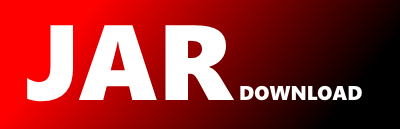
org.objectweb.petals.plugin.jbiplugin.JBIInstallMojo Maven / Gradle / Ivy
/**
* PETALS - PETALS Services Platform.
* Copyright (c) 2005 EBM Websourcing, http://www.ebmwebsourcing.com/
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* -------------------------------------------------------------------------
* $Id: PackageAssemblyMojo.java 16:56:43 ddesjardins $
* -------------------------------------------------------------------------
*/
package org.objectweb.petals.plugin.jbiplugin;
import java.io.File;
import java.net.InetAddress;
import java.net.MalformedURLException;
import java.net.URISyntaxException;
import java.net.URL;
import java.net.UnknownHostException;
import java.util.ArrayList;
import java.util.List;
import org.apache.maven.artifact.Artifact;
import org.apache.maven.artifact.manager.WagonConfigurationException;
import org.apache.maven.artifact.manager.WagonManager;
import org.apache.maven.artifact.resolver.ArtifactNotFoundException;
import org.apache.maven.artifact.resolver.ArtifactResolutionException;
import org.apache.maven.artifact.versioning.InvalidVersionSpecificationException;
import org.apache.maven.artifact.versioning.VersionRange;
import org.apache.maven.model.Dependency;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.project.MavenProject;
import org.apache.maven.project.ProjectBuildingException;
import org.apache.maven.wagon.ConnectionException;
import org.apache.maven.wagon.ResourceDoesNotExistException;
import org.apache.maven.wagon.TransferFailedException;
import org.apache.maven.wagon.UnsupportedProtocolException;
import org.apache.maven.wagon.Wagon;
import org.apache.maven.wagon.authentication.AuthenticationException;
import org.apache.maven.wagon.authorization.AuthorizationException;
import org.apache.maven.wagon.observers.Debug;
import org.apache.maven.wagon.repository.Repository;
import org.objectweb.petals.jbi.descriptor.ComponentDescription;
import org.objectweb.petals.jbi.descriptor.JBIDescriptor;
import org.objectweb.petals.jbi.descriptor.SharedLibrary;
import org.objectweb.petals.tools.jmx.api.PetalsComponentComponentClient;
import org.objectweb.petals.tools.jmx.api.PetalsDeploymentServiceClient;
import org.objectweb.petals.tools.jmx.api.PetalsInstallationServiceClient;
import org.objectweb.petals.tools.jmx.api.PetalsInstallerComponentClient;
import org.objectweb.petals.tools.jmx.api.PetalsJMXClient;
import org.objectweb.petals.tools.jmx.api.exception.AttributeErrorException;
import org.objectweb.petals.tools.jmx.api.exception.ComponentComponentDoesNotExistException;
import org.objectweb.petals.tools.jmx.api.exception.ComponentErrorException;
import org.objectweb.petals.tools.jmx.api.exception.ConnectionErrorException;
import org.objectweb.petals.tools.jmx.api.exception.DeploymentServiceDoesNotExistException;
import org.objectweb.petals.tools.jmx.api.exception.DeploymentServiceErrorException;
import org.objectweb.petals.tools.jmx.api.exception.InstallationServiceDoesNotExistException;
import org.objectweb.petals.tools.jmx.api.exception.InstallationServiceErrorException;
import org.objectweb.petals.tools.jmx.api.exception.InstallerComponentDoesNotExistException;
import org.objectweb.petals.tools.jmx.api.exception.PerformActionErrorException;
/**
* Install a JBI Archive
*
* @goal jbi-install
* @description Install a JBI Archive
*
* @author cdeneux - Capgemini Sud
*/
public class JBIInstallMojo extends JBIAbstractJMXMojo {
/**
* When installing a service assembly, if some component are not started,
* they can be installed and started from Maven repositories if this flag
* value is true
. If a such component should be installed
* and needs a shared-library, the shared-library processing is drived
* according to the parameter installMissingSharedLibraries
.
* This parameter is mainly used to install the JBI archives that are not in
* the Maven reactor. A component inside the Maven reactor will be installed
* before its service assemblies by the ordering of Maven dependency
* mechanism, or a shared-library for a component.
*
* @parameter expression="true"
* @editable true
* @description When installing a service assembly, if some component are
* not started, they can be installed and started from Maven
* repositories if this flag value is true
. If
* a such component should be installed and needs a
* shared-library, the shared-library processing is drived
* according to the parameter
* installMissingSharedLibraries
. This
* parameter is mainly used to install the JBI archives that
* are not in the Maven reactor. A component inside the Maven
* reactor will be installed before its service assemblies by
* the ordering of Maven dependency mechanism, or a
* shared-library for a component.
*/
private Boolean startMissingComponents;
/**
* When installing a component, if some shared-libaries are not installed,
* they can be installed from Maven repositories if this flag value is
* true
. This parameter is mainly used to install the
* shared-libraries that are not in the Maven reactor. A shared-library
* inside the Maven reactor will be installed before its components by the
* ordering of Maven dependency mechanism.
*
* @parameter expression="true"
* @editable true
* @description When installing a component, if some shared-libaries are not
* installed, they can be installed from Maven repositories if
* this flag value is true
. This parameter is
* mainly used to install the shared-libraries that are not in
* the Maven reactor. A shared-library inside the Maven reactor
* will be installed before its components by the ordering of
* Maven dependency mechanism.
*/
private Boolean installMissingSharedLibraries;
/**
* Specifies the server id (in settings.xml) to retrieve the credentials
* needed to upload the JBI archive on the Petals server if it is different
* from the local host.
*
* @parameter expression=""
* @editable true
* @description Specifies the server id (in settings.xml) to retrieve the
* credentials needed to upload the JBI archive on the Petals
* server if it is different from the local host.
*/
private String serverId;
/**
* The protocol to use to upload the JBI archive on a remote Petals server.
* Should be a protocol supported by Wagon.
*
* @parameter expression="scp"
* @editable true
* @description The protocol to use to upload the JBI archive on a remote
* Petals server. Should be a protocol supported by Wagon.
*/
private String protocol;
/**
* The directory of the Petals server on which the JBI archive will be
* uploaded if needed.
*
* @parameter expression="/tmp"
* @editable true
* @description The directory of the Petals server on which the JBI archive
* will be uploaded if needed.
*/
private String remoteDirectory;
/**
* @component
*/
private WagonManager wagonManager;
/**
* Execute the plugin
*/
@Override
public void executeMojo() throws MojoExecutionException, MojoFailureException {
if (project.getPackaging().equals(PACKAGING_COMPONENT)
|| project.getPackaging().equals(PACKAGING_SU)
|| project.getPackaging().equals(PACKAGING_SA)
|| project.getPackaging().equals(PACKAGING_SL)) {
try {
JBIDescriptor jbiDescriptor = this.readJbiDescriptor(project.getArtifact().getFile());
this.info("Using JMX Petals server located at: " + this.host
+ ":" + this.port);
PetalsJMXClient petalsJMXClient = new PetalsJMXClient(
this.host, this.port, this.username, this.password);
if (PACKAGING_COMPONENT.equals(project.getPackaging())) {
this.installComponent(petalsJMXClient, jbiDescriptor);
} else if (PACKAGING_SU.equals(project.getPackaging())) {
/*
* NOP because an SU is not installable, see its service
* assembly.
*
* No error is returned to be able to use the same plugin
* configuration for all JBI artefacts.
*/
this
.info("Install a service-unit has no sens. This goal is skipped.");
} else if (PACKAGING_SA.equals(project.getPackaging())) {
this.installServiceAssembly(petalsJMXClient, jbiDescriptor);
} else if (PACKAGING_SL.equals(project.getPackaging())) {
this.installSharedLibrary(petalsJMXClient, jbiDescriptor);
}
} catch (ConnectionErrorException e) {
throw new MojoExecutionException("Error", e);
}
}
else {
this.info("This artefact is not a JBI artefact. Skiped.");
}
}
private void installComponent(PetalsJMXClient petalsJMXClient, final JBIDescriptor jbiDescriptor)
throws MojoExecutionException {
try {
URL jbiPackageURL = new File(outputDirectory + File.separator + jbiName
+ ".zip").toURI().toURL();
installComponentCore(petalsJMXClient, jbiDescriptor,
jbiPackageURL, "");
} catch (MalformedURLException e) {
throw new MojoExecutionException(
"Invalid URL for JBI archive location", e);
}
}
private void installComponentCore(PetalsJMXClient petalsJMXClient,
final JBIDescriptor jbiDescriptor,
URL jbiPackageURL, final String prefixLog)
throws MojoExecutionException {
try {
boolean componentInstalled = true;
boolean componentInstallerLoaded = true;
String componentName = this.getComponentNameFromJBIDescriptor(jbiDescriptor);
PetalsComponentComponentClient pccc = null;
PetalsInstallerComponentClient picc = null;
PetalsInstallationServiceClient pisc = petalsJMXClient
.getInstallationServiceClient();
// We check if the component and its installer exist
try {
pccc = petalsJMXClient
.getComponentComponentClient(componentName);
} catch (ComponentComponentDoesNotExistException e1) {
// The component is not installed
this.info(prefixLog + "The component is not installed.");
componentInstalled = false;
}
try {
picc = petalsJMXClient
.getInstallerComponentClient(componentName);
} catch (InstallerComponentDoesNotExistException e1) {
// The component installer is not loaded
this.info(prefixLog+ "The component installer is not loaded.");
componentInstallerLoaded = false;
}
if (!this.force && componentInstalled) {
throw new MojoExecutionException(
"The component is already installed");
} else if (!this.force && componentInstallerLoaded) {
throw new MojoExecutionException(
"The component installer is already loaded");
} else if (this.force) {
// We force the installation
this.info(prefixLog + "The force mode is asked.");
if (componentInstalled) {
// We force the shutdown of the component:
if (PetalsComponentComponentClient.State.STARTED
.equals(pccc.getState())) {
// We stop the component
this.info(prefixLog + "Stoping the component.");
pccc.stop();
}
if (PetalsComponentComponentClient.State.STOPPED
.equals(pccc.getState())) {
// Check and shutdown SA if needed
this.checkAndUndeployServiceAssemblies(petalsJMXClient, componentName, "\t");
// We shutdown the component
this.info(prefixLog + "Shutdowning the component.");
pccc.shutdown();
}
if (!PetalsComponentComponentClient.State.SHUTDOWN
.equals(pccc.getState())) {
// The component state is not "SHUTDOWN", so a problem
// occured during the component shutdowning.
throw new MojoExecutionException(
"The component is not in the expected state (SHUTDOWN)");
}
this.info(prefixLog + "The component is shutdowned.");
if (picc.isInstalled()) {
this.info(prefixLog + "Uninstalling the component.");
picc.uninstall();
}
this.info(prefixLog + "The component is not installed.");
}
// At this point the component is not installed
if (componentInstallerLoaded) {
// We unload the installer
this.info(prefixLog + "Unloading the component installer.");
pisc.unloadInstaller(componentName);
this.info(prefixLog + "The component installer is not loaded.");
}
}
// At this point, we have a clean environement.
// We check that all needed shared-libraries are installed
if (this.force) {
this.info(prefixLog + "Checking needed shared-libraries ...");
checkAndInstallSharedLibraries(petalsJMXClient, jbiDescriptor, prefixLog + "\t");
this.info(prefixLog + "Needed shared-libraries installed.");
}
// We can install the JBI component
jbiPackageURL = uploadJBIArchiveIfNeeded(jbiPackageURL, prefixLog + "\t");
this.info(prefixLog + "Loading the component installer ("
+ jbiPackageURL.toExternalForm() + ").");
picc = pisc.loadNewInstaller(jbiPackageURL);
this.info(prefixLog + "The component installer is loaded.");
this.info(prefixLog + "Installing the component.");
picc.install();
this.info(prefixLog + "The component is installed.");
} catch (ComponentErrorException e) {
throw new MojoExecutionException("Error", e);
} catch (ConnectionErrorException e) {
throw new MojoExecutionException("Error", e);
} catch (AttributeErrorException e) {
throw new MojoExecutionException("Error", e);
} catch (PerformActionErrorException e) {
throw new MojoExecutionException("Error", e);
} catch (InstallationServiceErrorException e) {
throw new MojoExecutionException("Error", e);
} catch (InstallationServiceDoesNotExistException e) {
throw new MojoExecutionException(
"Unable to find the installation service ", e);
}
}
private void installServiceAssembly(PetalsJMXClient petalsJMXClient,
final JBIDescriptor jbiDescriptor) throws MojoExecutionException {
try {
String serviceAssemblyName = this
.getServiceAssemblyNameFromJBIDescriptor(jbiDescriptor);
URL jbiPackageURL = new File(outputDirectory + File.separator
+ jbiName + ".zip").toURI().toURL();
installServiceAssemblyCore(petalsJMXClient, jbiDescriptor,
serviceAssemblyName, jbiPackageURL, force.booleanValue(),
"");
} catch (MalformedURLException e) {
throw new MojoExecutionException(
"Invalid URL for JBI archive location", e);
}
}
private void installServiceAssemblyCore(PetalsJMXClient petalsJMXClient,
final JBIDescriptor jbiDescriptor, String serviceAssemblyName,
URL jbiPackageURL, boolean forceInstallation, final String prefixLog)
throws MojoExecutionException {
try {
PetalsDeploymentServiceClient pdsc = petalsJMXClient
.getDeploymentServiceClient();
boolean serviceAssemblyFound = pdsc
.isServiceAssemblyDeployed(serviceAssemblyName);
if (!forceInstallation && serviceAssemblyFound) {
throw new MojoExecutionException(
"The service-assembly is already deployed");
} else if (!serviceAssemblyFound) {
this.info(prefixLog + "Service-assembly is not deployed.");
}
// We undeploy the service-assembly if it was previously deployed
if (forceInstallation && serviceAssemblyFound) {
this.info(prefixLog + "The force mode is asked.");
this.info(prefixLog + "The service-assembly will be undeployed.");
// We check its state to known if we can undeploy it
if (PetalsDeploymentServiceClient.State.STARTED.equals(pdsc
.getState(serviceAssemblyName))) {
// We stop it
this.info("The service assembly is started, we stop it.");
String result = pdsc.stop(serviceAssemblyName);
this.info("service-assembly successfully stopped: " + result);
}
if (PetalsDeploymentServiceClient.State.STOPPED.equals(pdsc
.getState(serviceAssemblyName))) {
// We shutdown it
this.info("The service assembly is stopped, we shutdown it.");
String result = pdsc.shutdown(serviceAssemblyName);
this.info("service-assembly successfully shutdowned: " + result);
}
if (PetalsDeploymentServiceClient.State.SHUTDOWN.equals(pdsc
.getState(serviceAssemblyName))) {
// We undeploy it
this.info("The service assembly is shutdowned, we undeploy it.");
String result = pdsc.undeploy(serviceAssemblyName);
this.info(prefixLog + "service-assembly successfully undeployed: " + result);
}
else {
throw new MojoExecutionException(
"Error, the service assembly "
+ serviceAssemblyName
+ " is in the following state: "
+ pdsc.getState(serviceAssemblyName));
}
}
// At this point, we have a clean environnment
// We check that all needed components are installed
this.info(prefixLog + "Checking needed components ...");
checkAndStartComponents(petalsJMXClient, jbiDescriptor, prefixLog + "\t");
this.info(prefixLog + "Needed components installed.");
// We can deploy the service assembly
jbiPackageURL = uploadJBIArchiveIfNeeded(jbiPackageURL, prefixLog
+ "\t");
this.info(prefixLog + "Deploying the service-assembly ("
+ jbiPackageURL.toExternalForm() + ").");
String result = pdsc.deploy(jbiPackageURL);
this.info(prefixLog + "The service-assembly ("
+ serviceAssemblyName + ") is deployed: " + result);
} catch (ConnectionErrorException e) {
throw new MojoExecutionException("Error", e);
} catch (PerformActionErrorException e) {
throw new MojoExecutionException("Error", e);
} catch (DeploymentServiceErrorException e) {
throw new MojoExecutionException("Error", e);
} catch (DeploymentServiceDoesNotExistException e) {
throw new MojoExecutionException(
"Unable to find the deployment service ", e);
}
}
private void installSharedLibrary(PetalsJMXClient petalsJMXClient,
final JBIDescriptor jbiDescriptor) throws MojoExecutionException {
try {
String sharedLibraryName = this.getSharedLibraryNameFromJBIDescriptor(jbiDescriptor);
URL jbiPackageURL = new File(outputDirectory + File.separator
+ jbiName + ".zip").toURI().toURL();
installSharedLibraryCore(petalsJMXClient, sharedLibraryName, jbiPackageURL,
force.booleanValue(), "");
} catch (MalformedURLException e) {
throw new MojoExecutionException(
"Invalid URL for JBI archive location", e);
}
}
private void installSharedLibraryCore(PetalsJMXClient petalsJMXClient,
String sharedLibraryName, URL jbiPackageURL,
boolean forceInstallation, final String prefixLog) throws MojoExecutionException {
try {
PetalsInstallationServiceClient pisc = petalsJMXClient
.getInstallationServiceClient();
String[] installedSharedLibraries = pisc
.retrieveInstalledSharedLibraries();
boolean sharedLibraryFound = false;
for (int i = 0; i < installedSharedLibraries.length; i++) {
if (sharedLibraryName.equals(installedSharedLibraries[i])) {
this.info(prefixLog + "The shared-library is already installed.");
sharedLibraryFound = true;
break;
}
}
if (!forceInstallation && sharedLibraryFound) {
throw new MojoExecutionException(
"The shared-library is already installed");
} else if (!sharedLibraryFound) {
this.info(prefixLog + "Shared-library uninstalled.");
}
// We uninstall the shared-library if it was previously installed
if (forceInstallation && sharedLibraryFound) {
this.info(prefixLog + "The force mode is asked.");
// we check if components are depending on the shared-library.
// If yes, shutdown these components.
this.checkAndShutdownComponents(petalsJMXClient, pisc,
sharedLibraryName, prefixLog + "\t");
this.info(prefixLog + "Uninstalling the shared-library ("
+ sharedLibraryName + ").");
if (pisc.uninstallSharedLibrary(sharedLibraryName)) {
this.info(prefixLog + "Shared-library uninstalled.");
} else {
throw new MojoExecutionException(
"Problem during uninstallation. Check server logs.");
}
}
// At this point, the SL is not installed. We have a clean
// environnment
// We can install the shared libaray
jbiPackageURL = uploadJBIArchiveIfNeeded(jbiPackageURL, prefixLog + "\t");
this.info(prefixLog + "Installing the shared-library ("
+ jbiPackageURL.toExternalForm() + ").");
String slName = pisc.installSharedLibrary(jbiPackageURL);
this.info(prefixLog + "The shared-library (" + slName + ") is installed.");
} catch (ConnectionErrorException e) {
throw new MojoExecutionException("Error", e);
} catch (PerformActionErrorException e) {
throw new MojoExecutionException("Error", e);
} catch (InstallationServiceErrorException e) {
throw new MojoExecutionException("Error", e);
} catch (InstallationServiceDoesNotExistException e) {
throw new MojoExecutionException(
"Unable to find the installation service ", e);
}
}
private void checkAndShutdownComponents(PetalsJMXClient petalsJMXClient,
final PetalsInstallationServiceClient pisc,
final String sharedLibraryName, final String prefixLog)
throws MojoExecutionException {
try {
String[] components = pisc
.retrieveComponentsForInstalledSharedlibrary(sharedLibraryName);
for (int i = 0; i < components.length; i++) {
this.info(prefixLog + "Checking " + components[i] + " ...");
try {
PetalsComponentComponentClient pccc = petalsJMXClient
.getComponentComponentClient(components[i]);
this.info(prefixLog + components[i] + " installed.");
String componentState = pccc.getState();
if (PetalsComponentComponentClient.State.STARTED
.equals(componentState)
|| PetalsComponentComponentClient.State.STOPPED
.equals(componentState)) {
this.info(prefixLog + components[i] + " is in state: "
+ componentState);
this.info(prefixLog + "Shutdowning it ...");
pccc.shutdown();
this.info(prefixLog + components[i] + " shutdowned.");
}
} catch (ComponentComponentDoesNotExistException e) {
this.info(prefixLog + components[i] + " not installed.");
}
this.info(prefixLog + components[i] + " checked.");
}
} catch (AttributeErrorException e) {
throw new MojoExecutionException("Error", e);
} catch (PerformActionErrorException e) {
throw new MojoExecutionException("Error", e);
} catch (ComponentErrorException e) {
throw new MojoExecutionException("Error", e);
} catch (ConnectionErrorException e) {
throw new MojoExecutionException("Error", e);
}
}
/**
*
* This method checks that all components needed by a service assembly are
* installed and started.
*
*
* If a component is missing and the parameter
* startMissingComponents
is set to true
, the
* component will be installed and started from the declared Maven
* repositories. The parameter force
is used to drive the
* component installation processing.
*
*
* Note: A component needed by several service units of the service assembly
* is installed only once.
*
*
* @param petalsJMXClient
* The JMX connection to use for JMX calls.
* @throws MojoExecutionException
*/
private void checkAndStartComponents(PetalsJMXClient petalsJMXClient,
final JBIDescriptor jbiDescriptor, final String prefixLog)
throws MojoExecutionException {
try {
List usedComponents = this
.getComponentsFromServiceAssemblyJBIDescriptor(jbiDescriptor);
List processedComponents = new ArrayList();
for (String usedComponent : usedComponents) {
if (!processedComponents.contains(usedComponent)) {
PetalsComponentComponentClient pccc = null;
try {
this.info(prefixLog + "Checking " + usedComponent + " ...");
pccc = petalsJMXClient
.getComponentComponentClient(usedComponent);
this.info(prefixLog + usedComponent + " installed.");
} catch (ComponentComponentDoesNotExistException e) {
// The component is not installed
this.info(prefixLog + usedComponent + " not installed.");
if (startMissingComponents.booleanValue()) {
// We install it from Maven repositories
pccc = this.installComponentFromMaven(petalsJMXClient,
usedComponent, prefixLog + "\t");
if (pccc == null) {
throw new MojoExecutionException(
"Unable to install the dependent component.");
}
}
else {
throw new MojoExecutionException("A component ("
+ usedComponent
+ ") is missing on the PEtALS server.");
}
}
if (pccc != null) {
// The component is installed
if ((PetalsComponentComponentClient.State.STOPPED
.equals(pccc.getState()))
|| (PetalsComponentComponentClient.State.SHUTDOWN
.equals(pccc.getState()))
&& this.startMissingComponents.booleanValue()) {
this.info(prefixLog + "Starting " + usedComponent
+ "...");
pccc.start();
this.info(prefixLog + usedComponent + " started.");
} else if (PetalsComponentComponentClient.State.STARTED
.equals(pccc.getState())) {
this.info("The component (" + usedComponent
+ ") is started on the PEtALS server.");
} else {
throw new MojoExecutionException("The component ("
+ usedComponent + ") is in the state \""
+ pccc.getState() + "\" on the PEtALS server.");
}
processedComponents.add(usedComponent);
}
}
else {
this.info("Component previously installed.");
}
}
} catch (ComponentErrorException e) {
throw new MojoExecutionException("Error:", e);
} catch (ConnectionErrorException e) {
throw new MojoExecutionException("Error:", e);
} catch (PerformActionErrorException e) {
throw new MojoExecutionException("Error on starting component:", e);
} catch (AttributeErrorException e) {
throw new MojoExecutionException(
"Error getting the component status:", e);
}
}
/**
*
* This method checks that all shared-libraries needed by a component are
* installed.
*
*
* If a shared-library is missing and the parameter
* startMissingComponents
is set to true
, the
* shared-library will be installed from the declared Maven repositories.
* The parameter force
is not used to drive the
* shared-library installation processing.
*
*
* @param petalsJMXClient
* The JMX connection to use for JMX calls.
* @apram prefixLog The prefix of each logged messages.
* @throws MojoExecutionException
*/
private void checkAndInstallSharedLibraries(
PetalsJMXClient petalsJMXClient, final JBIDescriptor jbiDescriptor, final String prefixLog)
throws MojoExecutionException {
try {
PetalsInstallationServiceClient pisc = petalsJMXClient
.getInstallationServiceClient();
this.info(prefixLog
+ "Getting installed and needed shared-libraries ...");
String[] installedSharedLibraries = pisc
.retrieveInstalledSharedLibraries();
List neededSharedLibraries = this
.getSharedLibrariesFromComponentJBIDescriptor(jbiDescriptor);
this.info(prefixLog
+ "Installed and needed shared-libraries retrieved.");
for (String neededSharedLibrary : neededSharedLibraries) {
boolean neededSharedLibraryInstalled = false;
this.info(prefixLog + "Checking the needed shared-library "
+ neededSharedLibrary + " ...");
for (int i = 0; i < installedSharedLibraries.length; i++) {
if (neededSharedLibrary.equals(installedSharedLibraries[i])) {
neededSharedLibraryInstalled = true;
break;
}
}
if (neededSharedLibraryInstalled) {
this.info(prefixLog + "The shared-library "
+ neededSharedLibrary + " is installed.");
} else {
this.info(prefixLog + "The shared-library "
+ neededSharedLibrary + " is not installed.");
if (installMissingSharedLibraries.booleanValue()) {
// We install it from Maven repositories
if (PACKAGING_COMPONENT.equals(this.project.getArtifact().getType())) {
installSharedLibraryFromMaven(petalsJMXClient,
neededSharedLibrary, this.project, prefixLog + "\t");
}
else {
// We must retrieve the Maven project associated to
// the component to be able to install the SL
installSharedLibraryFromMaven(petalsJMXClient,
neededSharedLibrary, getComponentProjectFromSAProject(this.project, jbiDescriptor.getComponent().getIdentification().getName(), prefixLog), prefixLog + "\t");
}
}
else {
throw new MojoExecutionException("A shared-libarry (" + neededSharedLibrary + ") is missing on the PEtALS server.");
}
}
}
} catch (InstallationServiceDoesNotExistException e) {
throw new MojoExecutionException(
"Unable to find the installation service ", e);
} catch (InstallationServiceErrorException e) {
throw new MojoExecutionException("Error", e);
} catch (ConnectionErrorException e) {
throw new MojoExecutionException("Error:", e);
} catch (PerformActionErrorException e) {
throw new MojoExecutionException("Error on starting component:", e);
}
}
private MavenProject getComponentProjectFromSAProject(
final MavenProject saProject, final String componentName,
final String prefixLog) throws MojoExecutionException {
try {
MavenProject componentProject = null;
List serviceAssemblyDependencies = saProject
.getDependencies();
for (Dependency serviceAssemblyDependency : serviceAssemblyDependencies) {
this.debug(prefixLog + "service-assembly dependency: "
+ serviceAssemblyDependency.getGroupId() + ":"
+ serviceAssemblyDependency.getArtifactId());
if (PACKAGING_SU.equals(serviceAssemblyDependency.getType())) {
Artifact serviceUnitArtifact = this.artifactFactory
.createDependencyArtifact(
serviceAssemblyDependency.getGroupId(),
serviceAssemblyDependency.getArtifactId(),
VersionRange
.createFromVersionSpec(serviceAssemblyDependency
.getVersion()),
serviceAssemblyDependency.getType(),
serviceAssemblyDependency.getClassifier(),
serviceAssemblyDependency.getScope(), null,
serviceAssemblyDependency.isOptional());
MavenProject serviceUnitProject = mavenProjectBuilder
.buildFromRepository(serviceUnitArtifact,
this.project
.getRemoteArtifactRepositories(),
this.localRepository);
List serviceUnitDependencies = serviceUnitProject
.getDependencies();
for (Dependency serviceUnitDependency : serviceUnitDependencies) {
this.debug(prefixLog + "service-unit dependency: "
+ serviceUnitDependency.getGroupId() + ":"
+ serviceUnitDependency.getArtifactId());
if (PACKAGING_COMPONENT.equals(serviceUnitDependency
.getType())) {
Artifact componentArtifact = this.artifactFactory
.createDependencyArtifact(
serviceUnitDependency.getGroupId(),
serviceUnitDependency
.getArtifactId(),
VersionRange
.createFromVersionSpec(serviceUnitDependency
.getVersion()),
serviceUnitDependency.getType(),
serviceUnitDependency
.getClassifier(),
serviceUnitDependency.getScope(),
null, serviceUnitDependency
.isOptional());
this.artifactResolver.resolve(componentArtifact,
this.project
.getRemoteArtifactRepositories(),
this.localRepository);
// We check if the artifact is the expected
// component using its jbi.xml
JBIDescriptor jbiComponentDescriptor = this
.readJbiDescriptor(componentArtifact
.getFile());
ComponentDescription componentDescription = jbiComponentDescriptor
.getComponent();
if (componentName.equals(componentDescription
.getIdentification().getName())) {
// It's the expected component.
componentProject = mavenProjectBuilder
.buildFromRepository(
componentArtifact,
this.project
.getRemoteArtifactRepositories(),
this.localRepository);
break;
}
}
}
if (componentProject != null) {
// The component maven project has been found, we stop
// to find it
break;
}
}
}
if (componentProject == null) {
throw new MojoExecutionException(
"Component maven project not found.");
}
return componentProject;
} catch (ArtifactResolutionException e) {
throw new MojoExecutionException(
"Error during the artifact resolution.", e);
} catch (ArtifactNotFoundException e) {
throw new MojoExecutionException(
"Error during the artifact resolution.", e);
} catch (InvalidVersionSpecificationException e) {
throw new MojoExecutionException(
"Error during the artifact resolution.", e);
} catch (ProjectBuildingException e) {
throw new MojoExecutionException(
"Error during the artifact resolution.", e);
}
}
/**
*
* Install a component from a JBI archive located in a Maven repository.
*
* @param petalsJMXClient
* The JMX connection to use for JMX calls.
* @param componentName
* The component identifier.
* @return The associated PetalsComponentComponentClient
if
* the installation is successfulled, null
otherwise.
* @throws MojoExecutionException
*/
private PetalsComponentComponentClient installComponentFromMaven(
PetalsJMXClient petalsJMXClient, final String componentName,
final String prefixLog) throws MojoExecutionException {
try {
PetalsComponentComponentClient pccc = null;
this.info(prefixLog + "Installing " + componentName
+ " from a Maven repository...");
/* We retrieve the artifact associated to the component */
boolean serviceUnitFoundInDependencies = false;
boolean componentFoundInDependencies = false;
List serviceAssemblyDependencies = project.getDependencies();
for (Dependency serviceAssemblyDependency : serviceAssemblyDependencies) {
this.debug(prefixLog + "service-assembly dependency: " + serviceAssemblyDependency.getGroupId() + ":" + serviceAssemblyDependency.getArtifactId());
if (PACKAGING_SU.equals(serviceAssemblyDependency.getType())) {
serviceUnitFoundInDependencies = true;
Artifact serviceUnitArtifact = this.artifactFactory
.createDependencyArtifact(
serviceAssemblyDependency.getGroupId(),
serviceAssemblyDependency.getArtifactId(),
VersionRange
.createFromVersionSpec(serviceAssemblyDependency
.getVersion()),
serviceAssemblyDependency.getType(),
serviceAssemblyDependency.getClassifier(),
serviceAssemblyDependency.getScope(), null,
serviceAssemblyDependency.isOptional());
MavenProject serviceUnitProject = mavenProjectBuilder
.buildFromRepository(serviceUnitArtifact, project
.getRemoteArtifactRepositories(),
this.localRepository);
List serviceUnitDependencies = serviceUnitProject
.getDependencies();
for (Dependency serviceUnitDependency : serviceUnitDependencies) {
this.debug(prefixLog + "service-unit dependency: " + serviceUnitDependency.getGroupId() + ":" + serviceUnitDependency.getArtifactId());
if (PACKAGING_COMPONENT.equals(serviceUnitDependency.getType())) {
Artifact componentArtifact = this.artifactFactory
.createDependencyArtifact(
serviceUnitDependency.getGroupId(),
serviceUnitDependency
.getArtifactId(),
VersionRange
.createFromVersionSpec(serviceUnitDependency
.getVersion()),
serviceUnitDependency.getType(),
serviceUnitDependency
.getClassifier(),
serviceUnitDependency.getScope(),
null, serviceUnitDependency
.isOptional());
this.artifactResolver.resolve(componentArtifact,
this.project
.getRemoteArtifactRepositories(),
this.localRepository);
// We check if the artifact is the expected
// component using its jbi.xml
JBIDescriptor jbiComponentDescriptor = this
.readJbiDescriptor(componentArtifact
.getFile());
ComponentDescription componentDescription = jbiComponentDescriptor
.getComponent();
if (componentName.equals(componentDescription
.getIdentification().getName())) {
// It's the expected component. We install the component
componentFoundInDependencies = true;
this.installComponentCore(petalsJMXClient,
jbiComponentDescriptor,
componentArtifact.getFile()
.toURI().toURL(), prefixLog
+ "\t");
this.info(prefixLog + componentName
+ " installed.");
pccc = petalsJMXClient
.getComponentComponentClient(componentName);
}
else {
this.info(prefixLog + componentDescription
.getIdentification().getName() + " found but not needed -> not installed.");
}
}
}
}
}
if (!componentFoundInDependencies) {
throw new MojoExecutionException("The JBI component is declared in no service unit POM.");
}
if (!serviceUnitFoundInDependencies) {
throw new MojoExecutionException("No service-units declared in this service-assembly.");
}
this.info(prefixLog + componentName
+ " installed from a Maven repository...");
return pccc;
} catch (ConnectionErrorException e) {
throw new MojoExecutionException(
"Unbale to retrieve the JMX component associated to the JBI component",
e);
} catch (ComponentErrorException e) {
throw new MojoExecutionException(
"Unbale to retrieve the JMX component associated to the JBI component",
e);
} catch (ComponentComponentDoesNotExistException e) {
throw new MojoExecutionException(
"Unbale to retrieve the JMX component associated to the JBI component",
e);
} catch (MalformedURLException e) {
throw new MojoExecutionException(
"Invalid URL for JBI archive location", e);
} catch (InvalidVersionSpecificationException e) {
throw new MojoExecutionException(
e.getLocalizedMessage(),
e);
} catch (ProjectBuildingException e) {
throw new MojoExecutionException(
e.getLocalizedMessage(),
e);
} catch (ArtifactResolutionException e) {
throw new MojoExecutionException("Error during the artifact resolution.",
e);
} catch (ArtifactNotFoundException e) {
throw new MojoExecutionException("Artifact not found.", e);
}
}
/**
*
* Install a shared-library from a JBI archive located in a Maven
* repository.
*
* @param petalsJMXClient
* The JMX connection to use for JMX calls.
* @param sharedLibraryName
* The shared-library identifier.
* @throws MojoExecutionException
*/
private void installSharedLibraryFromMaven(PetalsJMXClient petalsJMXClient,
String sharedLibraryName, final MavenProject mvnProject,
final String prefixLog) throws MojoExecutionException {
try {
this.info(prefixLog + "Installing " + sharedLibraryName
+ " from a Maven repository...");
boolean sharedLibraryInstalled = false;
boolean foundInDependencies = false;
List dependencies = mvnProject.getDependencies();
for (Dependency dependency : dependencies) {
if (PACKAGING_SL.equals(dependency.getType())) {
foundInDependencies = true;
try {
Artifact artifact = this.artifactFactory
.createDependencyArtifact(dependency
.getGroupId(), dependency
.getArtifactId(), VersionRange
.createFromVersionSpec(dependency
.getVersion()), dependency
.getType(), dependency.getClassifier(),
dependency.getScope(), null, dependency
.isOptional());
this.artifactResolver.resolve(artifact, this.project
.getRemoteArtifactRepositories(),
this.localRepository);
// We check if the artifact is the expected component
// using its jbi.xml
JBIDescriptor jbiComponentDescriptor = this
.readJbiDescriptor(artifact.getFile());
SharedLibrary sharedLibrary = jbiComponentDescriptor
.getSharedLibrary();
if (sharedLibraryName.equals(sharedLibrary
.getIdentification().getName())) {
// We install the component
this.installSharedLibraryCore(petalsJMXClient,
sharedLibraryName, artifact.getFile().toURI()
.toURL(), false, prefixLog /*+ "\t"*/);
sharedLibraryInstalled = true;
}
} catch (InvalidVersionSpecificationException e) {
throw new MojoExecutionException(e
.getLocalizedMessage(), e);
} catch (ArtifactResolutionException e) {
this.error(prefixLog + "Error during the artifact resolution.",
e);
} catch (ArtifactNotFoundException e) {
this.error(prefixLog + "Artifact not found.");
}
}
}
if (!foundInDependencies) {
this.info(prefixLog + sharedLibraryName + " not found in dependencies.");
}
if (sharedLibraryInstalled) {
this.info(prefixLog + sharedLibraryName + " installed.");
} else {
this.info(prefixLog + sharedLibraryName + " not installed.");
}
} catch (MalformedURLException e) {
throw new MojoExecutionException(
"Invalid URL for JBI archive location", e);
}
}
private void uploadArchive(File jbiArchive, final String prefixLog) throws MojoExecutionException {
Wagon wagon = null;
try {
String url = this.protocol + "://" + this.host + this.remoteDirectory;
this.debug(prefixLog + "uploading URL: " + url);
Repository repository = new Repository(this.serverId, url);
wagon = this.wagonManager.getWagon(repository);
try {
Debug debug = new Debug();
wagon.addSessionListener(debug);
wagon.addTransferListener(debug);
wagon.connect(repository, this.wagonManager
.getAuthenticationInfo(this.serverId));
wagon.put(jbiArchive, jbiArchive.getName());
} catch (ConnectionException e) {
throw new MojoExecutionException(
"Error connecting to the Petals server", e);
} catch (AuthenticationException e) {
throw new MojoExecutionException(
"Error authenticating on the Petals server", e);
} catch (TransferFailedException e) {
throw new MojoExecutionException(
"Error transfering the JBI archive", e);
} catch (AuthorizationException e) {
throw new MojoExecutionException(
"Not authorized to upload in the remote directory.", e);
} catch (ResourceDoesNotExistException e) {
throw new MojoExecutionException("Error uploading", e);
} finally {
try {
wagon.disconnect();
} catch (ConnectionException e) {
getLog().error("Error disconnecting wagon - ignored", e);
}
}
} catch (UnsupportedProtocolException e) {
throw new MojoExecutionException("Unsupported protocol: '"
+ this.protocol + "'", e);
} catch (WagonConfigurationException e) {
throw new MojoExecutionException("Misconfigured protocol: '"
+ this.protocol + "'", e);
}
}
private URL uploadJBIArchiveIfNeeded(final URL jbiArchiveURL, final String prefixLog)
throws MojoExecutionException {
// If the Petals server is not the local machine, we should upload
// the component. The upload will be relaized by wagon
URL newJBIArchiveURL = null;
try {
InetAddress localIp = InetAddress.getLocalHost();
InetAddress petalsServerIp = InetAddress.getByName(this.host);
if (!localIp.equals(petalsServerIp)) {
this.info(prefixLog
+ "The JBI archive will be uploaded on the Petals server.");
this.debug(prefixLog + "source JBI archive URL: " + jbiArchiveURL);
File fileToUpload = new File(jbiArchiveURL.toURI());
uploadArchive(fileToUpload, prefixLog);
newJBIArchiveURL = new URL("file", null, this.remoteDirectory
+ "/" + new File(jbiArchiveURL.toURI()).getName());
this.debug(prefixLog + "remote JBI archive URL: " + newJBIArchiveURL);
}
else {
newJBIArchiveURL = jbiArchiveURL;
}
return newJBIArchiveURL;
} catch (UnknownHostException e) {
throw new MojoExecutionException("Networking error", e);
} catch (URISyntaxException e) {
throw new MojoExecutionException(
"URI error", e);
} catch (MalformedURLException e) {
throw new MojoExecutionException(
"Invalid URL for JBI archive location", e);
}
}
private void checkAndUndeployServiceAssemblies(PetalsJMXClient petalsJMXClient,
final String componentName, final String prefixLog)
throws MojoExecutionException {
try {
PetalsDeploymentServiceClient pdsc = petalsJMXClient
.getDeploymentServiceClient();
String[] serviceAssemblies = pdsc
.getDeployedServiceAssembliesForComponent(componentName);
for (int i = 0; i < serviceAssemblies.length; i++) {
this.info(prefixLog + "Undeploying the service-assembly: "
+ serviceAssemblies[i] + " ...");
pdsc.undeploy(serviceAssemblies[i]);
this.info(prefixLog + "service-assembly undeployed: " + prefixLog);
}
} catch (DeploymentServiceErrorException e) {
throw new MojoExecutionException("Error", e);
} catch (DeploymentServiceDoesNotExistException e) {
throw new MojoExecutionException(
"Unable to find the deployment service ", e);
} catch (ConnectionErrorException e) {
throw new MojoExecutionException("Error", e);
} catch (PerformActionErrorException e) {
throw new MojoExecutionException("Error", e);
}
}
}