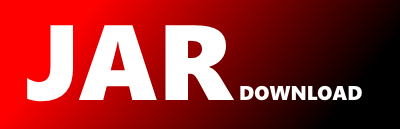
org.objectweb.petals.plugin.jbiplugin.JBIAbstractMojo Maven / Gradle / Ivy
/**
* PETALS - PETALS Services Platform.
* Copyright (c) 2005 EBM Websourcing, http://www.ebmwebsourcing.com/
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* -------------------------------------------------------------------------
* $Id: AntTaskJBI.java 09:01:14 ddesjardins $
* -------------------------------------------------------------------------
*/
package org.objectweb.petals.plugin.jbiplugin;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.util.zip.ZipEntry;
import java.util.zip.ZipFile;
import org.apache.maven.artifact.factory.ArtifactFactory;
import org.apache.maven.artifact.repository.ArtifactRepository;
import org.apache.maven.artifact.resolver.ArtifactResolver;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.project.MavenProject;
import org.apache.maven.project.MavenProjectBuilder;
import org.objectweb.petals.jbi.descriptor.JBIDescriptor;
import org.objectweb.petals.jbi.descriptor.JBIDescriptorBuilder;
import org.objectweb.petals.jbi.descriptor.JBIDescriptorException;
/**
* This class is used to give the main configuration parameters of
* all JBI goals
*
* @author cdeneux - Capgemini Sud
*/
public abstract class JBIAbstractMojo extends AbstractMojo {
/*
* Definition of all possible values of :
* - jbi-component <-> binding-component or service-engine
* - jbi-service-unit <-> service-unit
* - jbi-service-assembly <-> service-assembly
* - jbi-shared-library <-> shared-library
*/
protected static final String PACKAGING_COMPONENT = "jbi-component";
protected static final String PACKAGING_SU = "jbi-service-unit";
protected static final String PACKAGING_SA = "jbi-service-assembly";
protected static final String PACKAGING_SL = "jbi-shared-library";
/**
* @component
*/
protected ArtifactResolver artifactResolver;
/**
* JBI archive name (will be suffixed by .zip).
*
* @parameter expression="${project.artifactId}-${project.version}"
* @editable true
* @required
* @description Name of the generated jbi archive (will be suffixed by .zip).
*/
protected String jbiName;
/**
* Verbose
*
* @parameter expression="false"
* @editable true
* @required
* @description Boolean to switch to verbose mode
*/
protected boolean verbose;
/**
* Local maven repository.
*
* @parameter expression="${localRepository}"
* @required
* @readonly
*/
protected ArtifactRepository localRepository;
/**
* Output directory
*
* @parameter expression="${project.build.directory}"
* @required
*/
protected File outputDirectory;
/**
* The Maven Project.
*
* @parameter expression="${project}"
* @required
* @readonly
*/
protected MavenProject project;
/**
* @parameter expression="${project.packaging}"
* @readonly
* @required
*/
protected String packaging;
/**
* JBI conf directory
*
* @parameter expression="${basedir}/src/main/jbi"
* @editable true
* @required
* @description Path of the JBI source directory. This directory will be
* zipped as it is in the directory META-INF. It should contain
* only the file jbi.xml. Other files should be read through
* the classpath.
*/
protected File jbiDirectory;
/**
* @component
* Needed to retrieve needed JBI archive dependencies.
*/
protected ArtifactFactory artifactFactory;
/**
* @component
* Needed to retrieve needed JBI archive dependencies.
*/
protected MavenProjectBuilder mavenProjectBuilder;
/**
* Read and parse the JBI descriptor of an artifact
*
* @throws MojoExecutionException
*/
final protected JBIDescriptor readJbiDescriptor(final File file)
throws MojoExecutionException {
try {
final ZipFile zipFile = new ZipFile(file);
final ZipEntry jbiDescriptorZipEntry = zipFile
.getEntry("META-INF/jbi.xml");
final InputStream jbiDescriptorInputStream = zipFile
.getInputStream(jbiDescriptorZipEntry);
// Load the JBI descriptor
final JBIDescriptor jbiDescriptor = JBIDescriptorBuilder.getInstance()
.buildDescriptor(jbiDescriptorInputStream);
if (jbiDescriptor == null) {
throw new MojoExecutionException("JBI Descriptor is null");
}
return jbiDescriptor;
} catch (JBIDescriptorException e) {
throw new MojoExecutionException("Error loading JBI descriptor", e);
} catch (IOException e) {
throw new MojoExecutionException(
"Error retrieving the JBI descriptor in the JBI archive or loading the jbi xsd schema", e);
}
}
protected void debug(String msg) {
this.getLog().debug(msg);
}
protected void info(String msg) {
if (verbose) {
this.getLog().info(msg);
}
}
protected void warn(String msg) {
this.getLog().warn(msg);
}
protected void error(String msg) {
this.getLog().error(msg);
}
protected void error(String msg, Throwable exception) {
this.getLog().error(msg, exception);
}
/**
* Execute the plugin
*/
final public void execute() throws MojoExecutionException, MojoFailureException {
if (PACKAGING_COMPONENT.equals(this.project.getPackaging())
|| PACKAGING_SA.equals(this.project.getPackaging())
|| PACKAGING_SL.equals(this.project.getPackaging())
|| PACKAGING_SU.equals(this.project.getPackaging())) {
this.executeMojo();
}
else {
this.debug("The project is not a JBI artifact so the plugin skips it.");
}
}
abstract protected void executeMojo() throws MojoExecutionException, MojoFailureException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy