for (SharedLibraryList sharedLibrary : sharedLibraries) {
String sharedLibraryName = sharedLibrary.getName();
if (!usedSharedLibraies.contains(sharedLibraryName)) {
usedSharedLibraies.add(sharedLibraryName);
}
}
return usedSharedLibraies;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy